How to Compare Two Characters in Java
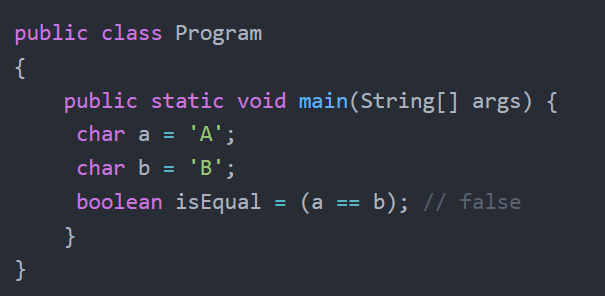
When working with characters in the Java programming language, we often need to compare them to determine equality, order, or perform other operations based on their values. However, comparing characters in Java requires consideration of the differences between character primitives and character objects.
In this article, we will delve into the details of how to compare two characters in Java. We’ll explore and contrast char primitives and char objects, and see a number of methods for comparing characters in each scenario. This will help shed light on the techniques and best practices that can be used to ensure accurate and efficient character comparisons.
Throughout this article, we will provide code examples and explanations to illustrate the different techniques for comparing characters, enabling you to make informed decisions based on the requirements of your Java projects.
By the end of this exploration, you will have a comprehensive understanding of the various ways to compare characters in Java, allowing you to choose the most appropriate method for your specific programming needs. Let’s dive in and unravel the mysteries of comparing two characters in the world of Java!
Characters in Java: Char Primitives vs. Char Objects
To begin, let’s briefly get into the fundamental distinction between char primitives and char objects in Java.
Char Primitives
In Java, a char primitive represents a single 16-bit Unicode character. It is a primitive data type and can store any character from the Unicode character set, making it suitable for most character-related operations.
Comparing char primitives involves examining their integer values, which correspond to their Unicode representations.
Char Objects
Char objects are instances of the `Character` class, a wrapper class that provides additional functionality and methods for working with characters.
These objects encapsulate a char primitive within them, allowing for more complex operations such as conversions, parsing, and formatting.
Char Primitives vs. Char Objects
Now that we’ve defined character primitives and character objects in Java, let’s compare them so that we can better understand how they actually work.
Char primitives and char objects have differences in terms of representation, behavior, and usage.
The following table outlines these differences:
Char Primitives | Char Objects | |
Representation | 16-bit Unicode character | Instance of the ‘Character’ class (wrapper for a char primitive) |
Features | Lightweight; primitive; limited operations | Require more memory; more operations are available |
Flexibility/ Functionality | Excel at simple comparison operations | Wider range of methods can be used, including case conversion and many others |
Comparing Two Char Primitives in Java
In this section we will cover several different ways to compare char primitives in Java. These include: using relational (<, >, ==) operators, using the Character.compare() method, and using the Character.hashcode() method.
Which one to use depends on what you are trying to do:
- Relational operators compares the Unicode value of two char primitives and returns a Boolean value (true or false).
- Character.compare(char x, char y) compares two char primitives and returns an integer.
- The Character.hashcode(char) method compares two char primitives based on their hash codes.
Using Relational (<, >, ==) Operators
In Java, we can use relational operators to compare char primitives directly. This is often a great solution for small programs, is very easy and can be surprisingly powerful. For these reasons, the use of relational operators wherever practical is common practice.
Relational operators evaluate the Unicode value of each character and can be used to determine equality, order, or perform other comparisons.
When using these methods, we need to keep in mind that the Unicode values are different for upper and lowercase characters. Upper case characters start with a Unicode value of ’65’, while lower case characters start with a Unicode value of ’97’. This means that we can’t compare them directly. However, we could use an offset of ’32’ to perform case-insensitive comparisons between upper and lowercase characters.
