How to Print an ArrayList in Java
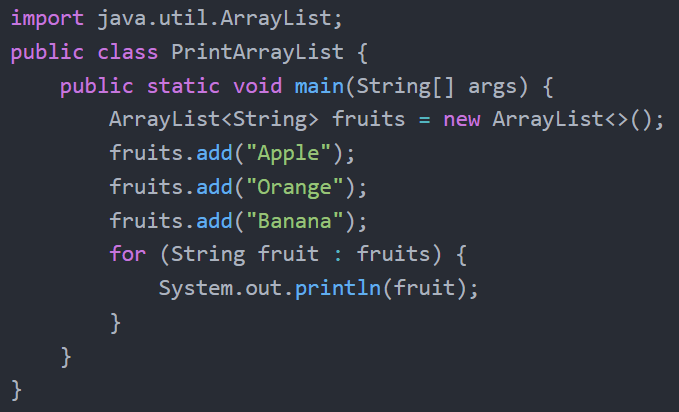
One commonly used data structure in Java is the ArrayList, a dynamic array that can grow or shrink as needed. Printing the contents of an ArrayList is a common task, and is often used for debugging, displaying results to users, generating reports, and other tasks. In this comprehensive guide, we’ll explore various methods and techniques for effectively printing an ArrayList in Java.
In this article, we will first look at an overview of the ArrayList data structure and how it is used in Java. Next, we’ll look at a few different ways of printing a single element of an ArrayList, printing part of an ArrayList, and printing an entire ArrayList. Finally, we’ll see a number of different methods and techniques that can be used for printing an a ArrayList. These include Java Streams, Iterators, using the toString() method, and using String.join().
By the end of this article, you’ll have a thorough understanding of these methods and the flexibility to choose the one that best suits your project’s needs. Whether you’re new to Java programming or an experienced developer looking to improve your ArrayList printing skills, this guide will equip you with the knowledge to handle this common task effectively.
What is an ArrayList in Java?
Before we dive into the details of printing an ArrayList, let’s review what it is. An ArrayList is a dynamic data structure that is part of the Java Collections Framework. It’s an implementation of the List interface, which allows you to store and manipulate a collection of elements. Unlike basic arrays in Java, ArrayLists can grow or shrink in size dynamically as elements are added or removed, making them a flexible choice for managing lists of data. An ArrayList can be thought of as a resizable array.
Here are some key characteristics and features of ArrayLists in Java:
1. Dynamic Sizing: Standard arrays have a fixed size, but ArrayLists resize themselves to accommodate the number of elements you add to them. You don’t need to specify the size of the ArrayList in advance.
2. Ordered: You can access elements by their index.
3. Duplicates: ArrayLists may contain duplicate elements, meaning you can have multiple elements with the same value.
4. Generics: ArrayLists can be parameterized with a specific data type using Java generics. This ensures type safety, so an ArrayList can’t contain elements of the wrong type.
Here’s an example of how to create and use an ArrayList in Java:
import java.util.ArrayList;
public class ArrayListExample {
public static void main(String[] args) {
// Creating an ArrayList of Strings
ArrayList<String> fruits = new ArrayList<>();
// Adding elements to the ArrayList
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Orange");
// Accessing elements by index
System.out.println("First fruit: " + fruits.get(0));
System.out.println("Second fruit: " + fruits.get(1));
// Iterating through the ArrayList
for (String fruit : fruits) {
System.out.println("Fruit: " + fruit);
}
// Removing an element
fruits.remove("Banana");
// Checking if an element exists
if (fruits.contains("Banana")) {
System.out.println("Banana is in the list.");
} else {
System.out.println("Banana is not in the list.");
}
// Getting the size of the ArrayList
System.out.println("Number of fruits: " + fruits.size());
}
}
Output:
First fruit: Apple
Second fruit: Banana
Fruit: Apple
Fruit: Banana
Fruit: Orange
Banana is not in the list.
Number of fruits: 2
This code demonstrates some of the basic operations you can perform with an ArrayList, including adding elements, accessing elements, removing elements, checking for element existence, and getting the size of the ArrayList.
Printing an ArrayList in Java
You may want to print a single element of an ArrayList, a slice of an ArrayList, or the entire ArrayList. Let’s look at simple ways of accomplishing each of these.
