How to Remove an Element From a List in Python
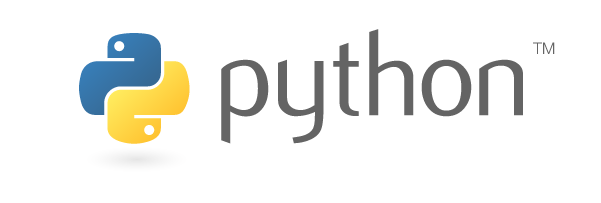
Lists are one of the most useful data types in Python because they can store multiple items within a single variable. Python gives us many different ways of working with lists. As a result, there are several easy ways to remove an element from a list in Python.
The most common ways to remove an element from a list in Python are: del, pop(), slice(), clear(), remove(), and list comprehensions.
These may seem similar, but they operate in different ways that allow developers to solve different types of problems. For example, the del operator can be used to delete elements from a list. If we want to remove and store a list element in another variable, we can use pop(). If we want to remove and store multiple list elements, pop() won’t work. Instead, we’ll need to use something like slice().
In this article, we’ll cover the following operators, functions, and methods that give us different ways to remove an element from a list in Python:
- The del operator
- The pop() method
- The slice() function
- The clear() function
- The remove() method
- List comprehensions
What it Does | When to Use | |
del | Deletes one or more list elements | Return value not needed |
pop() | Removes and returns a single list element | You need to remove and return a single list item |
slice() | Removes and returns one or more list elements | You need to remove and return multiple list items |
remove() | Removes and returns elements of a specific value | You know the value, not the index |
clear() | Deletes all items from list | You need to empty a list |
In addition to seeing example code for each list method above, we will also cover the advantages, disadvantages, and ideal applications of each to help you select the right method for your application.
Introduction to Removing an Item from a List in Python
Part of what makes lists useful is the fact that they are mutable, meaning that they can be changed after they are created.
At the most basic level, mutability in lists depends on being able to add or remove elements from a list. For example, we may want to remove or return a single list element or multiple elements. These items may be at a given index location, or you may know the value of the item but not the location.
Luckily there are different ways of performing these basic actions – and they differ in function as well as syntax. In this article we’ll cover several of the most popular methods of how to remove an element from a list in Python.
Review of Lists in Python
In the Python programming language, lists are mutable data types that can store multiple values. The list data type is one of four built-in data types designed for storing collections of data.
Mutability is an important concept in Python programming. A mutable object can be changed after it is created, and lists are an important category of mutable objects.
As a review, we can create a list variable by placing elements inside square brackets with commas separating them.
Creating a new list in Python:
counting_tens = [10, 20, “Thirty”, 40, 50, “Sixty”, “Seventy”]
print(counting_tens)
Output:
[10, 20, ‘Thirty’, 40, 50, ‘Sixty’, ‘Seventy’]
Now that we have a list, let’s see various methods for removing list elements.
Remove List Elements Using del
In Python, del is a keyword that is used to delete objects. It can be used to delete an entire object or part of an object, like an item in a list.
The del operator removes an element by taking its index as the argument. You can use it to remove a range of items at once or just a single item from a given list. The key to using the del operator to remove items is to ensure your range specification is accurate. The data will be deleted and will not be stored in a different variable, so it’s important to ensure we only delete what we want to.
Using del to Delete a Single List Item
In the following example we want to delete the third item of the list, “Third”. The first element in a list has an index of ‘0’, so to access the third element we will need to use an index of ‘2’:
my_items = [“First”, “2nd”, “Third”, 4, 5, 6, “End”]
del my_items[2]
print(my_items)
Output:
[“First”, “2nd”, 4, 5, 6, “End”]
This syntax can be used anytime we need to delete a specific element at a given index position.
Note that since the item has been removed from the list, all of the items after it now have their index value decremented. Before using the del keyword, the fourth item in the list was ‘4’. After deleting the third item, the fourth item in the list is now ‘5’.
