Bash Input
In Bash, input refers to data that a script gets externally, from the user, from another program, or from a file.
Input is important because it allows scripts to be dynamic and interactive.
There are several common kinds of input that we can expect to frequently encounter, including command-line arguments, user prompts, stdin (standard input), and environment variables.
In this article, we’ll learn the ins and outs of working with input in Bash scripts.
Command-Line Arguments
Command-line arguments are one of the most common ways of interacting with user input. Bash makes it easy by storing the arguments in numbered variables called special variables.
The variable $1 represents the first argument passed to the script following the name of the script. For example, in the terminal:
./my_script.sh arg1 arg2
The first part ‘my_script.sh’ is the name of the script; it can be accessed using the $0 special variable. Next is ‘arg1’, the first argument. It can be called using $1. Similarly, arg2 can be called using $2.
In Bash scripts, command-line arguments are accessible through special variables. Here’s how you can work with them:
#!/bin/bash
echo "Script Name: $0"
echo "First Argument: $1"
echo "Second Argument: $2"
./script.sh arg1 arg2
Output:
Script Name: ./script.sh
First Argument: arg1
Second Argument: arg2
Accessing All Arguments
We can access all command-line arguments using $@
or $*
. For example:
#!/bin/bash
echo "All Arguments: $@"
If we run this and provide ‘arg1‘, ‘arg2‘, and ‘arg3‘ as arguments, we can see that the script returns them all:
./script.sh arg1 arg2 arg3
Output:
All Arguments: arg1 arg2 arg3
We can use a loop to process a variable number of arguments.
For example:
#!/bin/bash
echo "Total Number of Arguments: $#"
for arg in "$@"; do
echo "Argument: $arg"
done
Running the Script:
./script.sh arg1 arg2 arg3
Output:
Total Number of Arguments: 3
Argument: arg1
Argument: arg2
Argument: arg3
Total Number of Arguments: 3
Argument: arg1
Argument: arg2
Argument: arg3
These examples demonstrate how to work with command-line arguments in a Bash script.
User Prompts in Bash
User prompts are messages that prompt the user to input data. They are useful for creating interactive scripts that require user input.
User prompts are typically used with the read
command, which reads input from the user and stores it in a variable. When used with the -p
option, it displays a prompt before reading input:
read -p "Enter your name: " name
Note that we used the string ‘name’ following the double quotes at the end of the prompt. This creates a new variable $name, which we can use to access the user input. Let’s see an example:
#!/bin/bash
read -p "Enter your name: " name
echo "Hello, $name!"
When you run the script, it will display the prompt and wait for you to enter your name. If you enter your name, it will be echoed back to you!
./script.sh
Enter your name: John
Hello, John!
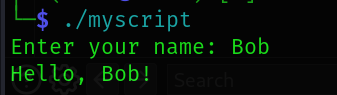
We can also use the -s flag, which will make the input ‘silent’, i.e. the input won’t show up on the screen as the user types it in. This can be useful when asking the user to enter sensitive information like a password:
#!/bin/bash
read -p "Enter your name: " name
read -p "Enter your password: " passwd

User prompts are a simple yet effective way to make Bash scripts interactive and allow them to receive input from users during execution.