How to Get the Last Character of a String in Python
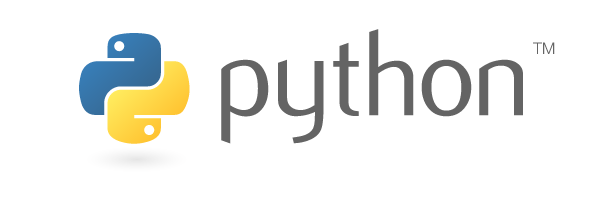
In the Python programming language, a character is a letter, number, or punctuation mark. In an ordered sequence, a group of characters become a string. Strings are excellent for helping a user communicate with a program.
One common task, and a great example for learning string manipulation, is how to get the last character of a string in Python.
Python Strings – Indexing and Slice Notation
Like most high-level programming languages, strings in Python are an array of letters. As such, they are subject to operations performed on arrays.
A Python string is always enclosed in either single or double quotation marks and can be assigned to a variable. In the first example below, we can see that strings can be created using single or double quotes:
# Writing a string in python with single quote or double quotes
print(‘Hello there’)
print(“Hello there”)
You can carry out many operations on strings using Python. These operations range from concatenation, extraction of partial strings, and adding and removing spaces to formatting using string formatting functions.
In this article, we will see three different ways for how to get the last character of a string. We also include how to get the last n characters and the last word of a string in python. Along the way, we will learn some powerful methods of string manipulation. Let’s begin with a review of string indexing.
String Indexing in Python
Indexing is one of the most useful tools programmers have. Indexing in Python means that each character in a string is indexed with a number. The string index uses square brackets and starts at zero [0]. The first character of the string is indexed [0], the second item is [1] and so forth.
As you can imagine, indexing is very useful. For string operations, indexing allows you to access individual characters of a given string directly via a numeric value.
In Python, indexing is zero-based. As a consequence, the first character of a string bears an index of 0; the next character has an index of 1, and so on.
How to Access Different Characters of a String in Python
Indexing comes to play when trying to access different characters of a string. Since indexing in Python is zero-based, you have to keep in mind that the very first character in a string has an index of zero. Other characters follow suit. Take the following example with a sample string assigned to the variable ‘text’:
#string assigned to the variable ‘text’
text = ‘Learning Python’
print(text[0])
Output:
L
print(text[3])
Output:
r
print(text[7])
Output:
g
In the above example, ‘L’ has an index of zero, ‘r’ an index of three and ‘g’ has an index of seven.
String Slicing
You can also specify a range of characters to slice using a colon ‘ : ‘ within the square brackets. This is also called slice notation.
string[start:stop]
Where the first index, ‘start’ indicates the starting index of the slice and ‘stop’ the ending index.
text = ‘Learning Python’
print(text[0:5])
Output:
Learn
In the above code, the start index was set to 0, the first character of the string.
When specifying a range, Python will get the characters up to but not including the character with the last index of the specified range. In other words, the character with an index of five in the previous example was the ‘i’:
With slice notation, we can get the first n characters of a string using a colon with no number in front of it:
text = ‘Learning Python’
print(text[:8])
Output:
Learning
We can also grab the last few characters of a string using slice notation. In the following code,
text = ‘Learning Python’
print(text[9:])
Output:
Python
We can tell Python to get everything after and including the ninth character by using the colon without a number after it, e.g. text[9:]
How to Get the Last Character of a String in Python
Imagine you have a long string and you want to get the last character. It would not be practical to start counting how many letters are there for you to get a good guess of the number the index falls into. Moreover, you may not even be able to see the string. We have to have ways of accessing the last character without being able to see the string itself.
You might think to check the length of the string (see sample code below) first, to use that as a means of getting the index of the last character. You can do this using the len() function, which we will see in greater detail below.
# create new string named ‘lorem_ipsum’
lorem_ipsum = ‘Lorem ipsum dolor sit amet’
#print length of string using len()
print(len(lorem_ipsum))
Output:
26
#Get the last character
print(lorem_ipsum[25]
Output:
t
We can optimize this further but Python actually gives us multiple ways to perform the same task more easily.
There are two simple methods you can use to get the last character of a string in python without breaking a sweat. The first, and easiest, is by using negative indexing. The next is using an optimized version of the len() code we saw above. Finally, we can use the endswith() function, which has some unique functionality.
The Negative Indexing Method
We have seen that string indexing can be powerful in Python. So far we have used positive string indexes. What if we use negative numbers to index characters in a string?