Using del to Delete Multiple List Items
We can also use the del keyword to remove multiple items from a list:
my_items = [“First”, “2nd”, 3, 4, 5, “Finish”]
del my_items[2:4]
print(my_items)
Output:
[‘First’, ‘2nd’, ‘Finish’]
In the del statement above, we used the colon syntax to specify a range of elements to be deleted.
The del operator will return a ValueError if you use an index that does not exist for that list.
The following code shows the outcome of using a non-existent index with the del operator:
my_items = [“First”, “2nd”, “Finish”]
del my_items[4]
Output:
ValueError
The primary disadvantage of the del method is that it does not return the value of the element removed from the list. If we want the value to be returned so that it can be used or stored, we can use the pop() method.
Using pop to Remove an Element From a List
The pop() method is used to remove and return a single item from a list. It is widely used due to its simplicity. Like the del operator, the pop method involves specifying the index of the element.
While del deletes the element in question, pop() returns it and can store the deleted element in another variable. In other words, the pop() method produces a return value equal to the list element that we specify.
Additionally, pop() only takes one argument and can therefore only be used on a single element.
Using pop() to Remove and Return a Single List Item
school_things = [“backpack”, “pencil”, “pen”, “calculator”, “computer”]
shopping_list = school_things.pop(3)
print(school_things)
print(shopping_list)
Output:
[‘backpack’, ‘pencil’, ‘pen’, ‘computer’]
calculator
In the above example, we use pop() to remove item at index ‘3’, which has the value ‘calculator’. The removed element was stored in the variable shopping_list.
Using pop() to Remove and Return the Last Item of a List
When pop() is used without an argument, it removes and returns the last item of the list.
school_things = [“backpack”, “pencil”, “pen”, “calculator”, “computer”]
shopping_list = school_things.pop()
print(school_things)
print(shopping_list)
Output:
[‘backpack’, ‘pencil’, ‘pen’, ‘calculator’]
computer
An incorrect index will also return a ValueError message, like the del operator.
Using Slice() to Return Elements From a List
Slice() is a built-in function that allows us to obtain a part, or slice, of a list. We can use slice() to get the beginning, end, or middle of a list. Note that when using slice(), the original list will remain intact.
The slice function is different from regular slicing in Python. It does not make use of indexing. Instead, the number called in the function is the exact number of the order the element appears.
Calling a positive number in the slice() function makes it recall items from the beginning of the list to the specified position, omitting the others. Using a negative number in the function makes it remove items from the last element in the list to the one in the position of the specified number.
In both cases, the slice will start at the beginning of the list and go until the item at the specified index.
Slice a list using a positive index
the_whole = [“James”, 33, 71, “Eddie”, 44, “Smith”, 76, 59, 65, “Dawn”]
new = (the_whole[slice(4)])
print(new)
Output:
[‘James’, 33, 71, “Eddie”]
Slice list using negative index values
new = slice(-4)
print(the_whole[new])
Output:
[‘James’, 33, 71, “Eddie”, 44]
We can specify the start of the slice by adding a second argument. When using two arguments with the slice() function, we can remove a slice from the middle of a list or even remove and store a single list item.
Using slice() with two arguments to remove a single element
the_whole = [“James”, 33, 71, “Eddie”, 44, “Smith”, 76, 59, 65, “Dawn”]
new = (the_whole[slice(3,4)])
print(new)
Output:
[‘Eddie’]
del vs. pop vs. slice
We have seen three built-in methods for how to remove an element from a list in Python: del, pop, and slice.
The first method uses del to delete a single or multiple elements from a list. It is ideal when you don’t want to return an item or items; you only want to delete them/remove them from the list.
The pop() method removes an item from a list and returns its value, but it can only handle a single item. Therefore pop() is best when you only want to get a single item and return its’ value. This value can be stored in another variable, printed, or used in other ways.
Finally, slice is a function that allows us to return any number of list items. It does not alter the original list. The slice function is best when you want to return more than one list item at a time and you don’t want to remove the items from the original list.
