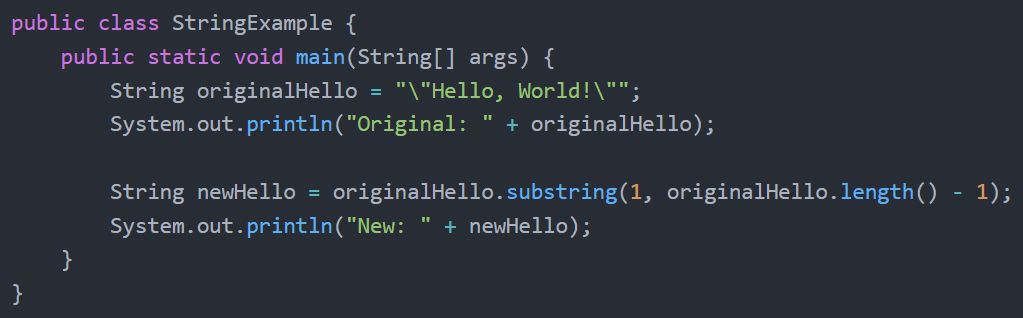
Manipulating strings is a fundamental aspect of programming, and Java offers a wide array of tools and methods to perform a wide range of operations on strings.
One common task that Java developers encounter is the need to remove quotes from strings, whether they are single quotes (”) or double quotes (“”). These quotes might be present due to data formatting, input processing, or many other reasons. Understanding how to efficiently remove quotes from strings is crucial for maintaining data integrity and consistency in your Java applications.
In this article, we will look at a variety of simple and helpful techniques to remove both single and double quotes from strings in the Java programming language. We’ll explore several different scenarios and provide step-by-step guides along with code examples to illustrate how to implement each approach. Whether you are a beginner trying to learn the basics of Java or an experienced programmer looking for a quick reference, this guide has you covered!
Introduction – Quotes and Escape Characters in Java Strings
In Java, a string is a sequence of characters encapsulated within double quotes. This can make it a bit tricky to remove quotes, because there may be situations when we need to correctly escape one or more sets of double quotes in order for our code to work.
Let’s look at the simple example of a string containing the text “Hello, World!”:
String helloWorld = "Hello, World!";
In this example, the string variable `helloWorld` holds the value “Hello, World!”. Strings in Java are sequences of characters enclosed within double quotes (“”).
We can print this string to the console with the following code:
String helloWorld = "Hello, World!";
public class StringExample {
public static void main(String[] args) {
String helloWorld = "Hello, World!";
System.out.println(helloWorld);
}
}
Output:
Hello, World!
Notice that the output text isn’t encapsulated by double quotes. The double quotes that we see in the variable initialization are used to tell Java where the string starts and ends, but they aren’t included in the actual string text.
If we want to encapsulate our string in double quotes, we can do this with the backslash escape sequence character ‘\‘. This would look like the following:
public class StringExample {
public static void main(String[] args) {
String helloWorld = "\"Hello, World!\"";
System.out.println(helloWorld);
}
}
Output:
“Hello, World!”
This time, the string is encapsulated in double quotes!
At this point, you might be wondering why this article is teaching you how to add quotes instead of removing them. The key point here is that we will need to use the escape character when dealing with double quotes. However, this is not true when dealing with single quotes:
public class StringExample {
public static void main(String[] args) {
String helloWorld = "'Hello, World!'";
System.out.println(helloWorld);
}
}
Output:
‘Hello, World!’
As we can see in the above example, we can interact directly with single quotes without using the escape character.
Now that we understand how strings in Java function with regards to single quotes, double quotes, and escape characters, let’s learn how to remove them!
Removing Single and Double Quotes Using the substring() Method
The substring() method is very powerful and versatile, and should be sufficient in most use cases.
We can use substring() to remove single or double quotes that can be found in front of the string (leading quotes), at the back of the string (trailing quotes), or both.
The substring() Method
In Java, the `substring()` method is a part of the `String` class and is used to extract part of a string. It allows us to create a new string that contains a specified range of characters from the original string.
The `substring()` method takes one or two integer parameters that define the starting and ending indices of the desired substring. The index always starts at zero.
Here’s the general syntax of Java’s `substring()` method:
String substring(int startIndex)
String substring(int startIndex, int endIndex)
- `startIndex`: The index of the character where the substring should start. The character at this index is included in the substring.
