How to Check if String Contains Substring in Python
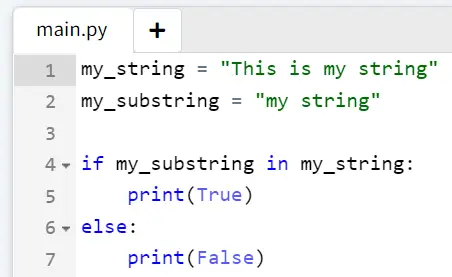
Strings are an essential part of Python, and there are many helpful functions and methods that allow us to efficiently work with them. One common task is checking if a given string contains a specific substring.
There are many situations when we may want to check if a string contains a substring, and there are various methods of accomplishing this task.
Here are a few use cases:
- Find out if a string contains a specific substring or word
- Get the location of a substring in a string
- Find matches for a substring regardless of character case
This article covers each of these cases and provides different ways of doing them.
It begins with a brief review of strings and how to use them in python programming. We then address substrings and give you different methods with which you can check if a string contains substrings using Python.
Hang on as we delve into the world of strings and substrings in Python!
Review of Strings in Python
Let’s review some important concepts related to strings and string methods before we cover how to check if a string contains a substring.
Strings are one of the basic data structures and are part of the basis for data manipulation. Strings are immutable (cannot be changed after creation) sequences of Unicode and are an essential part of programming.
Creating strings
You can create a string in Python by inserting characters inside a single quote or double quotes. Triple quotes are for creating multiline strings and docstrings (see sample below).
Assigning a string to a variable:
text = ‘Hello there!’
OR
text = “Hello there!’
Assigning multiline strings to a variable:
message = ”’Hello there!
Life is so much better when you see the bigger picture.”’
Accessing Characters in a String
You can access characters in a Python string by using the indexing method. Upon creation, each character in a string is automatically assigned an index number starting from zero (see sample code below).
Accessing string characters:
text = “Hello, world”
print(text[0])
print(text[5])
print(text[11])
Output:
H
,
d
The index method also works in a reverse manner. Using negative values of the index will have the program accessing the characters from the end of the string instead of the beginning shown above. Negative indexing begins with a minus one.
The following examples show how to access characters through negative indexing:
text = “Strings in Python”
print(text[-1])
print(text[-6])
Output:
n
P
Getting String Length
Rather than counting each character in a string to get its length, Python includes a function that lets you get the string length automatically.
The len() function returns the number of characters in a specific variable. The sample code below shows this usage.
Getting the length of the string stored in the variable ‘message’:
message = “The irony of life”
print(“The string length is; “+len(message))
Output:
The string length is 17
Create a Substring by Slicing
String slicing is a form of indexing syntax which draw out substrings from a larger string.
Like standard indexing, string slicing is zero-based. It involves using a colon; the index before the colon is the beginning of the slice, and the index after the colon is the end.
However, it is the character of the index immediately before the second argument that is returned and not the character of the end index itself. See the example code below:
Slicing a string into two:
name = “John Maze”
print(name[2:6])
Output:
hn Ma
Omitting the first index causes the slice to start from the beginning of the string. For instance:
Slice a string without specifying the first index:
message = “Clouds are beautiful”
print([message[:5])
Output:
Clouds
Likewise, omitting the last index will cause the slice to start from the first specified index to the end of the string.
Slice a string without specifying the last index:
message = “I love coding”print(message[2:])
message = “I love coding”print(message[2:])
Output:
love coding
Omitting both indexes will return the original string as a reference.
Slicing without any specified index:
message = “Python is a high-level programming language”
print(message[:])
Output:
Python is a high-level programming language
However, if the first index is greater than the second index, the python program will return an empty string.
Slice a string with the first index higher than the second:
message = “Keep the law”
print(message[5:3])
Output:
‘ ‘
Slicing also works well with negative indexing. Like standard indexing on python, the index value of -1 corresponds to the last character of a string.
Slice a string using negative indexing:
sample_word = “Chocolate”
print (sample_word[-4:-1])
print(sample_word[-4:]
print(sample_word[:-4])
Output:
lat
late
Choco
You can regulate the slicing of a string by assigning a step size. The step size indicates how many characters the program should jump after retrieving each character in a slice. It is great for presenting sliced data in a sequential format.
Slicing with a step size specified:
message = “Python is great”
print(message[0:15:3])
Output:
Ph e
The first and second indices can be omitted:
message = “Python is great”
print(message[::2])
Output:
Pto sget
Negative step values give the sequence in reverse order from the end of the string to the beginning:
message = “Python is great”
print(message[15:0:-5])
Output:
tst
Check if a String Contains a Substring in Python
Substrings in Python are sequences of characters within another string. In other words, a substring is just a subset of another string.
A common task is checking to see if a given string contains a given substring. There are several different ways to accomplish this. In the following section, we will see several methods for checking if a string has a substring in Python.
Ensure the String Contains Substrings Before Checking For a Specific Substring
Not all strings contain substrings. A typical example is this string below:
x = “7”
Before you can slice a string, it must have substrings. The absence of substrings in a string will make the program return an error when running an order to slice.
The string.find(substring) Method
The first method that we will cover is string.find(substring).
