Variables in Bash
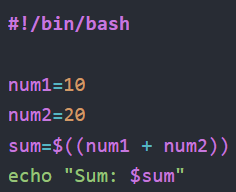
In Bash, variables are used to store data values for later use in the script. They can hold various types of data, such as numbers, strings, and arrays.
Variables are fundamental to Bash scripting and are used to control the flow of the script, store user input, and manipulate data.
In this article, we will dive deep into the topic of variables in Bash. We’ll see how variables function, learn about variable scope and see different types of variables, like special variables and read-only variables.
In Bash, variables are typically defined using the following syntax:
variable_name=value
For example:
name="John"
age=30
In order to access the value of a variable, we need to prefix the variable name with a dollar sign ($
):
echo "Name: $name"
echo "Age: $age"
By default, variables in Bash are treated as strings. However, we can perform arithmetic operations on variables containing integer values using the $((...))
syntax:
#!/bin/bash
num1=10
num2=20
sum=$((num1 + num2))
echo "Sum: $sum"
Output:
Sum: 30
Variables can also be used in loops, conditionals, and function definitions, making them a versatile tool for scripting in Bash.
Declaring Variables
In Bash, variables are declared by assigning a value to them. There are a few different ways to declare variables:
- Simple Assignment: Use the assignment (
=
) operator to assign a value to a variable. No spaces are allowed around the=
sign.
name="John"
age=30
- Using the
declare
Built-in: Thedeclare
built-in can be used to declare variables explicitly, with optional attributes such aslocal
,readonly
, ortypeset
.
declare -r readonly_var="Read-only"
declare -i integer_var=10 # Declare as an integer
declare -a array_var # Declare as an array
- Using
local
: Inside a function, you can use thelocal
keyword to declare variables that are local to the function.
function my_function {
local local_var="I am a local variable"
}
- Without Assignment: You can declare a variable without assigning a value, but this is less common. The variable will have an empty value.
empty_var=
After declaring a variable, you can use it throughout your script. Remember that variable names are case-sensitive in Bash.
Variable Scope in Bash
The scope of a variable refers to the parts of your script where the variable is recognized.
A global variable, as the name suggests, is one that can be accessed from anywhere within your script. Conversely, a local variable is confined and is only recognized within the function where it has been declared.
Now, let’s look at a related topic – the lifespan of variables in Bash. The lifespan is the duration for which it exists or is valid in your script.
A global variable in Bash, being accessible throughout the script, continues to exist until the execution of the script is complete. On the other hand, a local variable, bound by the borders of its function, ceases to exist when the function finishes execution.
Let’s take a look at these in greater detail.
Global Variables
Variables declared outside of any function or block have global scope. They can be accessed from anywhere in the script, including inside functions. Example:
global_var="Global variable"
function my_function {
echo "Inside function: $global_var"
}
echo "Outside function: $global_var"
my_function
Local Variables
Variables declared inside a function have local scope. They can only be accessed from within that function and are not visible outside of it.
The following example shows an error occurring when a local variable is accessed outside of its scope:
function my_function {
local local_var="Local variable"
echo "Inside function: $local_var"
}
my_function
# This will throw an error as $local_var is not accessible here:
echo "Outside function: $local_var"
Variable Shadowing in Bash
Variables with local scope can shadow variables with the same name in the global scope. In the example below, the my_function
function declares its own var
variable, which is different from the global var
variable:
var="Global var"
function my_function {
local var="Local var"
echo "Inside function: $var"
}
echo "Outside function: $var" # Outputs: Outside function: Global var
my_function # Outputs: Inside function: Local var
echo "Outside function: $var" # Outputs: Outside function: Global var
Understanding variable scope is important for writing scripts that behave as expected and for avoiding unintended side effects caused by variable naming conflicts.
Grasping these concepts is vital in managing the accessibility and lifecycle of your variables effectively. The judicious use of global and local variables can significantly enhance the efficiency of your Bash scripts. For instance, a variable that needs to be accessed and manipulated across your script can be declared as global. In contrast, a variable that is used only within a specific function can be kept local, promoting better memory management and preventing accidental alteration of its value elsewhere in the script.