- `endIndex`: The index of the character immediately after the last character of the desired substring. This character is not included in the substring.
Remove Leading Quotes From a String With substring()
We can use the `substring()` method to remove leading quotes from a string by calling it with a starting index of 1. By using an index of 1 instead of 0, we remove the first character of the original string:
public class StringExample {
public static void main(String[] args) {
String originalHello = "'Hello, World!'";
System.out.println("Original: " + originalHello);
String newHello = originalHello.substring(1);
System.out.println("New: " + newHello);
}
}
Output:
Original: ‘Hello, World!’
New: Hello, World!’
The resulting string (newHello) is the same as the original string, but has had the leading single quote removed.
Note that this works the same way to remove a leading double quote:
public class StringExample {
public static void main(String[] args) {
String originalHello = "\"Hello, World!\"";
System.out.println("Original: " + originalHello);
String newHello = originalHello.substring(1);
System.out.println("New: " + newHello);
}
}
Output:
Original: “Hello, World!”
New: Hello, World!”
Now let’s look at how to remove trailing quotes from a string.
Remove Trailing Quotes From a String With substring()
In order to remove the trailing quote from a string, we need to call the susbtring() method with an ending index of the string length minus one.
We can do this using the .length() method on the original string. The new substring command will look like this:
myString.substring(0, myString.length() – 1)
The following example demonstrates how to do this to remove a trailing single quotation mark:
public class StringExample {
public static void main(String[] args) {
String originalHello = "'Hello, World!'";
System.out.println("Original: " + originalHello);
String newHello = originalHello.substring(0, originalHello.length() - 1);
System.out.println("New: " + newHello);
}
}
Output:
Original: ‘Hello, World!’
New: ‘Hello, World!
We can also use this method in the same exact way to remove a trailing double quote:
public class StringExample {
public static void main(String[] args) {
String originalHello = "\"Hello, World!\"";
System.out.println("Original: " + originalHello);
String newHello = originalHello.substring(0, originalHello.length() - 1);
System.out.println("New: " + newHello);
}
}
Output:
Original: “Hello, World!”
New: “Hello, World!
Now let’s put these two techniques together to remove both leading and trailing quotes.
Remove Leading and Trailing Quotes From a String With substring()
As you may have already figured out, one of the best things about substring() is that we can easily remove both leading and trailing quotes with a single statement.
To do so, we will simply call the substring() method with a starting index of 1 and an ending index of the string length minus one.
Let’s see how this works to remove single quotes:
public class StringExample {
public static void main(String[] args) {
String originalHello = "'Hello, World!'";
System.out.println("Original: " + originalHello);
String newHello = originalHello.substring(1, originalHello.length() - 1);
System.out.println("New: " + newHello);
}
}
Output:
Original: ‘Hello, World!’
New: Hello, World!
As with the previous examples, we can do the same exact thing to remove double quotes:
public class StringExample {
public static void main(String[] args) {
String originalHello = "\"Hello, World!\"";
System.out.println("Original: " + originalHello);
String newHello = originalHello.substring(1, originalHello.length() - 1);
System.out.println("New: " + newHello);
}
}
Output:
Original: “Hello, World!”
New: Hello, World!
In most scenarios, the substring() method should work well to remove quotes from a string in Java.
However, what if we have a more complex situation? For example, the string itself might also contain quotes that we want to remove, or we may have a block of text with a number of strings separated by quotes that we want to remove. For these or other situations where we need to remove quotes from a string, one of the following methods may work.
Using replace() to Remove Quotes From a String
In Java, the `replace()` method is used to replace all occurrences of a specified substring within a string with another substring. We can utilize this method to remove quotes from a string by replacing them with an empty string.
The replace() method takes in two arguments, each enclosed within double quotes:
myString.replace(” “, ” “)
The first set of quotes contains the substring that we want to search for and replace. The second set of quotes contains a substring that we want to replace it with. In other words:
myString.replace(“<substring to search and replace>”, “<substring to replace with>”)
Since we will be removing quotes and replacing them with nothing, the second set of quotes in the replace() method (i.e. the specified substring in the second argument) should be blank.
If this is difficult to visualize, the following examples should help make this clear.
Here’s an example of how to use the replace() method to remove single quotes from as string:
public class StringExample {
public static void main(String[] args) {
String originalHello = "'Hello, World!'";
System.out.println("Original: " + originalHello);
String newHello = originalHello.replace("'","");
System.out.println("New: " + newHello);
}
}
Output:
Original: ‘Hello, World!’
