How to Round Numbers in Java
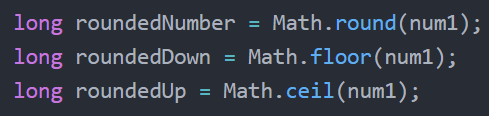
Rounding is a common task in programming, and it’s important to know what the options are in order to reduce the loss of precision as much as possible. While rounding numbers up or to the nearest integer is a common practice, there are situations where rounding down is the key to maintaining the integrity of your calculations.
In Java’s Math class, there are several helpful methods when it comes to rounding numbers:
- round() rounds to the nearest integer – either up or down using ’round half up’
- floor() can be used to round down
- ceil() can be used to round up
In this article, we will break down how each of these methods work so that you can decide which is best depending on your project.
How to Round Up or Down in Java
Having to round numbers is a common situation in programming. There are cases when we need to round up, other times when we need to round down, and also situations when we need to round to the nearest integer.
Rounding to the nearest integer can also help round-off precision (rounding error) as much as possible.
Using Java’s Math Class
Java’s `Math` class is a built-in class in the Java Standard Library that provides a collection of methods for performing mathematical operations.
These methods are static, meaning that we can call them directly on the `Math` class without needing to create an instance of it. One of the common uses of the `Math` class is for rounding numbers. Here are some of the methods it provides to help round numbers:
- Math.round(): Rounds the specified `double` value to the closest integer value. `Math.round(15.49)` returns `15`, and `Math.round(15.5)` returns `16`.
- Math.floor(): Rounds down to the nearest integer number or the integer portion of the decimal number. `Math.floor(15.5)` returns `15.0`.
- Math.ceil(): Rounds up to the nearest integer or the integer portion of the number. `Math.ceil(15.49)` returns `16.0.
- Math.floorDiv(): Returns the largest (closest to negative infinity) integer that is less than or equal to the quotient of the two arguments. `Math.floorDiv(15, 4)` returns `3`, representing the integer division of 15 by 4.
- Math.ceilDiv(): Returns the smallest (closest to positive infinity) integer that is greater than or equal to the quotient of the two arguments. `Math.ceilDiv(15, 4)` returns `4`, representing the integer division of 15 by 4 rounded up.
These methods are handy when you need to perform various mathematical operations, including rounding, in your Java programs. Depending on your specific requirements and the desired rounding behavior, you can choose the appropriate method from the `Math` class.
Rounding to the Nearest Integer in Java
The Math.round() method can be used to round to the nearest integer. This is the most common type of rounding.
The way that Math.round() works is that it adds .5 to the value and then performs a floor of the result. We will see how floor() works later in this article.
Here’s an example of how the Math.round() method works:
public class MathRoundExample {
public static void main(String[] args) {
double number1 = 15.49;
double number2 = 15.5;
// Using Math.round() to round numbers to the nearest integer
long roundedNumber1 = Math.round(number1);
long roundedNumber2 = Math.round(number2);
// Displaying the original numbers and the rounded numbers
System.out.println("Original number 1: " + number1);
System.out.println("Rounded number 1: " + roundedNumber1);
System.out.println("Original number 2: " + number2);
System.out.println("Rounded number 2: " + roundedNumber2);
}
}
Output:
Original number 1: 15.49
Rounded number 1: 15
Original number 2: 15.5
Rounded number 2: 16
In this example:
As you can see, `Math.round()` rounds `15.49` down to `15` because it’s closer to `15`, and it rounds `15.5` up to `16` because it’s closer to `16`. This demonstrates how `Math.round()` works to round to the nearest number (integer) in Java.
Rounding Down in Java
To round down, we can use the Math.floor() method.
In Java, the `Math.floor()` method is used to round a floating-point number down to the nearest integer value. It basically truncates the decimal part of the number (i.e. everything to the right of the decimal point) and returns the largest integer that is less than or equal to the original value. Here’s how it works:
public class MathFloorExample {
public static void main(String[] args) {
double number1 = 15.49;
double number2 = 15.5;
// Using Math.floor() to round numbers down to the nearest integer
double floorNumber1 = Math.floor(number1);
double floorNumber2 = Math.floor(number2);
// Displaying the original numbers and the rounded down numbers
System.out.println("Original number 1: " + number1);
System.out.println("Rounded down number 1: " + floorNumber1);
System.out.println("Original number 2: " + number2);
System.out.println("Rounded down number 2: " + floorNumber2);
}
}
Output:
Original number 1: 15.49
Rounded down number 1: 15.0
Original number 2: 15.5
Rounded down number 2: 15.0
The `Math.floor()` method rounds `15.49` down to `15.0` and `15.5` down to `15.0`. It always rounds the number down to the nearest integer or the integer part of the number. This is useful in situations where you want to discard the decimal part of a float value while always rounding down.