Here’s how we can use relational operators to compare char primitives in Java:
Equality Comparison:
The ‘==’ (equal) operator compares if two char primitives have an equal value. It has a return value of `true` if the characters have the same Unicode value and `false` if they do not. For example:
public class Program
{
public static void main(String[] args) {
char a = 'A';
char b = 'B';
boolean isEqual = (a == b); // false
}
}
The boolean isEqual has a value of ‘false’ because the first char variable ‘a’ has a value of ‘A’, while the second variable ‘b’ has a value of ‘B’. This is a simple example, but we will be building on these principles throughout this article.
Note: In the other examples within this article, we will omit the ‘public class Program’ and ‘public static void main()’ lines for simplicity and brevity:
The `!=` (not equal) operator checks if two char primitives are not equal. It returns `true` if the characters have different Unicode values and `false` if they are the same. For example:
char a = 'A';
char b = 'B';
boolean isNotEqual = (a != b); // true
Order Comparison
Less Than
The `<` (less than) operator compares if one char primitive is less than another in terms of Unicode value. It returns `true` if the left operand is smaller than the right operand and `false` otherwise. For example:
char a = 'A';
char b = 'B';
boolean isLessThan = (a < b); // true
Greater Than
The `>` (greater than) operator checks if the Unicode value of one char primitive is greater than another. It returns `true` if the left operand is larger than the right operand and `false` otherwise. For example:
char a = 'A';
char b = 'B';
boolean isGreaterThan = (a > b); // false
Less Than or Equal To
The `<=` (less than or equal to) operator compares if one char primitive is less than or equal to another. It returns `true` if the left operand is smaller than or equal to the right operand and `false` otherwise.
The only difference between ‘<=’ and ‘<‘ is that ‘<=’ will evaluate to ‘true’ if the values are equal. For example:
char a = 'A';
char b = 'B';
boolean isLessThanOrEqual = (a <= b); // true
Greater Than or Equal To
The `>=` (greater than or equal to) operator checks if one char primitive is greater than or equal to another. It returns `true` if the left operand is larger than or equal to the right operand and `false` otherwise. For example:
char a = 'A';
char b = 'B';
boolean isGreaterThanOrEqual = (a >= b); // false
Remember that when using relational operators to compare char primitives, the comparison is based on their Unicode values. This means that uppercase letters have lower Unicode values than lowercase letters, and digits have lower Unicode values than letters.
By utilizing these relational operators, you can compare char primitives in Java based on their Unicode values to determine equality, order, or perform other comparisons as needed in your programs.
Using Character.compare(char x, char y)
In Java, the Character class provides the compare() method that allows us to compare two char primitives for order. The compare() method takes two char primitives as arguments and returns an int value. The following example shows how we can use the `Character.compare` method to compare char primitives in Java:
char a = 'A';
char b = 'B';
int result = Character.compare(a, b);
The `compare` method compares the Unicode value of the two char primitives. It returns an integer value with the comparison result:
- If `a` is less than `b`, compare() returns a negative value.
- If `a` is greater than `b`, compare() returns a positive value.
- If `a` is equal to `b`, compare() returns 0.
Here’s an example that demonstrates the usage of `Character.compare`:
char a = 'A';
char b = 'B';
char c = 'C';
int result1 = Character.compare(a, b); // -1
int result2 = Character.compare(b, a); // 1
int result3 = Character.compare(b, c); // -1
int result4 = Character.compare(a, a); // 0
In the example above, `result1` is -1 because the Unicode value of the first character, ‘A’ is less than that of the second character, ‘B’. In the second statement, `result2` is positive 1 because ‘B’ is greater than ‘A’. `result3` is -1 because ‘B’ is less than ‘C’. Finally, `result4` is 0 because ‘A’ is equal to ‘A’.
By using the `Character.compare` method, we can compare char primitives and obtain meaningful comparison results based on their Unicode values.
Using Character.hashcode(char)
In Java, the `Character` class provides the `hashCode(char value)` method that allows us to obtain the hash code of a char primitive.
While the `hashCode` method is primarily used for generating hash codes for objects, it can also be utilized to compare char primitives indirectly. Here’s how we can use the `Character.hashCode` method to compare character values:
char a = 'A';
char b = 'B';
int hashCodeA = Character.hashCode(a);
int hashCodeB = Character.hashCode(b);
The `hashCode(char value)` method takes a char value as an argument and returns the hash code as an int value. It calculates the hash code based on the Unicode value of the character.