New: Hello, World!
We can also use the replace() method to remove double quotes. To do so, we will need to use the backslash escape character:
public class StringExample {
public static void main(String[] args) {
String originalHello = "\"Hello, World!\"";
System.out.println("Original: " + originalHello);
String newHello = originalHello.replace("\"","");
System.out.println("New: " + newHello);
}
}
Output:
Original: “Hello, World!”
New: Hello, World!
The replace() method is powerful but not very versatile. It is designed to remove all instances of a substring and replace them with another substring.
The previous section covered use of the replace() method. If we want to perform a more precise replacement operation, we can use the replaceAll() method instead.
Using replaceAll() to Remove Quotes From a String
The `replaceAll()` method in Java is a part of the `String` class and is used to perform replacement based on regular expressions (regex). It parses through the whole string and replaces all occurrences of substrings that match a specified regular expression with a replacement string.
We can use the replaceAll() method using the same syntax that we used with the replace() method if we just want to remove all occurrences of a substring.
Single Quotes
For example, we can use it replace all single quotes as follows:
public class StringExample {
public static void main(String[] args) {
String originalHello = "'Hello, World!'";
System.out.println("Original: " + originalHello);
String newHello = originalHello.replaceAll("'","");
System.out.println("New: " + newHello);
}
}
Output:
Original: ‘Hello, World!’
New: Hello, World!
Double Quotes
Similarly, we can use replaceAll() to remove double quotes, keeping in mind the need to use an escape character:
public class StringExample {
public static void main(String[] args) {
String originalHello = "\"Hello, World!\"";
System.out.println("Original: " + originalHello);
String newHello = originalHello.replaceAll("\"","");
System.out.println("New: " + newHello);
}
}
Output:
Original: “Hello, World!”
New: Hello, World!
However, the strength of the replaceAll() method is that we can also use regular expressions to define the location of the substring to be removed. This means that we can use it to remove quotes from the beginning or end of a string.
Using the replaceAll() Method With Regular Expressions
To remove quotes from the beginning or end of a string using the `replaceAll()` method, we need to use a regular expression pattern that matches the quotes that we want to remove and replace them with an empty string.
Here are examples of how we can use `replaceAll()` to remove quotes from different parts of a string:
Removing Leading Quotes from the Beginning of a String With replaceAll()
To remove quotes from the beginning of a string, we can use the caret `^` symbol in our regular expression pattern, before the single or double quote. This symbol matches the start of the string.
Single Quote:
public class StringExample {
public static void main(String[] args) {
String originalHello = "'Hello, World!'";
System.out.println("Original: " + originalHello);
String newHello = originalHello.replaceAll("^'","");
System.out.println("New: " + newHello);
}
}
Output:
Original: ‘Hello, World!’
New: Hello, World!’
Double Quote:
To remove a leading double quote, we must use the ‘/’ escape character.
public class StringExample {
public static void main(String[] args) {
String originalHello = "\"Hello, World!\"";
System.out.println("Original: " + originalHello);
String newHello = originalHello.replaceAll("^\"","");
System.out.println("New: " + newHello);
}
}
Output:
Original: “Hello, World!”
New: Hello, World!”
Removing Leading Quotes from the Beginning of a String With replaceAll()
To remove quotes from the end of a string, we can use the `$` symbol in your regular expression pattern after the single or double quote. This symbol matches the end of the string.
Single Quote:
public class StringExample {
public static void main(String[] args) {
String originalHello = "'Hello, World!'";
System.out.println("Original: " + originalHello);
String newHello = originalHello.replaceAll("'$","");
System.out.println("New: " + newHello);
}
}
Output:
Original: ‘Hello, World!’
New: ‘Hello, World!
Double Quote:
For double quotes, we again need to use the escape character.
public class StringExample {
public static void main(String[] args) {
String originalHello = "\"Hello, World!\"";
System.out.println("Original: " + originalHello);
String newHello = originalHello.replaceAll("\"$","");
System.out.println("New: " + newHello);
}
}
Output:
Original: “Hello, World!”
New: “Hello, World!
In all of these examples, the `replaceAll()` method is used with regular expression patterns to match and replace the desired quotes with an empty string.
