How to get the Length of a Two Dimensional (2d) Array in Java
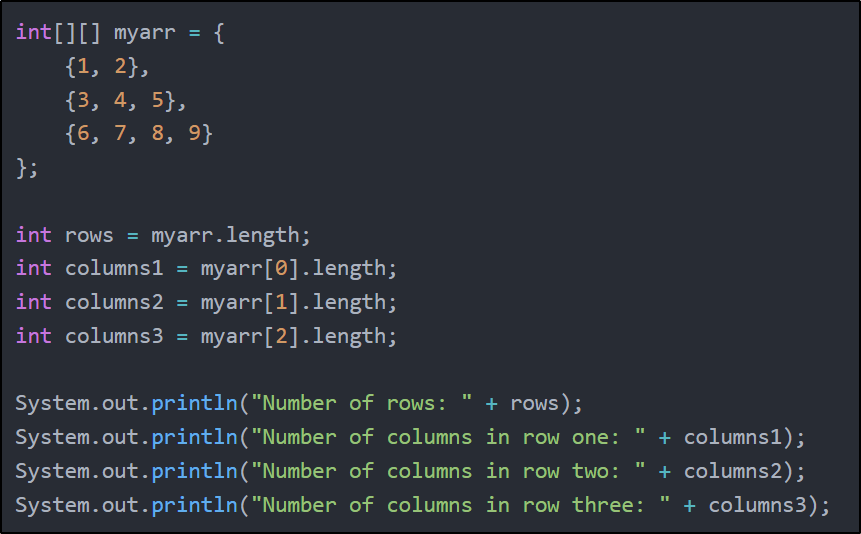
Arrays are fundamental data structures, allowing us to efficiently store and manipulate collections of values. In Java, arrays can be one-dimensional or multidimensional, providing a multitude of powerful ways to organize, access, and work with data. Two-dimensional arrays, also known as 2D arrays, are a common type of multidimensional array. 2D arrays are particularly popular because they offer a way to represent grids, matrices, and tables in a structured manner.
While working with two-dimensional arrays, it is often necessary to determine their dimensions. One crucial piece of information is the length of the array, which most commonly refers to the number of rows within the 2D structure.
In this article, we will explore the topic of 2D arrays in Java and learn how to get the length of an array. To help you avoid any confusion, this comprehensive tutorial goes well-beyond this simple operation and covers array basics, 1D arrays, how to work with 2D arrays, and even a brief overview of 3D arrays. By the end of this article, you will be on your way to mastering the topic of arrays in Java!
What is a 2d Array in the Java Programming Language?
In the Java programming language, a two-dimensional array, also known as a 2D array, is a data structure that represents a table-like structure consisting of rows and columns. Unlike a one-dimensional array that stores elements in a linear fashion, a 2D array organizes elements in a grid-like formation.
Conceptually, a 2D array can be visualized as an array of arrays. Each element within the 2D array represents a value, and the overall structure can be thought of as a matrix or a table, where each row corresponds to an array, and each column represents an element within that array.
In order to fully understand 2D arrays, let’s start with a one-dimensional array and build our understanding from there.
1-D Arrays in Java
The simplest type of array is the one-dimensional array, also known as a 1D array. A 1-D array is a data structure that allows storing a fixed number of elements of the same type in a linear sequence. When we refer to ‘array elements’, we are talking about the values within the array itself.
The 1D array is one of the most basic and commonly used data structures in Java, and serves as a foundation for more complex data structures including 2D arrays.
In Java, a 1D array is declared by specifying the data type of the elements it will hold, followed by square brackets indicating the array size. For example, to declare a 1D array of integers named “numbers” that can hold 5 elements, we can use the following syntax:
int[] numbers = new int[5];
In the above example, the array “numbers” stores 5 integers, and the indices to access its elements range from 0 to 4. Each element within the array occupies a specific position, or index, which allows for efficient retrieval and manipulation of data. We can get the length of this 1D array using the ‘length‘ attribute:
int[] numbers = new int[5];
System.out.println(numbers.length);
Output:
5
This prints ‘5’ to the console. Although this example is simple, it is important to understand because we can build on it to discover how we can get the length of a 2D array in Java.
Note: For the sake of simplicity, in this article we will be working with arrays containing elements that have integer values (int). The concepts we discuss in this article can easily be expanded while working with arrays containing elements of a different type.
2-D Arrays in Java
A 2-D array can be thought of as an array of arrays. There can be any number of arrays within the outer array, and each of these arrays can have a different number of elements.
