Java Programming
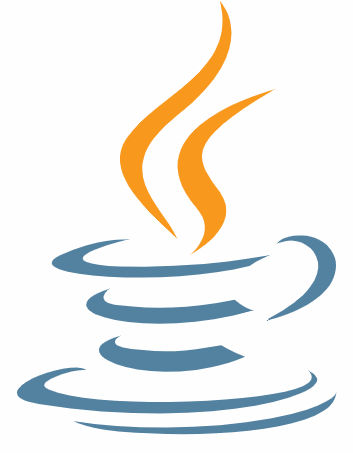
Java is a high-level, object-oriented programming language that originally developed by Sun Microsystems and released in 1995. It was designed to be platform-independent, meaning that Java programs can run on any devices or operating systems that have a Java Virtual Machine (JVM) installed.
Java is known for its “write once, run anywhere” principle. This allows developers to write code once and run it on multiple platforms without the need for recompilation. It is achieved through the use of the JVM, which translates Java bytecode into machine-specific instructions at runtime.
The Java language is strongly typed and supports features such as automatic memory management via garbage collection, exception handling, and multithreading. It provides a rich set of libraries and APIs (Application Programming Interfaces) which make it easier for developers to build various types of applications, ranging from desktop software to web and mobile applications.
Java is used in many industries and it has a large and active developer community. It is commonly used for enterprise applications, Android app development, web development (using frameworks like JavaServer Faces, Spring, or JavaServer Pages), scientific computing, and more. Java’s popularity and versatility have contributed to its continued relevance in the software development landscape.
Java Tutorials
The Java Compiling Process
Java Comments
Java Printing
Whitespace in Java
Java Variables
— Printing Variables
— Declaring Multiple Variables
— Variable identifiers
Java Data Types
— Numeric Types (Numbers)
— Booleans
— Characters
Java Strings
Java Operators
— Arithmetic Operators
— Assignment Operators
Java Articles
Add a char to a string
Compare if Strings are Equal (or Not Equal)
Comparing Two Characters
Convert an Integer to a Char
Convert a String to BigDecimal
Default value of boolean primitives and Boolean objects
Exiting a Program
Exponents
Get the Length of a Two-Dimensional (2D) Array
Global Variables in Java
Instance Variables
Java vs. Golang
Java vs. The .NET Framework
Print a HashMap
Print an ArrayList
Remove Quotes from a String
Rounding Numbers
Java Tutorial
A Quick Look at Java Code
One of the best ways to get start learning any programming language is to have a quick look at the structure of a program.
We’ll use the following Hello, World! program to demonstrate some of the most fundamental aspects of the Java language:
// Every Java program must have at least one class.
public class HelloWorld {
// Every program must have a main() function.
public static void main(String[] args) {
// The following statement prints the string "Hello, World!"
System.out.print("Hello, World!");
}
}
There’s a lot we can learn from this short program, and the comments in the code help to explain what’s going on.
public class HelloWorld {}
The first line is used to create a class called ‘HelloWorld’. All Java programs must have at least one class. We will learn a lot about classes going forward, but for now we just need to know that Java is an object-oriented programming language and a class is an object template.
public static void main(String[] args) {}
The second line ‘public static void main(String[] args)’ creates the main() function. All Java programs require a main() function, and it tells the system where to begin code execution. The beginning of main() is the official start of the program.
System.out.print("Hello, World!");
Finally, this line prints the string “Hello, World!” to the console output.
Of course, there’s more to this example than what we’ve covered. Java can be intimidating because it looks (and is) more verbose than some other languages like Python or Rust, but it’s also relatively easy to learn once we understand the basic principles.
Java Comments
Java supports both single-line and multi-line comments. Single-line comments start with a //, while multi-line comments start with a /* and end with */.
// Single line comment
/* Multi
line
comment
*/
Main Article: Java Comments
Printing To The Console
In Java, we can print to the console using three types of print statements:
- System.out.print()
- System.out.println()
- System.out.printf()
Of these, System.out.println() is the most common because it prints and then adds a new line character so that the next print statement will print on a new line!
