Carbon Constants
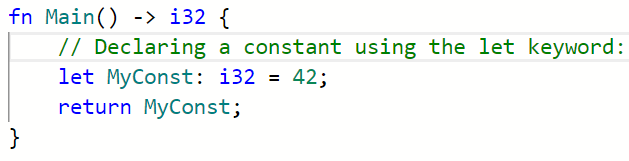
In Carbon, constants are irrefutable patterns that are matched to a value. Constants cannot be changed after they have been assigned a value.
Carbon uses the let keyword to declare a constant:
let MyConstant: i32 = 42;
Let’s break this down:
let is used to declare the constant
MyConstant is the name of the constant
i32 is the type of the variable, in this case a 32 bit signed integer
42 is the value being assigned to MyConstant
In summary, we are declaring a new constant called ‘MyConstant’. It is of type i32, which is a signed 32-bit integer. Finally, we set MyConstant equal to the value ’42’.
Constants vs. Variables in Carbon
There are two kinds of name-binding declarations in Carbon: constant declarations and variable declarations.
We’ve seen that constants are declared using the let keyword. Variables, on the other hand, are declared using the keyword var.
Declaration Keyword | Syntax | |
Constants | let | UpperCamelCase |
Variables | var | lower_snake_case |
Naming Convention for Constants in Carbon
Carbon uses UpperCamelCase for constants. This means that the first letter of each word in the name is capitalized and there are no separations.
UpperCamelCase is used in Carbon whenever the entity cannot have a dynamically changing value. Since constants (by definition) can’t be changed, we use UpperCamelCase for them.
In contrast, variables may be reassigned and the convention is to use lower_snake_case.
Compiler Errors with Constants
If an entity is declared to be a constant but its value is changed after being assigned, the compiler will throw an error.
Rectifying this can be as simple as replacing let with var. In order to maintain naming convention, lower_snake_case should be used.