In Java, a 2D array can be declared by specifying two sets of square brackets following the array’s type:
<data type>[][]
In math and science, a 2D array is commonly referred to as a matrix (there are some differences between a classic matrix and a 2D array but we won’t worry about them here). For example, if we want to declare a 2D array of integers named “matrix”, we can use the following syntax:
int[][] matrix;
Note that we use two square brackets ‘[][]’ after the data type. The first set of square brackets represents the first dimension, and the second set represents the second dimension. This creates a 2D array without initializing the size of its’ rows and columns.
The size of our 2D array is determined by specifying the number of rows and columns it contains. Each row can have a different number of columns, allowing for flexibility in representing irregularly shaped data structures. We can specify the number of rows and columns in the declaration in the following manner:
int[][] matrix = new int[3][4];
This creates a 2D array with 3 rows and 4 columns. Since the default value for an array of integers in Java is 0, our array would look like this:
{
{0000}
{0000}
{0000}
}
Elements within the 2D array are also accessed using two indices: one for the row and another for the column.
These two indices are represented by a set of two closed brackets (similar to the array declaration): [#][#].
The first index indicates the row and the second index indicates the column (aka the nth element within the row). We can access any of the elements of a two-dimensional array using this syntax. For example, to access the element at the second row and first column of the “matrix” array declared earlier, we can type:
int element = matrix[1][0];
As we’ve seen, in this example, the first number in square brackets represents the row number/row index, while the second number indicates the column number/column index.
Note that when we declare the size of the array by specifying its’ rows and columns, we just use the exact number of rows and columns we want (i.e. [2][3] would create an array with two rows and three columns. In contrast when we want to access an array element, the index starts with 0 (in this case [2][3] represents the element located in the third row and the fourth column of that row).
Let’s see one more example before moving on to the next topic. In the following example, we are creating a 2D array of integers with dimensions 3×4 and then initialize three of its elements:
int[][] matrix = new int[3][4];
matrix[0][0] = 1;
matrix[0][1] = 2;
matrix[1][2] = 3;
In this example we are setting the values of the first element in the first row, the second element in the first row, and the third element in the second row. All of the other elements are equal to zero, the default value.
Note: In the examples so far, we have created two-dimensional arrays in which every row has the same number of columns. This doesn’t have to be the case! In Java, 2D arrays can have rows with different lengths. We can demonstrate this easily by specifying the values of each row and column in the declaration.
In the following example, the first row only has two elements while the second row contains three and the third row has four:
int[][] myarr = {
{1, 2},
{3, 4, 5},
{6, 7, 8, 9}
};
System.out.println(myarr[2][3]);
Output:
9
This prints ‘9’. But if we try to print the fourth element from the second row, we will get an Out Of Bound Error:
int[][] myarr = {
{1, 2},
{3, 4, 5},
{6, 7, 8, 9}
};
System.out.println(myarr[1][3]);
Output:
Exception in thread “main” java.lang.ArrayIndexOutOfBoundsException: Index 3 out of bounds for length 3 at Program.main(Program.java:14)
This is because the second row of our 2-dimensional array only has three elements!
Now let’s move on to getting the length of a 2D array.
Getting the Length of a 2d Array in Java
In our 1D array example, we saw that getting its’ length was easy to define and visualize, because a one-dimensional array is a simple collection of elements and the length is just the total number of elements in the array.
To get the length of a 2D array in Java, we need to consider both dimensions: the number of rows and the number of columns in each row. There are therefore technically multiple possible ‘lengths’ of a 2D array. Generally, ‘length’ most often refers to the number of rows, but it could also refer to the number of columns in a given row.
In order to add clarity to the following examples, it may help to remember that a 2D array can be thought of as ‘an array of arrays’. We can get the length of the ‘outer’ array, or the length of any of the ‘inner’ arrays.
1. Getting the Length of a 2D array in Java:
As we’ve seen, the length of a 2D array most commonly refers to the number of rows. We can also think of this as getting the length of the outer array (in our ‘array of arrays’ model).
To get the number of rows, we can use the `length` property directly on the array variable. Here’s an example:
int[][] matrix = {
{1, 2, 3},
{4, 5, 6}
};
int rows = matrix.length;
System.out.println("Number of rows: " + rows);
“`
Output:
2
In this example, `matrix.length` returns the number of rows in the `matrix` array, which is 2. This array has 2 rows: {1, 2, 3} and {4, 5, 6}. Thus the length of the two-dimensional array in this example is two (2).