Print Elements of ArrayList
To print individual elements of an ArrayList, we can access them by their index using the get() method. In the following simple example, we create an ArrayList called fruit, and then populate it with our three favorite fruits. We then print each element to the console individually.
import java.util.ArrayList;
public class PrintArrayList {
public static void main(String[] args) {
ArrayList<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Orange");
fruits.add("Banana");
System.out.println("Fruit 1: " + fruits.get(0));
System.out.println("Fruit 2: " + fruits.get(1));
System.out.println("Fruit 3: " + fruits.get(2));
}
}
Output:
Fruit 1: Apple
Fruit 2: Orange
Fruit 3: Banana
This method allows for a fine degree of control, but has some important limitations as well.
Print Part of an ArrayList
To print part of an ArrayList we can use the get() method along with a for loop. The for loop will iterate from the starting index to the ending index, printing each element consecutively. We must provide integer values specifying the starting and ending indices.
Note that in this example, the element at the starting index is included in the slice while element at the ending index is excluded.
import java.util.ArrayList;
public class PrintArrayList {
public static void main(String[] args) {
ArrayList<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Orange");
fruits.add("Grape");
fruits.add("Kiwi");
int startIndex = 1; // Inclusive
int endIndex = 4; // Exclusive
// Print a slice of the ArrayList
System.out.println("Slice of ArrayList:");
for (int i = startIndex; i < endIndex; i++) {
System.out.println("Element " + i + ": " + fruits.get(i));
}
}
}
Output:
Slice of ArrayList:
Element 1: Orange
Element 2: Banana
Element 3: Grape
In this example, we specified a starting index of 1 and an ending index of 4. The first and last element of the original ArrayList have both been excluded from the print operation. We can use this method to print out any ‘slice’ of an ArrayList.
Print a Whole ArrayList
To print an entire ArrayList, we can iterate through it using a for-each loop similarly to the previous example. However this time, the for loop will just iterate over the entire ArrayList.
import java.util.ArrayList;
public class PrintArrayList {
public static void main(String[] args) {
ArrayList<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Orange");
fruits.add("Banana");
for (String fruit : fruits) {
System.out.println(fruit);
}
}
}
Output:
Apple
Orange
Banana
Methods for Printing an ArrayList in Java
The above methods aren’t the only way of printing an ArrayList. In this section, we will briefly cover several commonly used methods for printing an ArrayList:
Using a for-each Loop
This is the same technique that we saw above. We can use a for-each loop to iterate through an ArrayList and print each element individually.
ArrayList<String> list = new ArrayList<>();
// Add elements to the ArrayList
for (String element : list) {
System.out.println(element);
}
Using a Traditional for Loop
We can also use a traditional for loop to iterate through the ArrayList by index and print each element. In the following example, we are using the size() method so that the ‘for’ loop will iterate until it prints the last element. This works because the index of the last element is one less than the size of the list.
ArrayList<String> list = new ArrayList<>();
// Add elements to the ArrayList
for (int i = 0; i < list.size(); i++) {
System.out.println(list.get(i));
}
Using Java Streams
We can use Java Streams to print the contents of an ArrayList by applying the `forEach` method on the stream. Here’s an example:
import java.util.ArrayList;
import java.util.List;
public class PrintArrayListWithStreams {
public static void main(String[] args) {
ArrayList<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
list.add("Orange");
// Use Java Streams to print the ArrayList
list.stream().forEach(element -> System.out.println(element));
}
}
Output:
Apple
Orange
Banana
In this example, we first created an ArrayList called `list` and add some elements to it. Next we converted the ArrayList into a Stream using the `stream()` method. Finally, we used the `forEach` method to iterate through the elements and print each to the console. The lambda expression `element -> System.out.println(element)` defines the action to be performed on each element – which in this case is printing it.
Using Java Streams can make your code more concise and expressive, especially when you need to perform more complex operations on the elements of the ArrayList.
Using an Iterator
An `Iterator` is an interface that provides a way to iterate over a collection of objects, such as an ArrayList, without having to expose the underlying data structure’s implementation details. We can use an Iterator to access elements sequentially, one at a time, and perform operations on them.
To use an `Iterator` to print the elements of an ArrayList in Java, you can follow these steps:
- Create an ArrayList and populate it with elements.
- Obtain an Iterator for the ArrayList via the `iterator()` method.
- Use a loop to iterate through the ArrayList using the iterator.
- Inside the loop, retrieve each element using the `next()` method and print it.