By comparing the hash codes of two char primitives using the `Character.hashCode` method, we can determine whether they are equal or not.
The following code demonstrates the usage of `Character.hashCode`:
char a = 'A';
char b = 'B';
char c = 'C';
int hashCodeA = Character.hashCode(a);
int hashCodeB = Character.hashCode(b);
int hashCodeC = Character.hashCode(c);
System.out.println("Hash code of 'A': " + hashCodeA);
System.out.println("Hash code of 'B': " + hashCodeB);
System.out.println("Hash code of 'C': " + hashCodeC);
Output:
Hash code of ‘A’: 65
Hash code of ‘B’: 66
Hash code of ‘C’: 67
The example above generates and displays the hash codes of the characters ‘A’, ‘B’, and ‘C’.
Please note that while the `Character.hashCode` method can be used to compare char primitives indirectly, it is generally recommended to use direct comparison methods like relational operators (`==`, `!=`, `<`, `>`, etc.) or the Character.compare() method for character comparisons based on their Unicode values.
Comparing Two Char Objects in Java
Now that we’ve seen how to compare two char primitives, let’s move on to to char objects.
Using Character.compare(char x, char y)
In Java, the Character class provides the compare() method, which allows us to compare two char objects. The `compare` method is a static method of the character class that takes two char values as arguments and returns an int value. Here’s how we can use Character.compare() to compare char objects in Java:
Character charA = 'A';
Character charB = 'B';
int result = Character.compare(charA, charB);
The compare() method compares the Unicode values of the two char objects `charA` and `charB`. It returns an int value that indicates the comparison result:
- If `charA` is less than `charB`, the `compare` method returns a negative value.
- If `charA` is greater than `charB`, the `compare` method returns a positive value.
- If `charA` is equal to `charB`, the `compare` method returns 0.
Here’s an example that demonstrates the usage of `Character.compare` with char objects:
Character charA = 'A';
Character charB = 'B';
Character charC = 'C';
int result1 = Character.compare(charA, charB); // -1
int result2 = Character.compare(charB, charA); // 1
int result3 = Character.compare(charB, charC); // -1
int result4 = Character.compare(charA, charA); // 0
In the example above, `result1` is -1 because the Unicode value of ‘A’ is less than ‘B’. Similarly, `result2` is 1 because ‘B’ is greater than ‘A’. `result3` is -1 because ‘B’ is less than ‘C’. Finally, `result4` is 0 because ‘A’ is equal to ‘A’.
By using the Character.compare() method with char objects, you can compare their Unicode values and obtain meaningful comparison results. This can be useful when you are working with char objects or need to perform specific comparisons based on their Unicode values.
Using Character.compareTo()
In Java, the compareTo() method can be used to compare objects of the `Character` class. Here’s an example of how we can do it:
Character charA = 'A';
Character charB = 'B';
int result = charA.compareTo(charB);
In the example above, `charA` and `charB` are autoboxed to `Character` objects, allowing us to call the `compareTo` method.
Autoboxing is an automatic conversion that the Java compiler makes between primitive types and their corresponding object wrapper classes.
As with compare(), the compareTo() method compares the Unicode values of the two characters and returns an integer value:
- If the calling character (`charA` in this case) is less than the argument character (`charB`), compareTo` returns a negative value.
- If the calling character is greater than the argument character, `compareTo` returns a positive value.
- If the calling character is equal to the argument character, `compareTo` returns 0.
Here’s an example that demonstrates the usage of `compareTo` with char objects:
Character charA = 'A';
Character charB = 'B';
Character charC = 'C';
int result1 = charA.compareTo(charB); // -1
int result2 = charB.compareTo(charA); // 1
int result3 = charB.compareTo(charC); // -1
int result4 = charA.compareTo(charA); // 0
In the example above, `result1` is -1 because the Unicode value of ‘A’ is less than ‘B’. Similarly, `result2` is 1 because ‘B’ is greater than ‘A’. `result3` is -1 because ‘B’ is less than ‘C’. Finally, `result4` is 0 because ‘A’ is equal to ‘A’.