2. Getting the Length of a Row in a 2D Java array:
The wording can get a little tricky here. But what we are really doing is getting the length of a specific row.
In order to get the length of a row in a 2D array, we need to use the `length` property on that specific row. Since each row in a 2D array can have a different number of columns, we need to specify which row we are referring to in order to determine the number of columns. Here’s an example:
int[][] myarr = {
{1, 2},
{3, 4, 5},
{6, 7, 8, 9}
};
int rows = myarr.length;
int columns1 = myarr[0].length;
int columns2 = myarr[1].length;
int columns3 = myarr[2].length;
System.out.println("Number of rows: " + rows);
System.out.println("Number of columns in row one: " + columns1);
System.out.println("Number of columns in row two: " + columns2);
System.out.println("Number of columns in row three: " + columns3);
Output:
Number of rows: 3
Number of columns in row one: 2
Number of columns in row two: 3
Number of columns in row three: 4
In this example, we retrieved the length of the entire array, followed by the lengths of all three rows.
Remember that the length of a 2D array is dynamic and can vary depending on how you initialize or modify the array. These approaches provide a way to retrieve the current dimensions of the 2D array at runtime.
By utilizing these methods, we can accurately determine the length of rows and columns in a 2D array in Java, enabling you to perform operations or iterate through the array effectively.
Helpful Tricks for Working With 2d Arrays in Java
Here are a small collection of helpful properties and methods you can use when working with 2D arrays in Java:
- `length`: As we’ve seen, the `length` property allows you to retrieve the number of rows in a 2D array.
- `array[index].length`: This allows us to get the length of any row in a 2D array.
- `Arrays.deepToString(array)`: This method from the `java.util.Arrays` class allows us to print the contents of a 2D array in a readable format. It converts the array into a string representation, allowing us to see the elements of the array along with the row and column structure.
- `Arrays.fill(array, value)`: The `fill()` method allows you to set all elements in a 2D array to a specified value. By passing the array and the desired value as arguments to fill(), we can quickly fill the entire array with that value.
- `System.arraycopy(src, srcPos, dest, destPos, length)`: This method can be used to copy elements between 2D arrays or within the same array. It allows us to specify the source array, source position, destination array, destination position, and the number of elements to be copied.
- `Arrays.copyOf(array, length)`: This method creates a new 2D array with a specified length, and copies the elements from the original array. It is useful when you want to resize or create a partial copy of an existing 2D array.
- `Arrays.sort(array, comparator)`: If we need to sort the elements in a 2D array based on specific criteria, we can use the `sort()` method from the `java.util.Arrays` class. We can provide a custom comparator to define the sorting order.
These properties and methods can help us perform various operations on 2D arrays in Java. By leveraging these tools, we can efficiently work with the elements, dimensions, and contents of our 2D arrays. Hopefully this got your wheels spinning about the possibilities when working with 2D arrays!
3D Arrays in Java
While working with 2D arrays, it can be helpful to contrast them with three-dimensional arrays in order to deepen our understanding.
A three-dimensional array, also known as a 3D array, is multi-dimensional array that extends the concept of a two-dimensional array to include an additional dimension. It allows for the storage and organization of elements in a three-dimensional space, resembling a cuboid or a block of data. You can think of a 3D as an array within an array within an array! In other words, it is a structure of arrays 3 layers deep.
In Java, a 3D array can be declared by specifying three sets of square brackets after the array’s type. For example, to declare a 3D array of integers named “cube” we can use the following code:
int[][][] cube = new int[3][3][3];
In this example, the “cube” array can hold 3 layers, each with 3 rows and 3 columns. Elements within the 3D array are accessed using three indices: one for the layer, one for the row, and one for the column.
For example, to access the element at the second layer, third row, and fourth column of the “cube” array declared earlier, you would use the following syntax:
int element = cube[1][2][3];
3D arrays are useful for representing data structures that have three dimensions, such as 3D grids, volumetric data, or any scenario that requires organizing data in a three-dimensional space.
While 3D arrays introduce an additional level of complexity compared to one-dimensional and two-dimensional arrays, they expand the possibilities of data organization and enable the representation of intricate data structures within Java programs.
Conclusion
In this article, we’ve gone well beyond the basic knowledge required to get the length of a 2D array in Java. We reviewed how to create a 1D and get its’ length, and how to create a 2D array and how a 2D array can be visualized. We saw how to get the length of a 2D array, as well as how to get the length of any row in a 2D array. Finally, we briefly covered 3D arrays in order to deepen our understanding of how multi-dimension arrays work in general.