Carbon Variables
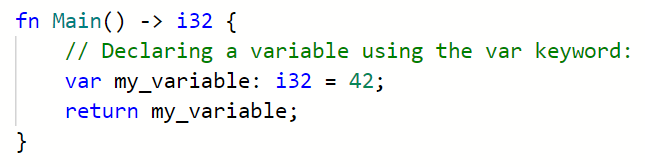
In Carbon, a variable is a modifiable pattern that is matched to a value. Variables can be changed after they have been assigned a value, unlike constants.
Carbon uses the var keyword to declare a variable:
var my_variable: i32 = 42;
Let’s break this down:
var is used to declare the variable
my_variable is the name of the variable
i32 is the type of the variable, in this case a 32 bit signed integer
42 is the value being assigned to my_variable
In summary, we are declaring a new variable called ‘my_variable’. It is of type i32, which is a signed 32-bit integer. Finally, we set my_variable equal to the value ’42’.
Variables vs. Constants in Carbon
Carbon uses two kinds of name-binding declarations: variable declarations and constant declarations. The value of a variable can be changed as many times as needed, but a constant can only be assigned a value once. Changing the value of a constant will result in an error.
Variables are declared using the keyword var, while constants are declared using the keyword let.
Variables and constants are also differentiated by naming convention.
Declaration Keyword | Syntax | |
Constants | let | UpperCamelCase |
Variables | var | lower_snake_case |
Naming Convention for Variables in Carbon
Carbon uses lower_snake_case for variables. This means that all letters are lowercase, and words are separated using an underscore.
In contrast, constants should use UpperCamelCase.