This technique uses the built-in Python find() method and is often considered the best way to check for the existence of a substring. It is always advantageous to take advantage of built-in functions and methods to accomplish a task cleanly.
The string.find(substring) method returns -1 if it does not find a match for the string in question.
If it finds the substring, it returns its starting index in the string (i.e. the left-most index of the substring). This method is very convenient for handling situations which need direct results without considering exceptions.
The string.find(substring) method can be used to find the index of a substring, or it can be used within a conditional statement to determine whether or not the substring can be found in the string.
Using string.find(substring) to get the index of a specific word or sub string in a string:
the_string = “My program works”
print(the_string.find(‘program’))
Output:
3
Note that in order to use the substring ‘program’ directly, we needed to put it in single quotes.
Instead of inputting the substring value itself inside the code, you can use the variable name directly:
the_string = “My program works”
the_substring = “program”
print(the_string.find(the_substring))
Output:
3
A common modification is combining the find() method with conditional statements. In the following code, we use an if/else statement to check for an error and to tell us if the string is present rather than returning the index:
the_string = “I have a computer”
substring = “compute”
if the_string.find(substring) != -1:
print(“Present”)
else:
print(“Absent”)
Output:
Positive
The ‘in’ Membership Operator
The easiest method in Python you can use to check substrings in strings is via the use of the ‘in’ membership operator. It checks the data structure for membership and returns a Boolean value (true or false).
To use it in checking if a string contains a specific substring, we can simply invoke it in a conditional statement. The following example shows how to use ‘in’ within an if/else statement as follows:
if substring in string
See the following example:
the_string = “This is a string”
substring = “is”
if substring in the_string:
print(“Present”)
else:
print(“Absent”)
Output:
Present
The ‘in’ membership operator has an exception when checking substring in a string. The exception happens when the string points to “None”. The None type is non-iterable and not able to contain a substring.
It is best to check whether the string points to None at once in the conditional statement to avoid having the exception.
the_string = None
substring = “is”
if the_string != None and substring in the_string:
print(“Present!”)
else:
print(“Absent!”)
Output:
Absent!
In the above code, we were able to prevent an exception error from being thrown using the conditional if/else statement.
Defeat Case Sensitivity With lower() Method
Each of these methods for checking if a string contains a substring in Python are case sensitive. As a result, every character of the substring that we check for must have a matching case within the string if it is to be identified.
This is useful if we want to check for an exact match, but can be frustrating if we don’t know the case of every character in the substring or if we want to more generally match the substring regardless of case.
Many times we want to use case insensitive matching.
For example, a user inputs a string with random case, assigning it to ‘variable’:
variable = “I go To scHool”
Checking for substrings in this string will be difficult because of case sensitivity. If we don’t capitalize the T and H letters in the string then Python won’t be able to find a substring.
However, you can easily make the ‘in’ membership operator case insensitive by including the lower() method. It is a simple but efficient addition to the conditional (if) statement.
The format is as follows:
if substring in string.lower()
This tells Python to first convert the string to all lowercase characters. In order for this to work, the substring that we search for must also be in lowercase.
See the below example:
the_string = “I gO to SchoOl”
substring = “school”
if substring in the_string.lower():
print(“Present”)
else:
print(“Absent”)
Output:
Present
The string.index() Method
The string.index() method is great for finding the position of the substring and not just if the substring exists. It does this by finding the starting index of the first occurrence of a substring in the string.
String.index() gives the starting index of the first occurrence of the specified substring:
the_string = “I am a Python programmer”
text = the_string.index(“programmer”)
print(text)
Output:
14
If the value of the substring is absent in string, the string.index() function raises an exception.
The string.find(substring) Method vs. string.index() Method
The string.index() method and the string.find(substring) are similar to each other. They both return the starting index of the substring of a string (if the substring exists in the string).
The major difference between the two is that unlike the string.index() method, the string.find(substring) method will return -1 when the substring is absent rather than an error message (see example below).
The following example shows the result of using the string.find(substring) method when the substring is not found:
the_string = “Exercise and food”
print(the_substring.find(“w”))
Output:
-1
Use Regular Expressions
Regular expressions (RegEx) allow us to check strings for substring matches using a flexible but complex process.
Regular expression is an inbuilt Python module known as the ‘re’. The re module has a search() function you can use to check for the presence of a substring in a string.
To use this method, you first import the module into your code before invoking the search() function in a conditional statement (see sample code below).
Check for a substring in the string using the regular expression (RegEx) method:
from re import search
the_string = “Maintenance”
substring = “ten”
if search(substring, the_string):
print(“Present”)
else:
print(“Absent”)
Output:
Present
The RegEx method is most useful when dealing with complex functions and strings with mixed cases. However, it is better to avoid using it when the conditions you are working with are simple because RegEx is quite complex and slow.
Conclusion – How to Check if String Contains Substring in Python
Checking to see if a string contains a substring is a common task in Python. We may we just want to see if there is a match for the substring, or find the location of a substring.
The easiest way to check for substrings is by using the ‘in’ membership operator (as shown above) which still does a better job when paired with the lower() function to ensure that case sensitivity does not affect your results. In contrast, the hardest way is via the Regular Expression method where you have to import the ‘re’ module and use the search() function in it to check if your substring is in a string. Of course, you should consider taking the easy way first before taking the hard way only if your string is a complex one.