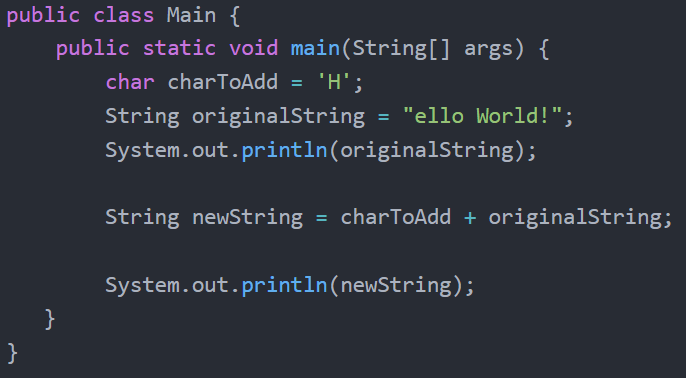
String manipulation is a fundamental aspect of Java programming, and there are countless scenarios where you may need to add a character to a string.
Whether you want to prepend a character to the beginning of a string, insert it into the middle of the string, or concatenate it to the end, there are several different ways of adding a character to a string.
In this article, we will delve into several different methods of adding a character to a string in Java.
We will cover three main approaches: (1) utilizing the simple yet effective ‘+’ concatenation operator, (2) leveraging the power of substring() to insert characters at specific positions, and (3) harnessing the versatility of the StringBuilder class for efficient string modifications.
Here’s a general summary of the important points:
- The concatenation operator works well for simply adding a character to the beginning or end of a string.
- To add a character to the middle of a string based on index, we can use substring()
- The StringBuilder class is a powerful way of working with strings. It gives us mutability and lots of useful methods for working with strings, including a few that can be used to add a character anywhere in a string.
By the end of this article, you will have gained a comprehensive understanding of the tools at your disposal, and hopefully learned something about working with characters and strings.
Whether you are a beginner trying to build your foundational knowledge or an experienced developer looking for a quick reference, this article will provide you with the understanding to choose the best tool for the job when adding characters to strings. Let’s dive in and learn how to seamlessly integrate characters into your strings in Java.
Characters vs. Strings
Before we dive into the techniques for adding a character to a string in Java, let’s take a step back and compare them. Their differences go beyond single vs. double quotation marks.
In Java, a character (`char`) represents a single Unicode character. It is enclosed in single quotes (e.g., ‘A’). It’s a primitive data type that is immutable, and always has a length of 1. Characters are used for storing individual characters or representing characters in primitive data types. Comparisons can be made using operators like `==`.
In contrast, a string (`String`) represents a sequence of characters in double quotes (e.g., “Hello, World!”). It’s a class in Java, is immutable, and the length of the string can be something other than ‘1’. Strings are used for working with text and sequences of characters. They can consume a lot more memory, especially longer strings. Comparisons of `String` objects should be done using the `.equals()` method since `==` compares object references, not content.
The Best/Easiest/Most Reliable Ways to Add a Character to a String in Java
We’ll be covering the following methods, and we’ll see how to use each of them to add a character to the beginning, middle, or end of a string in Java:
- The ‘+’ concatenation operator: We can use ‘+‘ to add a character anywhere into a string.
- The substring() method: The substring() method can be used to add a character to a specific position in a string.
- The StringBuilder class: The StringBuilder class contains several methods that can be used to precisely add a character to a string.
Add a char to a string Using the ‘+’ Operator
The plus symbol ‘+’ isn’t just used for numeric addition; it can also be used in many situations involving strings and characters. When used with strings or characters, the plus symbol ‘+’ can be used to concatenate sequences, and is therefore known as the ‘concatenation operator’.
The ‘+’ concatenation operator can be used to add a character to the beginning, middle, or end of a string in Java. It’s the most efficient method for adding a character to the beginning or end of a string.
Add a char to Beginning of a String Using the ‘+’ Operator
We can add a character to the beginning of a specified string using the ‘+’ operator.