You can use the del operator to remove several items at once from a list, but you need to know their exact indexes to get the correct result. It is easy to get the index of elements on a small list but lists with plenty of items or other lists within it make using the del operator to remove multiple items difficult.
In subsequent paragraphs, we show you how to remove all or multiple elements from different positions of a list at once without the necessary knowledge of their index numbers.
Removing All Elements of a List with Clear
The clear function presents an easy way to empty a list rather than manipulating the slice function or any other function. Lists, whether bulky or small, get cleared instantly by the clear function and returns nothing. See sample code below:
Using the clear function to remove all elements of a list at once:
my_list = [11, 22, 33, 44, 55, 66, 77, 88, 99, 110, 121, 132]
new_list = my_list.clear()
print(new_list)
Output:
None
In the above example, we can see that the print function returns an empty list.
Remove an Item by Value with remove()
The remove method is beneficial when you know the exact content of the item you wish to remove. It is case and order sensitive.
Removing an Item From a List With remove()
To use remove(), we simply specify the value of the item that we want to be removed:
six_items = [“one-apple”, “two-androids”, “three-macs”, “four-dells”, “five-macintosh”, “six-msis”, “seven-dolls”]
six_items.remove(“seven-dolls”)
print(six_items)
Output:
[‘one-apple’, ‘two-androids’, ‘three-macs’, ‘four-dells’, ‘five-macintosh’, ‘six-msis’]
This will search the list for an item with the given value and remove it from the list.
What if there are multiple items with the same value?
When two or more items are the same, remove() removes the first occurrence of the element. The disadvantage of this method presents itself when the removal of similar items from different positions other than the first is needed.
Removing the first repeat item using the remove function:
my_list = [9, 18, 81, 36, 45, 54, 81, 72, 81]
my_list.remove(81)
print(my_list)
Output:
[9, 18, 36, 45, 54, 81, 72, 81]
Therefore, if you have identical items in an list and you wish to remove all entries, you will need another method.
Remove Multiple List Items Using remove() With for Loop
One way to accomplish this is by using a for loop. In this case, we will combine the for loop with the remove() function to remove multiple entries.
Removing an item from the list using the for loop and the remove() function:
the_list = [1, 2, 3, 4, 5, 6]
for item in the_list.copy():
if item < 2:
the_list.remove(item)
break
print(the_list)
Output:
[2, 3, 4, 5, 6]
Using list comprehensions to remove items from a list
List comprehension in Python consists of brackets containing an expression that the program executes for each element in the list. The for loop in a list comprehension expression iterates over each item in the list. As a result, instead of individually picking off items that occur in very random sequences in a list, you can use list comprehension to remove all of them at once with sharp accuracy.
List comprehension reduces the effort you would use with writing loop conditions singly and does not only save time and space on a code but enables you to get more accurate iterations, especially on big data.
The general syntax for list comprehension is:
new = [(expression) for (item) in (old_list) if condition]
You can use list comprehension for several data manipulation like creating matrices.
Using list comprehension to create a matrix:
whole_list = [4, 1, 7]
squared_list = [(i, i**2) for i in whole_list]
print(squared_list)
Output:
[(4, 16), (1, 1), (7, 49)]
To utilize list comprehensions for the removal of items in a list, your code should:
- Iterate over the list (using the format of the list comprehension syntax given above)
- Have the proper condition that filters out the items you do not want in your result.
Removal of multiple items over a range using list comprehension:
the_list = [23, 33, 43, 53, 63, 73, 83, 93, 103]
new_list = [item for item in the_list if item <= 60]
print(new_list)
Output:
[23, 33, 43, 53]
List comprehension is most useful when you know how to construct the proper condition.
Summary
In this Python tutorial, we have seen multiple different methods, functions, and operators for removing and returning elements of a list in Python.
These include: del, pop(), slice(), clear(), remove(), and list comprehensions.
Each method has its’ own specific functions, which is why it is important to choose the right method for the application. We saw examples for each as well as discussing the advantages, disadvantages, and ideal applications of each method.