Computer Memory and Assembly Language
Computer memory is an important topic when it comes to learning assembly.
There are two types of memory: cache memory (L1, L2, L3), and random access memory, or RAM. Along with the registers, these two types of memory provide a place for the processor to store and retrieve data.
Because we work so closely with memory in assembly, it can be extremely helpful to learn the core concepts of how computer memory works.
What is Computer Memory?
Computer memory refers to the devices or components used to store data or instructions temporarily.
The term ‘memory’ typically refers to volatile storage; meaning that the data is gone once power is lost. This is contrast to storage, such as an SSD or HDD, which is much slower but can store data permanently.
Memory is a crucial component of any computing system, enabling the computer to store and retrieve data quickly for processing. There are two types of memory:
- Cache memory: L1, L2, and L3 caches.
- RAM: Random Access Memory.
Memory is extremely fast, but both types of memory are slower than the registers.
Cache Memory
Cache memory lives on the CPU itself and is therefore extremely fast.
It is essentially used as a buffer between the CPU and RAM, storing commonly used data and instructions to dramatically improve efficiency.
The cache memory is much smaller than RAM, but also much faster.
There are commonly three ‘levels’ of cache memory: L1, L2, and L3.
- L1 is the smallest and fastest cache. It is located within a single CPU core. Only the registers are faster than the L1 cache. Its size is typically in kilobytes.
- L2 is larger than the L1 cache, but also (slightly) slower. It is shared between CPU cores. The registers and L1 cache are faster than L2, but L2 is also larger, usually in megabytes.
- L3 is the largest and slowest of the cache memory levels. Note that it is still much faster than RAM. Not all CPUs use L3.
Cache memory is larger but slower than the registers, and RAM is larger but slower than cache memory.
RAM Memory
RAM is located on a separate chip from the CPU and is therefore slower than the registers or cache memory.
However, it can also be much bigger. Depending on the device, RAM can also be added or updated. It is common to see 16 GB of RAM on home laptop/desktop computers. Some workstations and servers have 64GB or more.
While we covered cache memory first, RAM is considered the primary form of temporary, or volatile, storage on a computer. In fact, when we start a program, the entire program gets at least partially loaded into RAM in order to speed up execution.
Registers and cache memory are both used to optimize the processor’s functionality, but RAM is where most data gets stored and is, by itself, commonly referred to as ‘memory’.
Register vs. Cache Memory vs. RAM Speed
In order to understand the speed difference between the registers, cache memory, and RAM, let’s look at how long each takes to retrieve a single assembly instruction.
Registers: Retrieving a single assembly instruction from the registers takes only one cycle.
Cache Memory: Retrieving it from cache memory typically takes a few cycles.
RAM: Retrieving the instruction from RAM takes about 200 cycles.
Type | Size | Speed |
Registers | 64 bytes | Fastest |
L1 Cache | kilobytes | Slower than the registers. Faster than anything else. |
L2 Cache | megabytes | Slower than the L1 cache. |
L3 Cache | megabytes | Slower than the L2 cache. |
RAM | gigabytes | Slower than L3 cache. |
Storage | giga/terabytes | Slower than RAM. |
Memory and Addressing for x86-64 Assembly
A memory address is a unique identifier assigned to each byte (or sometimes word) of memory in a computer system.
Memory addresses are used to locate and access specific data or instructions stored in memory. A memory address is typically represented in hexadecimal, and has the size indicated by the architecture. A 32-bit system has 32-bit memory addresses, while a 64-bit system has 64-bit addresses.
System architecture determines the size of memory addresses, the maximum amount of memory as well as the available memory addresses.
- 32-bit (x86) systems have a maximum possible RAM amount of 232 bytes, and the highest possible address is 0xffffffff.
- 64-bit (x64) systems have a maximum possible RAM amount of 264 bytes. The highest possible address is 0xffffffffffffffff.
Memory and Assembly Programs
When we run a program, its data and instructions are copied into memory. This allows the CPU to execute the code much faster than if all of this data needed to be retrieved from storage on a disk drive (e.g. SSD).
In memory, the program data is stored in a specific format, as shown in the image below:
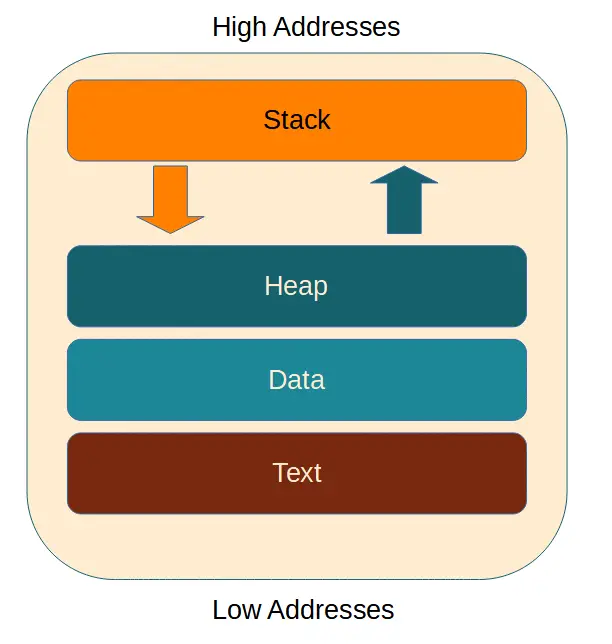
There are four regions of memory allocated for the program, each with a specific purpose.
Stack: The stack functions as a LIFO data structure. It is very fast to use. Data can be pushed onto, or popped off the stack. The stack is an essential part of the functionality of assembly programming, storing data needed for program execution.
Heap: The heap is a slower but larger area of memory in the stack. It is more versatile since data can be stored or retrieved from any location on the heap.
Data: Used to hold variables. There are two sections: Data holds assigned variables, while .bss holds unassigned variables.
Text: This is where the assembly instructions (i.e. the assembly code) are stored.
When we close or terminate a program, its data is removed in order to maximize the free memory available for other programs.