In the following example, we first create the string ‘originalString’ which contains the value “ello World!”. We need to add the character ‘H’ to the beginning of the string to complete it.
public class Main {
public static void main(String[] args) {
char charToAdd = 'H';
String originalString = "ello World!";
System.out.println(originalString);
String newString = charToAdd + originalString;
System.out.println(newString);
}
}
Output:
ello World!
Hello World!
In this example, we created a character variable called ‘charToAdd’ with the value ‘H’ inside single quotation marks. Then we used the ‘+’ operator to add it to ‘originalString’, making it the first character of the string, which was saved into a new string variable ‘newString’.
The resulting string contained the entire text “Hello World!”. We can see how the ‘+’ string concatenation operator made it easy to add a character to the start of a string.
Add a char to the Middle of a String Using the ‘+’ Operator
If you are creating a new string, you can add a char into the middle of the string during the variable initialization:
public class Main {
public static void main(String[] args) {
char charToAdd = 'W';
String myString = "Hello " + charToAdd + "orld!";
System.out.println(myString);
}
}
Output:
Hello World!
This method has limited usefulness, as it doesn’t allow us to easily add a character into the middle of an existing string. For this, we can use substring() along with the ‘+’ operator to precisely insert a char to any specified index in the string. Usage of substring() is covered in more detail just below.
Add a char to the End of a String Using the ‘+’ Operator
Similar to adding a character to the beginning of the string, it’s very easy to use the ‘+’ concatenation operator to add a char to the end of a string.
public class Main {
public static void main(String[] args) {
char charToAdd = '!';
String originalString = "Hello World";
System.out.println(originalString);
String newString = originalString + charToAdd;
System.out.println(newString);
}
}
Output:
Hello World
Hello World!
Here, we append the exclamation mark ‘!’ to the end of the original string “Hello World”.
These examples have demonstrated how we can use the ‘+’ concatenation operator to modify strings by adding characters at different positions. It’s the easiest way of adding a character to the beginning or end of a string.
Add a char to the Middle of a string Using the substring() Method
We’ve seen that the ‘+’ concatenation operator can be used to add a character to a string, but this method is a bit limited when it comes to adding a character into the middle of an existing string.
For this, we can use the `substring()` method to add a `char` to the middle of a string in Java by splitting the string into parts and inserting the `char` wherever it’s needed.
The substring() method creates a slice of a string. It can take either the starting index or the starting and ending index. If provided with the start index only, it will include the rest of the original string in the slice:
myString.substring(startingIndex);
myString.substring(startingIndex, endingIndex);
The following example should better explain usage of substring():
public class Main {
public static void main(String[] args) {
String originalString = "Hello, World!";
System.out.println("Original: " + originalString);
String hello = originalString.substring(0,5);
System.out.println("Slice 1: " + hello);
String world = originalString.substring(7);
System.out.println("Slice 2: " + world);
}
}
Output:
Original: Hello, World!
Slice 1: Hello
Slice 2: World!
Now that we know how the substring method works, let’s see how we can use it to insert a single character into the middle of a string:
public class Main {
public static void main(String[] args) {
String originalString = "Hello, orld!";
System.out.println(originalString);
char charToAdd = 'W';
int insertIndex = 7; // Position for character insertion
String newString = originalString.substring(0, insertIndex) + charToAdd + originalString.substring(insertIndex);
System.out.println(newString);
}
}
Output:
Hello, orld!
Hello, World!
Here, we insert the `char` ‘W’ into the middle of the original string “Hello, orld!” at index 7 using `substring()`.
The `substring()` method allows you to manipulate strings by extracting portions of the original string and combining them with the desired character or string, effectively adding characters at different positions.
Add a char to a string Using the StringBuilder Class
The `StringBuilder` class is part of the Java Standard Library and is used for creating and manipulating mutable strings. One of the great things about it is that it allows us to modify the contents of a string without creating a new object each time.
