Operators in Bash Scripts
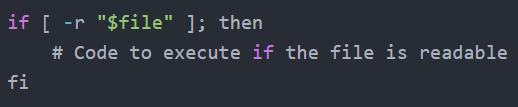
Operators are fundamental building blocks in Bash scripts. They enable us to perform a wide range of operations, from basic arithmetic calculations to complex string manipulations and file tests. Operators also provide a means to control the flow of your script and make decisions based on conditions.
In this article, we’ll explore the various types of operators in Bash scripting and discuss their unique features and functionalities. We’ll cover topics such as arithmetic operators for mathematical calculations, comparison operators for evaluating conditions, and logical operators for combining conditions.
One of the key aspects we’ll delve into is the set of operators that are unique to Bash as a language. These include features like pattern matching operators for string manipulation, file test operators for checking file attributes, and advanced assignment operators for variable manipulation.
Whether you’re new to Bash scripting or looking to expand your knowledge, this article will provide you with a comprehensive understanding of operators in Bash and how to leverage them effectively in your scripts.
Arithmetic Operators in Bash
Arithmetic operators in Bash are used to perform mathematical calculations on numerical values.
They allow us to add, subtract, multiply, divide, and perform other arithmetic operations. Here are the arithmetic operators available in Bash:
Addition
The addition (+) operator adds two numbers together.
result=$((num1 + num2))
Subtraction
The subtraction (-) operator subtracts one number from another.
result=$((num1 - num2))
The multiplication (*) operator multiplies two numbers.
result=$((num1 * num2))
The division (/) operator divides one number by another.
result=$((num1 / num2))
Modulus (%) computes the remainder of division.
result=$((num1 % num2))
The Increment (++) operator increases the value of a variable by 1.
num++
- The decrement (–) operator decreases the value of a variable by 1.
num--
These operators can be used in arithmetic expressions within double parentheses ((...))
or in arithmetic expansion $((...))
. They are essential for performing mathematical calculations in Bash scripts.
For more information on this topic, check out our tutorial on Math in Bash!
Comparison Operators in Bash
Comparison operators in Bash are used to compare values and evaluate conditions. They are commonly used in conditional statements (if
statements) to make decisions based on the result of the comparison. Here are the comparison operators available in Bash:
The equal to (==) operator checks if two values are equal. This is shown in the following if statement:
if [ "$var1" == "$var2" ]; then
# Code to execute if var1 is equal to var2
fi
The not Equal (!=) operator checks if two values are not equal.
if [ "$var1" != "$var2" ]; then
# Code to execute if var1 is not equal to var2
fi
Greater Than (>) checks if one value is greater than another.
if [ "$num1" -gt "$num2" ]; then
# Code to execute if num1 is greater than num2
fi
Greater Than or Equal To (>=) checks if one value is greater than or equal to another.
if [ "$num1" -ge "$num2" ]; then
# Code to execute if num1 is greater than or equal to num2
fi
Less Than (<) checks if one value is less than another.
if [ "$num1" -lt "$num2" ]; then
# Code to execute if num1 is less than num2
fi
Less Than or Equal To (<=) checks if one value is less than or equal to another.
if [ "$num1" -le "$num2" ]; then
# Code to execute if num1 is less than or equal to num2
fi
These comparison operators can be used in conditional statements to evaluate conditions and control the flow of your Bash scripts based on the result of the comparisons.
Assignment Operators in Bash
Assignment operators in Bash are used to assign values to variables. They provide a variety of ways to update the value of a variable based on its current value or the result of an operation.
The ‘simple’ assignment operator only performs one operation, which is assigning a value to a variable. The other assignment operators perform more than one operation. For example, the ‘addition assignment’ operator adds a value to the current value of a variable – performing two operations, addition and assignment.
Here are the assignment operators available in Bash:
The assignment/simple assignment (=) operator assigns a value to a variable.
var="value"
The addition Assignment (+=) operator appends a value to the end of a variable.
var+="value"
Subtraction Assignment (-=) removes a value from a variable.
var="Hello, World!"
var=${var/"Hello, "/}
The multiplication assignment (*=) operator multiplies the value of a variable by a specified value.
num=5
num=$((num * 2))
Division Assignment (/=) divides the value of a variable by a specified value.
num=10
num=$((num / 2))
Finally, the modulus assignment (%=) operator calculates the modulus of the value of a variable with a specified value.
num=5
num=$((num % 2))
These assignment operators can be used to modify the value of a variable in place, making it easier to perform operations and update variable values in Bash scripts.
String Operators in Bash
String operators in Bash are used to manipulate and compare strings. They allow us to check if a string is empty, compare two strings, extract or remove substrings, and more.
