Go Programming
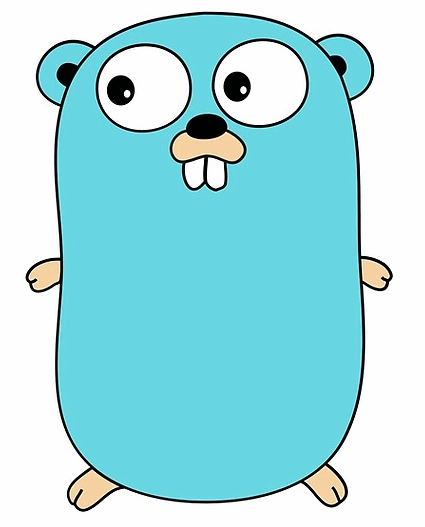
Welcome to our main course on the Go programming language (Golang)!
This page contains links to Go tutorials, as well as an in-depth one page tutorial to help you get up and running quickly with Golang.
GoLang Tutorials
Comments
Variables
Constants
Data Types
– Booleans
– Strings
– Integers
– Floats
– Complex Numbers
Arrays
Go is a programming language that is popular due to its’ ease of use, speed, and safety features.
Go is one of the fastest compiled languages in the world, and it is almost as easy to learn and use as Python.
One of the features unique to Go is its’ garbage collector, which is used to periodically clear memory space that is no longer needed.
Go isn’t quite as safe as Rust, but it is much easier to learn.
What is Go?
Created at Google, Go is a statically typed, compiled programming language.
What makes Go so appealing is its’ unique combination of features:
- Go is fast: Its speed is comparable to other high performance languages like C, C++, and Rust.
- Go is easy to learn and use: It’s great for a first language or a startup.
- Go is safe: It offers safety features similar to Rust (although Rust is definitely safer).
You can think of Go as a language that manages to capture most of the speed and safety of Rust while being almost as easy to learn as Python.
In order to understand Go, it’s helpful to review some of its’ basic features like the garbage collector.
The Golang Garbage Collector
The garbage collector is a bit unique, and its one of the things that make the Go language stand out. It results in much greater speed and safety, and it works in the background so that the programmer doesn’t have to worry about it.
The garbage collector is a background process that runs periodically. It scans the computer memory and clears any unnecessary space. This is great because normally after memory is allocated, it can only be cleared explicitly.
Most programming languages don’t worry about this, which causes them to take up more memory than is strictly necessary. In C/C++, programmers have to work hard to explicitly clean up, essentially doing the ‘garbage collection’ themselves.
The garbage collector in Go does this for the programmer, and this results in far less memory consumption.
Comments
As with many other languages, Go supports both single and multi-line comments.
Single lines can be commented out using two forward slashes // and multiple lines can be commented using a slash asterisk: /* */.
func main() {
// This is a single line comment
/* This is a
multi-line comment
*/
}
Comments are useful not only when annotating code but also for commenting out code while programming and debugging. In other words, comments are used as a general rule to tell the compiler what to execute (and what not to execute).
Main article: Comments
Variables
Variables in Go can be declared using either the var keyword or a colon before the assignment operator :=.
// Declare variable using var keyword
var var1 = 42
// Declare variable using :=
var2 := "Hello!"
//Declare variable without value assignment
var var3 string
// Value can be assigned after declaration
var3 = "Goodbye!"
There are some nuances and limitations with these different methods; check out the main tutorial on to learn all the juicy details.
Main article: Variables
Constants
We can use constants in cases when we know that the value of a variable will not need to be changed. Constants are declared using the keyword const, and the most common convention is to name them using UPPERCASE letters:
const MYNAME = "Bob"
const MYNUM = 42
const MYBOOL = true
Constants can be numeric types, Booleans, or strings. Once a value is assigned, a constant can never be changed. This makes them safer but also more restrictive than variables.
Main article: Constants
Data Types
There are four basic data type classifications in Golang: Boolean, string, numeric, and derived types.
Main article: Data Types
Boolean Data Types
Boolean types can have hold a value of either true or false. They can be declared in a variety of ways:
var b1 bool = true // Explicit declaration of Boolean variable
var b2 = true // Implicit declaration; compiler infers type
b3 := true // Simple way of declaring Boolean type var
var b4 bool // Will default to 'false'
const B1 = false // Boolean typed constant
Main article: Boolean Data Type
Strings
In Go, a string is a slice of bytes. You can think of a string as a data type comprised of a series of characters.
A string variable can easily be created by placing a series of characters inside double quotes:
greet := "Hello, World!"
In this example, we created a new string variable called ‘greet‘. The value of ‘greet’ is “Hello, World!”
There are many useful things that we can do with strings. We can print a string, gets its’ length, access its’ characters, and even join two strings together.
Some of these are shown in the code below:
var greet = "Hello, World!" // Create a string variable
var length = len(greet) // Get the length of a string
var mychar = hello[0] // Get a character from the string
fmt.Println(greet) // Print a whole string
fmt.Printf("String: %c", mychar) // Print a single character
Main article: Strings
Integers
Integers are whole numbers without a decimal point. Examples are numbers like 1, 2, -42, and 65535.
Integer types can be divided into signed and unsigned integer types. Unsigned integers can only be positive, while signed integers can be either positive or negative.
There are 5 types of signed integers and 6 types of unsigned integers. If we don’t specify the integer type, then the Go compiler will default to the ‘int‘ type. The size of an int type depends on the system architecture; 32-bit systems will default to 32-bit integers, while 64-bit systems default to 64-bit integers.
The following code example shows how to create and print integers of different types, including the default int type.
package main
import (
"fmt"
)
func main() {
var num1 = 42 // Default type is 'int'
var num2 uint32 = 1 // Declaring a uint32
var num3 int64 = -8 // Using an int64 to store a negative
fmt.Println(num1)
fmt.Println(num2)
fmt.Println(num3)
}
Output:
42 1 -8
Main article: Integers