Rounding Up Using Math.ceil()
In Java, the `Math.ceil()` method is used to round a float number up to the nearest integer. It returns the smallest integer value that is greater than or equal to the original value. Here’s how it works:
public class MathCeilExample {
public static void main(String[] args) {
double number1 = 15.49;
double number2 = 15.5;
// Using Math.ceil() to round numbers up to the nearest integer
double ceilNumber1 = Math.ceil(number1);
double ceilNumber2 = Math.ceil(number2);
// Displaying the original numbers and the rounded up numbers
System.out.println("Original number 1: " + number1);
System.out.println("Rounded up number 1: " + ceilNumber1);
System.out.println("Original number 2: " + number2);
System.out.println("Rounded up number 2: " + ceilNumber2);
}
}
Output:
Original number 1: 15.49
Rounded up number 1: 16.0
Original number 2: 15.5
Rounded up number 2: 16.0
In this code:
1. We declare two `double` variables, `number1` and `number2`, with values `15.49` and `15.5`, respectively.
2. We use the `Math.ceil()` method to round these numbers up to the nearest integer, and the results are stored in `ceilNumber1` and `ceilNumber2`.
3. Finally, we print the original numbers along with their rounded-up counterparts.
Math.ceil() rounds both `15.49` and `15.5` up to `16.0`. It always rounds floating-point numbers up to the nearest whole number, ensuring that the result is greater than or equal to the original value. This can be useful in situations where you want to ensure a minimum value for a quantity.
Rounding Negative Numbers in Java
The round(), floor(), and ceil() methods can be used with negative numbers as well as positive numbers. However, they can have some unexpected behaviors:
The round() method stills adds .5 and then performs a floor() operation. The following example demonstrates this:
public class MathRoundExample
public static void main(String[] args) {
double number1 = -15.50;
double number2 = -15.51;
// Using Math.round() to round numbers to the nearest integer
double roundedNumber1 = Math.round(number1);
double roundedNumber2 = Math.round(number2);
// Displaying the original numbers and the rounded numbers
System.out.println("Original number 1: " + number1);
System.out.println("Rounded number 1: " + roundedNumber1);
System.out.println("Original number 2: " + number2);
System.out.println("Rounded number 2: " + roundedNumber2);
}
}
Output:
Original number 1: -15.5
Rounded number 1: -15.0
Original number 2: -15.51
Rounded number 2: -16.0
The first number, -15.5, rounds up to -15. The second number, 15.51, rounds down to -16.
The floor() and ceil() methods are more predictable. We just have to keep in mind what is up vs down when it comes to negative numbers. floor() always rounds down to the next lower negative number:
public class MathRoundExample {
public static void main(String[] args) {
double number1 = -15.50;
double roundedNumber1 = Math.floor(number1);
System.out.println("Original number 1: " + number1);
System.out.println("Rounded number 1: " + roundedNumber1);
}
}
Output:
Original number 1: -15.5
Rounded number 1: -16.0
And the ceil() method rounds up:
public class MathRoundExample {
public static void main(String[] args) {
double number1 = -15.50;
double roundedNumber1 = Math.ceil(number1);
System.out.println("Original number 1: " + number1);
System.out.println("Rounded number 1: " + roundedNumber1);
}
}
Output:
Original number 1: -15.5
Rounded number 1: -15.0
The Art of Number Rounding in Java
Precision is often the key to accurate calculations and dependable software. Rounding plays a fundamental role in achieving greater precision, ensuring that numbers align with real-world expectations and mathematical integrity. Throughout this exploration, we’ve covered several different ways to round numbers using methods provided by the java.lang.math class, each serving a specific purpose in rounding numbers to fit the demands of various applications. This allows us to choose the best rounding mode for the application.
We began with Math.round(), a tool that rounds numbers to the nearest integer, using “round half up” rules. We then looked at Math.floor(), which elegantly truncates the decimal part, rounding down to the nearest integer. The Math.ceil() method does the opposite, rounding a number up to the nearest integer, a valuable trait when setting minimum values.
By harnessing the power of methods like `Math.round()`, `Math.floor()`, and `Math.ceil()`, you can ensure that your numbers align with real-world expectations and deliver results that are both dependable and precise.