Here are the string operators available in Bash:
The string length operator ${#string}
returns the length of the string.
string="Hello, World!"
length=${#string}
Substring extraction ${string:position:length}
extracts a substring starting at the specified position with the specified length.
string="Hello, World!"
substring=${string:7:5} # Extracts "World"
The substring removal operator ${string#substring}
removes the shortest match of substring from the beginning of the string.
string="Hello, World!"
removed=${string#Hello, } # Removes "Hello, "
There are two types of substring replacement operators. Substring replacement ${string/pattern/replacement}
replaces the first match of pattern with replacement.
string="Hello, World!"
replaced=${string/World/John} # Replaces "World" with "John"
In contrast, the global substring replacement ${string//pattern/replacement}
replaces all matches of pattern with replacement.
string="Hello, World!"
replaced=${string//o/0} # Replaces all "o" with "0"
We can check if a string is empty with the -z
operator:
if [ -z "$string" ]; then
# Code to execute if string is empty
fi
Alternately, we can check if a string is not empty with the -n
operator:
if [ -n "$string" ]; then
# Code to execute if string is not empty
fi
The comparison operators we saw above can also be used on strings. For example, we can check if strings are equal using the equal to ==
operator.
if [ "$string1" == "$string2" ]; then
# Code to execute if string1 is equal to string2
fi
We may check if strings are not equal using the!=
operator.
if [ "$string1" != "$string2" ]; then
# Code to execute if string1 is not equal to string2
fi
These string operators provide powerful capabilities for manipulating and comparing strings in Bash scripts, allowing you to perform various string operations efficiently.
Logical Operators in Bash
In Bash scripting, logical operators are used to combine or negate conditions. These include AND (&&), OR (||), and NOT (!). These logical operators allow us to create complex conditional expressions by combining multiple conditions.
The AND (&&)
operator returns true (0) only if both conditions on its left and right sides are true. In the example below, it returns true if condition1 and condition2 are both true.
if [ condition1 ] && [ condition2 ]; then
# Code to execute if both condition1 and condition2 are true
fi
The OR (||
) operator returns true (0) if at least one of the conditions on its left or right side is true. In the example below if either condition1 or condition2 are true.
if [ condition1 ] || [ condition2 ]; then
# Code to execute if either condition1 or condition2 is true
fi
- NOT (
!
): The NOT (!
) operator negates a condition, returning true (0) if the condition is false and false (1) if the condition is true.
if ! [ condition ]; then
# Code to execute if condition is false
fi
These logical operators can be used to create complex conditional expressions in Bash scripts, allowing you to control the flow of your script based on multiple conditions.
File Test Operators in Bash
File test operators are a helpful and unique set of operators in Bash. They are useful for performing file-related operations in Bash scripts. They can be used to check various attributes of files, such as whether a file exists, is a regular file, is a directory, or is readable/writable/executable.
Here are the file test operators available in Bash:
The existence (-e) operator checks if a file exists.
if [ -e "$file" ]; then
# Code to execute if the file exists
fi
The ‘regular’ file (-f) operator checks if a file is a regular file (e.g. not a directory or a device file).
if [ -f "$file" ]; then
# Code to execute if the file is a regular file
fi
The directory (-d) operator checks if a file is a directory.
if [ -d "$dir" ]; then
# Code to execute if the file is a directory
fi
The readability (-r) operator checks if a file is readable.
if [ -r "$file" ]; then
# Code to execute if the file is readable
fi
The writability (-w) operator checks if a file is writable.
if [ -w "$file" ]; then
# Code to execute if the file is writable
fi
The Executability (-x) operator checks if a file is executable.
if [ -x "$file" ]; then
# Code to execute if the file is executable
fi
The File Size (-s) operator checks if a file has a size greater than zero.
if [ -s "$file" ]; then
# Code to execute if the file has a size greater than zero
fi
These file test operators provide a convenient way to check file attributes and perform different actions based on the results. They are essential for file management in Bash scripts.
Bash Operator Reference
1. Arithmetic Operators:
+
(Addition)-
(Subtraction)*
(Multiplication)/
(Division)%
(Modulus)
2. Comparison Operators:
-eq
(Equal to)-ne
(Not equal to)-lt
(Less than)-le
(Less than or equal to)-gt
(Greater than)-ge
(Greater than or equal to)
3. String Operators:
=
(Equal to)!=
(Not equal to)-z
(Empty string)-n
(Non-empty string)>
(Greater than, in ASCII order)<
(Less than, in ASCII order)
4. Logical Operators:
!
(Logical NOT)&&
(Logical AND)||
(Logical OR)
5. File Test Operators:
-e
(File exists)-f
(File is a regular file)-d
(File is a directory)-r
(File is readable)-w
(File is writable)-x
(File is executable)
6. Assignment Operators:
=
(Simple assignment)+=
(Append to variable)
7. Miscellaneous Operators:
:
(Null command, does nothing, used for syntax purposes).
(Source a script, execute commands in the current shell)
These operators can be combined with conditional statements (if
, elif
, else
) and loops (for
, while
, until
) to build complex scripts for various purposes.