The print() method can be used to print without moving the cursor to a new line:
System.out.print("Hello, ");
System.out.print("World!");
Output:
Hello, World!
The println() method is almost identical, but it appends a new line as well:
System.out.println("Hello, ");
System.out.println("World!");
Output:
Hello,
World!
Variables and Data Types
Variables are used to store information, and allow that information to be associated with a name.
You can declare a variable without initializing it. In this example, we are declaring an integer variable called ‘myNum’:
int myNum;
In this case, we haven’t yet initialized it to a value, but we could have done this in the declaration:
int myNum = 42;
In Java, a variable can be one of several data types, including Boolean (boolean), character (char), integer (int) or floating-point number (float), or string (String).
bool myBool = false;
char myChar = 'A';
int myNum = 42;
String myGreet = "Hello, World!";
Main Article: Java Variables
Printing Variables
We can print variables using the print() and println() methods.
For example:
int myNum = 42;
System.out.println(myNum);
Output:
42
We can combine variables and text inside the print statement by designating text using double quotes “” and using the ‘+‘ concatenation operator:
int myNum = 42;
System.out.println("My number is " + myNum);
Output:
My number is 42
We can even do math inside a println() statement!
int x = 5;
int y = 10;
System.out.println(x + y);
Output:
15
Main Article: Printing Java Variables
Declaring Multiple Variables
Java allows us to declare and/or initialize multiple variables of the same type on the same line.
For example, if we want to declare and initialize two integer values, we can use a comma-separated list:
public class Main {
public static void main(String[] args) {
int foo = 12, bar = 30;
System.out.println(foo + bar);
}
}
Output:
42
Main Article: Declaring Multiple Variables in Java
Data Types
There are two classifications of data types in Java: primitive and reference.
Primitive data types are used to store numbers and simple values like Booleans (true/false values) and characters. Reference data types are used to store more complex values and include strings and arrays.
The following example shows how to use some of the most popular data types:
boolean isJavaFun = true; // Boolean
char grade = 'A'; // Character
int number = 42; // Integer
float pi = 3.14; // Float
String text = "Hello!"; // String
int[] numbers = {1, 2, 3}; // Array
You can learn more about both primitive and reference data types in the linked tutorial below.
Main Article: Java Data Types
Numbers
There are several data types that can be used to store numbers, in Java, including byte, short, int, long, float, and double. The first four (byte, short, int, and long) are used to store integers, while float and double are used to store floating-point (decimal) numbers.
public class Main {
public static void main(String[] args) {
byte myByte = 42;
short myShort = 128;
int myInt = 42;
long myLong = 4242L;
float myFloat = 42.42f;
double myDouble = 3.14159d;
}
}
Main Article: Numbers in Java
Booleans
Booleans are used to hold binary ‘true’ or ‘false’ values. They can be used to represent the state of any binary system, like ‘on’ or ‘off’, or ‘1’ or ‘0’. And they are incredibly efficient, requiring only a single bit.
boolean isJavaFun = true;
boolean isCodingEasy = false;
Booleans are frequently used in conditional expressions like if/else, for, and while. They allow the evaluation of complex comparison (greater than, less than, equal to) and logical (AND, OR, NOT) operations. Building effective programs often requires combining these features in order to control how code executes; this is called control flow, which we will see later in much greater detail.
Main Article: Booleans in Java
Characters
The char type is used to store a single 16-bit Unicode character. They are enclosed in single quotes:
char grade = 'A';
char dollar = '$';
Main Article: Characters
Strings
A string is an object that represents a sequence of characters. To create a string, we can simply declare a string type variable and initialize it with a sequence of characters inside double quotes:
String myStr = "Hello, World!";
This creates what is known as a string literal (we’ll learn about another type of string later in this tutorial). String literals are easy to use and efficient.
Strings can be concatenated using the ‘+’ operator:
String firstName = "John";
String lastName = "Doe";
String fullName = firstName + " " + lastName;
There are also many methods available to help us work with strings in Java. These methods allow us to get the length of a string, slice or split part of a string, access or replace a character or substring by index, and compare strings – just to name a few!
To learn more about strings and string methods, check out the main article linked below!
Main Article: Strings