We can use the `StringBuilder` class in Java to add a character to the beginning, middle, or end of a string.
StringBuilder is also designed for efficiency. When you modify the content of a StringBuilder object, it does not create new objects for each modification. Instead, it dynamically resizes its internal buffer to accommodate changes. This can significantly improve performance when you need to concatenate or modify strings repeatedly.
Java also has another class called `StringBuffer`. StringBuffer is similar to `StringBuilder` but is thread-safe (synchronized). In most cases, `StringBuilder` is preferred over `StringBuffer` because it offers better performance. However, if thread safety is a requirement, you can also use `StringBuffer`.
`StringBuilder` is particularly useful in scenarios where you need to perform multiple string operations or within a loop or when building complex strings efficiently.
Adding a Character to the Beginning of a String:
We can add a character to the beginning of a string using the insert() method. It takes two arguments: the index, and the character.
The following Java code shows how to create a new stringBuilder object and use it to add a character to the beginning of a string.
public class Main {
public static void main(String[] args) {
char charToAdd = 'H';
String originalString = "ello world!";
System.out.println(originalString);
StringBuilder stringBuilder = new StringBuilder(originalString);
stringBuilder.insert(0, charToAdd);
String newString = stringBuilder.toString();
System.out.println(newString);
}
}
Output:
ello world!
Hello world!
Adding a Character to the Middle of a String
In the previous example, we saw that we can use the insert() method to add a character to the beginning of a given string by specifying an index of 0. We can also use the insert() method to add a character to any part of the string by specifying an index other than 0. The following code shows how we can take advantage of StringBuilder to do this:
public class Main {
public static void main(String[] args) {
char charToAdd = 'W';
String originalString = "Hello orld!";
System.out.println(originalString);
StringBuilder stringBuilder = new StringBuilder(originalString);
stringBuilder.insert(6, charToAdd);
String newString = stringBuilder.toString();
System.out.println(newString);
}
}
Output:
Hello orld!
Hello World!
Here, we create a `StringBuilder` based on the original string “Hello, orld!” and use the `insert()` method to add the character ‘W’ at index 6, inserting it into the middle of the string.
Adding a Character to End of String:
public class Main {
public static void main(String[] args) {
char charToAdd = '!';
String originalString = "Hello World";
System.out.println(originalString);
// Create the StringBuilder object sbString:
StringBuilder sbString = new StringBuilder(originalString);
// Append the character and print:
sbString.append(charToAdd);
System.out.println(sbString);
}
}
Output:
Hello World
Hello World!
In this case, we create a `StringBuilder` based on the original string “Hello” and then use the `append()` method to add the exclamation point ‘!’ to the end of the string.
Using `StringBuilder` is a more efficient way to manipulate strings when you need to make multiple modifications because it avoids the overhead of creating new string objects with each change.
Conclusion
Mastering the art of basic string and character manipulation is a fundamental skill for any Java developer. In this comprehensive guide, we’ve explored several techniques for adding a character to any point in a string, a common task.
We started by discussing the simple yet effective use of the ‘+’ concatenation operator. We saw that ‘+‘ is the simplest way to append a character to the beginning or end of a string, but that its usefulness is limited when attempting to insert a character into the middle of an existing string.
Next, we delved into the use of the `substring()` method, demonstrating how it enables us to insert characters at specific positions within a string, providing fine-grained control over string manipulation.
Finally, we explored the power of the `StringBuilder` class, a versatile tool for efficiently constructing and modifying strings. With `StringBuilder`, we can effortlessly add characters to the beginning, middle, or end of a string while optimizing performance by avoiding the creation of unnecessary string objects.
By mastering the above methods, you will be well-prepared to tackle a wide range of string and character manipulation tasks, from simple concatenation to more complex text processing operations, allowing you to unlock the full potential of Java’s string handling capabilities.
If you have enjoyed this Java programming tutorial, be sure to check out our other Java tutorials and full course!