Keep in mind that regular expressions offer a powerful way to manipulate strings, but they can be complex and might require some understanding of the proper syntax and some tinkering to get it right. Always test your regular expressions thoroughly to ensure they match and replace the intended substrings.
Using the charmatcher Class from Guava
The `CharMatcher` class in Google Guava is a powerful utility class that provides a variety of methods for working with characters and strings in a concise and efficient manner.
To remove leading or trailing quotes from a string using the `CharMatcher` class, we can use its methods along with common string manipulation techniques.
In order to use any of these approaches, we will need to add the Guava library to our project:
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>${guava-version}</version>
</dependency>
Removing Quotes from a String With trimFrom()
The `trimFrom()` method can be used to remove a specified set of characters from the beginning and end of a string. To remove leading and trailing quotes from a string using `trimFrom()`, we can provide a `CharMatcher` that matches the quotes.
Here’s how we can use `trimFrom()` to remove leading or trailing quotes from a string:
import com.google.common.base.CharMatcher;
public class CharMatcherExample {
public static void main(String[] args) {
String originalHello = "\"Hello, World!\"";
System.out.println("Original: " + originalHello);
CharMatcher quoteMatcher = CharMatcher.is('"');
String newHello = quoteMatcher.trimFrom(originalHello);
System.out.println("New: " + newHello);
}
}
Output:
Original: “Hello, World!”
New: “Hello, World!
In this example, we first created a `CharMatcher` instance named `quoteMatcher` that matches double quotation marks (`”`). Then, we used the `trimFrom()` method on `quoteMatcher` to remove leading and trailing quotes from the `originalHello` string.
Remember that we must include the Google Guava library in the project in order to use the `CharMatcher` class. You can add the Guava library as a dependency in your project’s build configuration (such as Maven or Gradle).
Removing Leading Quotes from the Beginning of a String With trimLeadingFrom()
We can use the `CharMatcher`’s `trimLeadingFrom()` method to remove leading quotes from a string. Additionally, we can use the `replaceFrom()` method to remove other specific characters if needed.
The following Java program demonstrates this:
import com.google.common.base.CharMatcher;
public class CharMatcherExample {
public static void main(String[] args) {
String originalHello = "\"Hello, World!\"";
System.out.println("Original: " + originalHello);
String newHello = CharMatcher.is('"').trimLeadingFrom(originalHello);
System.out.println("New: " + newHello);
}
}
Output:
Original: “Hello, World!”
New: Hello, World!”
Removing Trailing Quotes from the End of a String With trimLeadingFrom()
Similarly, we can use the `trimTrailingFrom()` method to remove trailing quotes from a string.
import com.google.common.base.CharMatcher;
public class CharMatcherExample {
public static void main(String[] args) {
String originalHello = "\"Hello, World!\"";
System.out.println("Original: " + originalHello);
String newHello = CharMatcher.is('"').trimTrailingFrom(originalHello);
System.out.println("New: " + newHello);
}
}
Output:
Original: “Hello, World!”
New: “Hello, World!
Keep in mind that while using Guava’s `CharMatcher` is a powerful option, if you only need to remove leading or trailing quotes, the built-in methods like `replace()` or `substring()` in the standard Java `String` class can also achieve this without requiring an external library.
Conclusion – How to Remove Quotes From a String in Java
In the world of Java programming, manipulating strings is a necessity and removing quotes from strings is a common task. Throughout this article, we’ve explored various techniques for achieving this task effectively and efficiently.
From the versatile uses of the substring() method, to using the `replace()` method to remove specific quotes, and the more advanced capabilities offered by the `replaceAll()` method with regular expressions, we’ve uncovered a range of tools that don’t require any external resources at all. We learned how to address scenarios where quotes need to be removed from the beginning, end, or middle of strings, gaining a deeper understanding of string manipulation and the ways in which Java empowers us to do so.
Additionally, we’ve ventured into the Google Guava library, discovering how the `CharMatcher` class can provide an alternative solution through its versatile methods. This journey has showcased the power of Java’s built-in functionality as well as the flexibility offered by external libraries like Guava.
As we conclude this article, we can see that the ability to remove quotes from strings in Java is not merely a mechanical task, but a skill that empowers developers to maintain data integrity, streamline input processing, and create more robust applications. We may need to process a single string or a list of strings from external text files or another functionality in our program. No matter the situation, you should be covered using the information in this tutorial.