Here’s an example:
import java.util.ArrayList;
import java.util.Iterator;
public class PrintArrayListWithIterator {
public static void main(String[] args) {
ArrayList<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
list.add("Orange");
// Get an Iterator for the ArrayList
Iterator<String> iterator = list.iterator();
// Iterate through the ArrayList using the iterator
while (iterator.hasNext()) {
String element = iterator.next();
System.out.println(element);
}
}
}
In this example, we created an ArrayList called `list` and added some elements to it. We then obtain an Iterator for the ArrayList using the `iterator()` method. Next we used a `while` loop to iterate through the ArrayList using the iterator. The `hasNext()` method checks if there are still more elements in the ArrayList, and `next()` retrieves the next element in the iteration. Still inside the loop, we print each element to the console.
Using an Iterator is a common and efficient way to traverse and perform operations on the elements of a collection like an ArrayList without needing to know the underlying implementation details.
Using the `toString` Method
The `toString()` method is a method defined in the `Object` class and is used to provide a string representation of an object. When we call `toString()` on an object, it returns a string that represents the object’s state.
By default, the `toString()` method in the ArrayList class returns a string representation of the ArrayList’s contents with each element enclosed in square brackets and separated by commas.
The following code shows how to use the `toString()` method to print an ArrayList:
import java.util.ArrayList;
public class PrintArrayListWithToString {
public static void main(String[] args) {
ArrayList<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
list.add("Orange");
// Print the ArrayList using toString()
System.out.println(list.toString());
}
}
Output:
[Apple, Banana, Orange]
We can see that `toString()` is a helpful method of ArrayList and provides a convenient way to print the entire ArrayList on a single line. However, this format may not always be suitable if you need more control over formatting or if you want to perform operations on individual elements of the ArrayList. In such cases, other methods like using a loop or Java Streams may be more appropriate.
Using `String.join`
In Java 8 and later versions, the `String.join()` method provides a convenient way to concatenate elements of a collection, such as an ArrayList, into a single string with a specified delimiter. It can be used to create a formatted string representation of an ArrayList’s contents.
Here’s how we can use `String.join()` to print the elements of an ArrayList with a delimiter:
import java.util.ArrayList;
import java.util.List;
public class PrintArrayListWithJoin {
public static void main(String[] args) {
ArrayList<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
list.add("Orange");
// Convert the ArrayList to a single formatted string
String delimiter = ", ";
String formattedString = String.join(delimiter, list);
// Print the formatted string
System.out.println(formattedString);
}
}
Output:
Apple, Banana, Orange
In this above program, we created an ArrayList called `list` and added some elements to it. Next, we specified a delimiter (comma + space, “, “) that will be used to separate the elements in the formatted string. After this, we used `String.join(delimiter, list)` to join the elements of the array list with the specified delimiter. Th String.join() method takes two arguments: the delimiter and the collection of elements to join. Finally, we printed the formatted string to the console. The output is the contents of the ArrayList on a single line, with each element separated by a comma and space.
The `String.join()` method provides a clean way to format the contents of an ArrayList into a single string using the specified delimiter. It’s especially useful when we need to build comma-separated lists, create CSV (Comma-Separated Values) strings, or generate formatted output from ArrayList elements.
Conclusion
ArrayLists are powerful dynamic arrays that unlock a huge amount of potential. In the world of Java, the art of printing an ArrayList is an essential skill. As we’ve explored in this guide, there are multiple useful methods available, each with its advantages and use cases.
We began our journey by looking at the simplest ways to print ArrayList elements and slices, as well as the whole ArrayList.
For more advanced scenarios, we ventured into the usage of traditional for loops, Java Streams, and iterators. These methods offer greater control and flexibility, allowing us to fine-tune the printing process. We looked at complete code examples for each of these to solidify our practical understanding of them. Whether we need to print specific elements, apply conditional logic, or transform data, these techniques have us covered.
We also uncovered the convenience of the `toString()` method. This default method provides a quick way to generate a string representation of an ArrayList, suitable for many use cases.
Finally, we explored `String.join()`. This method simplifies the task of formatting and printing ArrayList elements with desired delimiters, making it an excellent choice for creating CSV files, generating reports, or formatting lists for display.
With these tools and techniques at your disposal, you should be well-equipped to tackle the challenge of printing ArrayLists in Java. The choice of which method to use depends on your specific requirements and the context of your Java program, and the ability to adapt and select the most suitable approach is a hallmark of a skilled Java developer.
We hope you’ve learned something valuable from this article, whether you’re a Java beginner or expert!