A Deeper Look at Variables in Bash
Variables can be thought of as placeholders or aliases, standing in for the actual data they represent. Each variable has a unique name, and the data it holds is known as its value.
Variables in Bash are case sensitive. A variable named ‘MyVar’ is not the same as ‘myvar’. The differentiation of case allows for more diversity in variable names and can also be used strategically in our scripts.
In Bash, variable names can be composed of any combination of letters, numbers, and underscores, but they must not begin with a number. The assignment operator (=) is used to assign a value to a variable, and there must be no space on either side of the operator.
Bash also enables the use of environment variables. These are a set of dynamic named values that are part of the operating environment. While most of these are set by the system, some are set by the user or by the shell.
Special Variables in Bash
Special variables in Bash are predefined variables that hold information about the shell environment, the script itself, and the arguments passed to the script.
These variables are set by the shell and can be referenced by the user to access useful information. Some of the most common special variables in Bash are:
- $0: The filename of the current script. This variable is used to reference the script’s name within the script itself.
- $1, $2, $3, …: The positional parameters or arguments passed to the script.
$1
represents the first argument,$2
represents the second argument, and so on. These are used to store and access the values of command-line arguments. - $?: The exit status of the last command executed. It is typically
0
for success and nonzero for failure. This variable is commonly used for error checking and handling in scripts.
These special variables are frequently used in Bash scripts for various purposes, such as script initialization, processing command-line arguments, and error handling. However, there are other useful special variables as well, and are worth looking into.
Array Variables in Bash
In Bash, array variables are variables that can hold multiple values under a single variable name. Unlike ‘scalar variables’, which can hold only a single value, array variables can store a collection of values indexed by a numerical index.
Array variables are declared using parentheses, and elements are separated by spaces:
my_array=(value1 value2 value3)
To access an element of an array, you use the array name followed by the index enclosed in curly braces {}
. Indexing starts at 0 for the first element. For example, to access the first element of my_array
:
echo ${my_array[0]} # Outputs: value1
You can also assign values to individual elements of an array:
my_array[1]=new_value
To iterate over all elements of an array, you can use a loop:
for element in "${my_array[@]}"; do
echo "$element"
done
Arrays in Bash provide a convenient way to store and manipulate collections of values, making them a powerful tool in Bash scripting.
Using Variables in Bash Scripts
Variables can be used in various ways to enhance the functionality and flexibility of Bash scripts. Some important and advanced uses of variables include:
- Command Substitution: Assigning the output of a command to a variable.
current_date=$(date +%Y-%m-%d)
echo "Current date: $current_date"
- Arrays: Using arrays to store and manipulate multiple values.
fruits=("apple" "banana" "orange")
echo "First fruit: ${fruits[0]}"
- Indirect Reference: Using variable indirection to access variables dynamically.
var_name="my_var"
my_var="Hello, World!"
echo "${!var_name}" # Outputs: Hello, World!
- Variable Expansion: Using parameter expansion for string manipulation.
greeting="Hello, World!"
echo "${greeting:0:5}" # Outputs: Hello
- Variable Scoping: Using local variables in functions to limit their scope.
my_function() {
local var="Local variable"
echo "Inside function: $var"
}
my_function
echo "Outside function: $var" # This will throw an error as $var is not accessible here
- Variable Defaults: Using default values for variables that may not be set.
echo "${undefined_var:-default_value}"
- Arithmetic Operations: Performing arithmetic operations using the
((...))
construct.
num1=10
num2=20
sum=$((num1 + num2))
echo "Sum: $sum"
- Read-Only Variables: Marking variables as read-only to prevent them from being changed.
readonly var="Read-only"
- Exporting Variables: Exporting variables to make them available to child processes.
export MY_VAR="Value"
- Using Variables in
awk
andsed
: Passing Bash variables toawk
andsed
commands for text processing.bash awk -v var="$my_var" '{print var, $0}' file.txt
These advanced uses of variables in Bash scripts can help you write more powerful and flexible scripts, enabling you to handle complex tasks more effectively.