Please note that `Character.compareTo` is typically used for comparing `Character` objects rather than comparing char primitives directly. However, since char objects can be autoboxed to `Character` objects, we can also leverage this method to indirectly compare char objects in Java.
Using .equals()
In Java, the equals() method is used to compare objects for equality. While the `equals` method is not directly applicable to comparing char primitives, as with the compareTo() method, we can use it to compare char objects by autoboxing the char primitives.
Here’s how we can use the `equals` method to compare char objects in Java:
Character charA = 'A';
Character charB = 'B';
boolean isEqual = charA.equals(charB);
In the example above, `charA` and `charB` are autoboxed to `Character` objects, allowing us to call the equals() method. The equals() method compares the Unicode values of the two characters and returns a Boolean, indicating whether or not they are equal.
Here’s an example that demonstrates the usage of `equals` with char objects:
Character charA = 'A';
Character charB = 'B';
Character charC = 'C';
boolean isEqual1 = charA.equals(charB); // false
boolean isEqual2 = charB.equals(charC); // false
boolean isEqual3 = charA.equals(charA); // true
In the example above, `isEqual1` is `false` because the Unicode value of ‘A’ is not equal to the Unicode value of ‘B’. Similarly, `isEqual2` is also `false` because ‘B’ is not equal to ‘C’. On the other hand, `isEqual3` is `true` because ‘A’ is equal to ‘A’.
Using Objects.equals()
In Java, we can use the Objects.equals() method to compare two different objects. The Objects.equals() method is a static method provided by the `java.util.Objects` class, and it’s commonly used to check for object equality (including comparing char objects). Here’s how we can use `Objects.equals()` to compare char objects in Java:
Character charA = 'A';
Character charB = 'B';
boolean isEqual = Objects.equals(charA, charB);
In this example, `charA` and `charB` are being compared for equality using the Objects.equals() method. The Objects.equals() method handles null-safe comparison and returns a Boolean: `true` if both objects are equal or `false` otherwise.
Here’s an example that demonstrates the usage of Objects.equals() with char objects:
Character charA = 'A';
Character charB = 'B';
Character charC = 'C';
boolean isEqual1 = Objects.equals(charA, charB); // false
boolean isEqual2 = Objects.equals(charB, charC); // false
boolean isEqual3 = Objects.equals(charA, charA); // true
In the example above, `isEqual1` is `false` because ‘A’ is not equal to ‘B’. Similarly, `isEqual2` is `false` because ‘B’ is not equal to ‘C’. Finally, `isEqual3` is `true` because ‘A’ is equal to ‘A’.
The `Objects.equals()` method is a convenient way to compare char objects, as it takes care of handling null values and provides a reliable and consistent approach to object equality checks.
Note that if you are comparing char primitives rather than char objects, you can just use the equality operator (`==`) or the `Character.compare()` method for direct comparison.
Using charValue()
The charValue() method can be used to retrieve the underlying value of a Character object, which can then be used for comparison. Here’s an example of how to use charValue():
Character charA = 'A';
char charAValue = charA.charValue();
In the example above, the `charValue()` method is invoked `charA` to retrieve it’s primitive value. The `charAValue` variable is a char primitive that contains the char value of `’A’`. We can then perform comparison operations using this value via any of the methods we discussed above (under ‘Comparing Char Primitives’).
Conclusion
There are many different possible reasons that a Java program would require the comparison of two characters, and we’ve tried to cover most of the common scenarios and solutions here.
In this article, we’ve covered all the details behind comparing characters in Java. We’ve seen the differences between the two types of characters in Java – char primitives and char objects, and multiple methods for performing comparison operations that cover most common scenarios.
Learning Java? Check out our other Java tutorials for more awesome tips and techniques!
We’re publishing new content all the time; check out our monthly newsletter to stay up to date – sign up below!