The negative indexing method is the easiest way to get the last character of a string in a python program. Positive index numbers start their counting from the beginning of the string while negative index numbers start from the end of the string.
While index numbers of 0 and 1 give the first two characters of the string, the negative index numbers of -1, -2 will give the last and the second to the last character respectively. This allows us to use negative indexes to reference characters at the end of a string.
# Sample code:
lorem_ipsum = ‘Lorem ipsum dolor sit amet’
print(lorem_ipsum[0])
Output:
L
print(lorem_ipsum[-1])
Output:
t
print(lorem_ipsum[-2])
Output:
e
print(small_text[-4:])
Output:
amet
The advantage of this method lies in its easy use, and reduced code length when compared with other methods. It is the least complex and is easy to remember.
Using the len() function
Another easy way to get the last character of a string is via using the len() function. The len() function returns the length of a string; an empty string will return a length of zero.
The first step in this method is to assign the length of a string to a variable. Then, another variable is used to store the last character of the string for display (check sample code below).
length = len(text) #length of string is assigned to the variable – length
last_char = text[length-1] #last character is stored in the variable – last_c
print(‘The last character in this string is: ‘, last_char)
In this case we are using the length of the string directly in the index using the variable ‘length’. Then we assign the last character to the variable ‘last_char’.
Using the endswith() function
The endswith() function allows us to check if a string ends in a specific character. Unlike the prior examples, it returns a Boolean value of ‘true’ or ‘false’.
Imagine you wish to continue from where you stopped while inputting a very long piece of string. You might have it committed to memory but not entirely sure. The endswith() fiction will save you the stress of having to employ methods requiring you to see the last character.
The method involving the endswith() function is quite different from the other methods above. It involves the use of a data type called the Boolean type. What this data type does is to return true or false depending on the condition given. Hence, in this case, you can use the endswith() function to find out if the last character or string ends with a particular character or string you believe it should, or not. An example of this method is given below:
text = “Python is the best programming language”
print(text.endswith(‘language’)) #prints true since the string ‘text’ ends with ‘language’
The use of this method is most suitable for validating purposes. As such, you will have to revert to other means if your interest lies not in knowing if the end is what it is supposed to be.
How to Get the Last N Characters of a String
So far, we have looked at how to get one character out of all others in a string. What if you need to get more than just the last character? What if you need to print the last ‘n’ number of characters in succession? In this case, we want to carve a new string out of an existing one.
The easiest method you can apply for this task is by combining negative indexing with slice notation. Recall that we could use a colon to designate slice notation if we want to obtain a section of a string.
In this case, we combine negative indexing with a colon in order to get the last n characters of the string.
# Code that prints the last n characters of a string
print(message[n:]) #prints last n characters where n is an index number.
Example:
message = “Jimmy Carter is an old man.”
print(message[-8:])
#prints ‘old man.’
#Note: The space between words also counts as a character.
How to Get the Last Word of a String in Python
Many times, the task may not be to get the last character, but the last word in the whole string. In this case, it is best to use a slightly more advanced method: the split() function.
To get the last word of a string, you can use the split() function, which will separate the original string into a group of smaller strings.
The split() function is simple and easy to use. As the name suggests, it splits the original string into parts. You can print any of those parts or use them for further manipulations. Here is a format you can follow:
string = “Run down home”
first, middle, last = string.split()
print(last) #prints last word – ‘home’
Output:
home
print(first) #prints first word – ‘Run’
Output:
Run
print(middle) #prints middle word – ‘down’
Output:
down
You can print the string in a rearranged manner by manipulating the split parts.
print(first, last, last) #prints ‘Run home home’
If you do not wish to define the splitting of the string, you can use the format below. What happens in the code is that the string gets split by the .split() function with the [-1] returning the last word from the split.
string = “Run down home”
last_word = string.split()[-1])
print(last_word) #prints the last word – home
The last sample code is very convenient and most preferred over the other for obtaining just the last word.
An example of a task this function is widely used for is the arrangement of surnames and first names in forms and records. The code below illustrates this.
# Program prints the last and first name in that order
name = “Chase Henry Martin”
first_name, *middle_name, last_name = name.split()
print(last_name, first_name)
Summary
Getting the last character of a string in Python can be done in several ways. It comes in handy, especially in operations involving the sorting of data. You can choose between the negative indexing method or use the len() function to get the last character. A slight modification to the negative indexing method using slice notation gives you a set of last characters (last n characters) and you can get the last word of a string by using the .split() function.