Java Variables
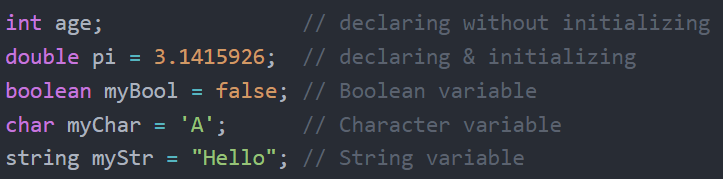
In Java, variables are used to store and manipulate data. Variables act as placeholders for various data types, and their specific data type determines the kind of values they can hold.
There are several types of variables in Java, including local variables, instance variables, static variables, and final variables.
Declaring a Variable in Java
In Java, you can declare a variable by specifying its data type and giving it a name. The basic syntax for declaring a variable in Java is as follows:
data_type variable_name;
Here’s a breakdown of the syntax:
- data_type: The type of data that the variable will hold. It can be one of the primitive data types (such as int, double, boolean, etc.) or it can be a reference data type (such as a class, interface, or array). You can also use custom data types that you define in your program.
- variable_name: The name you give to the variable. It must follow Java’s naming rules and conventions, such as starting with a letter, using letters, digits, or underscores, and not being a Java keyword.
Here are some examples of variable declarations:
int age; // Declaring an integer variable named 'age'
double salary; // Declaring a double variable named 'salary'
boolean isJavaFun; // Declaring a boolean variable named 'isJavaFun'
You can also initialize a variable at the time of declaration by providing an initial value:
int score = 100;
double pi = 3.1415926;
String greeting = "Hello, World!";
Initializing a variable means assigning an initial value to it. It’s not mandatory to initialize a variable at the time of declaration, but it’s a good practice to do so, especially for local variables, to avoid unexpected behavior.
Remember that variables in Java have a specific scope, which defines where in your code they can be accessed. Local variables have limited scope within the block or method in which they are declared, while instance and static variables have broader scopes within the class and its instances.
Variable Data Types in Java
Java provides a variety of data types that allow you to specify the type of data a variable can hold. These data types can be categorized into two main groups: primitive and reference data types.
Primitive Data Types
Primitive data types are the fundamental data types, and represent simple values. There are eight primitive data types in Java:
- boolean: A true or false value. The smallest possible data type, at just 1 bit.
- char: A 16-bit Unicode character.
- byte: A signed 8-bit integer value. Range: -128 to 127.
- short: A signed 16-bit integer value. Range: -32,768 to 32,767.
- int: A signed 32-bit integer value. Range: -2^31 to (2^31 – 1).
- long: A signed 64-bit integer value. Range: -2^63 to (2^63 – 1).
- float: A 32-bit floating-point value.
- double: A 64-bit floating-point value (default for decimals).
Here are examples of declaring variables with primitive data types:
boolean isJavaFun = true;
char myChar = 'A';
byte myByte = 10;
short myShort = 100;
int myInt = 1000;
long myLong = 100000L; // Note the 'L' suffix for long
float myFloat = 3.14f; // Note the 'f' suffix for float
double myDouble = 3.14159265359;
Reference Data Types
Reference data types refer to objects or complex data structures in Java. They do not store the actual data but instead store references (memory addresses) to the data. Common reference data types include:
- Strings: Used for text storage.
- Classes: User-defined data types created using classes.
- Arrays: Collections of elements of the same data type.
- Interfaces: Used for defining abstract methods and implementing multiple inheritance.
Here’s an example of declaring a reference variable:
String myString = "Hello, World!"; // String
int[] numbers = {1, 2, 3, 4, 5}; // Array of integers
These are the primary data types in Java, and they allow you to work with various types of data, from simple numbers to complex objects and data structures.
You can also create your own custom reference data types by defining classes or interfaces according to your own needs.
In addition to having different data types, there are also different categories of variables in Java, including: local, instance, static, and final variables. We’ll cover these next.
Types of Variables in Java
In this section, we’ll cover the different variable types in Java and see their unique properties.
Local Variables
Local variables are declared within a method or a block of code and have limited scope, typically existing only inside the block in which they are declared. They must be initialized before use.
Example of a local variable:
public void exampleMethod() {
int localVar = 10; // Declaration and initialization of a local variable
System.out.println(localVar);
}
Instance Variables (Non-static Variables)
Instance variables are declared within a class but outside of any method, constructor, or block.
They belong to instances of the class, so each instance has its own copy of these variables.
Instance variables are initialized with default values if not explicitly initialized.
Example of an instance variable:
public class MyClass {
int instanceVar; // Declaration of an instance variable
public void setInstanceVar(int value) {
instanceVar = value; // Initialization of the instance variable
}
}
Static Variables (Class Variables):
Static variables are declared with the static
keyword within a class but outside of any method. Unlike instance variables, they belong to the class itself, rather than instances of the class. They are shared among all instances of the class.
Static variables are initialized with default values if not explicitly initialized.
Example of a static variable:
public class MyClass {
static int staticVar; // Declaration of a static variable
public static void main(String[] args) {
staticVar = 5; // Initialization of the static variable
System.out.println(staticVar);
}
}
Final Variables (Constants):
Final variables are declared using the final
keyword and cannot be modified once they are assigned a value. They are typically used to define constants.
Example of a final variable:
public class Constants {
final double PI = 3.14159; // Declaration and initialization of a final variable
public static void main(String[] args) {
Constants constants = new Constants();
// constants.PI = 3.14; // This would result in a compilation error because PI is final
System.out.println(constants.PI);
}
}
Method Parameters:
Method parameters are variables that receive values when a method is called. They act as local variables within the method, and are used to pass data into the method.
Example of a method parameter:
public void add(int num1, int num2) { // num1 and num2 are method parameters
int sum = num1 + num2; // Local variable within the method
System.out.println("Sum: " + sum);
}
These are the main types of variables in Java, each serving a specific purpose.