Go Language Comments
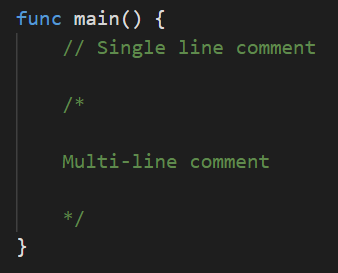
Comments can be used to explain code in Go and make it more readable. Go supports both single line and multi-line comments.
Go uses two forward slashes // to indicate a single line comment and /* */ for multi-line comments.
This is identical to comments in C/C++/Rust.
Comments aren’t just for annotation. They are also useful to prevent code from running, which is helpful when testing or tinkering with code.
Single Line Comments in Go
In Go, a single line comment can be created using two forward slashes //.
A single line comment starts with the double slash // and continues to the end of the line. Any text after the two forward slashes will not be executed:
// This is a single line comment
Single line comments can be placed at any point in the code:
// Comment outside main() function
func main() {
// Comment inside main() function
}
End of Line Comments in Go
A comment can be created to the right of executable code using two forward slashes. This is sometimes known as an end of line comment:
package main
import ("fmt")
func main() {
fmt.Println("Hello World!") // End of line comment
}
Multi-Line Comments in Go
Multi-line comments can be created using /* and */.
Multi line comments start with /* and end with */. Any text between /* and */ is ignored by the compiler.
/*
This is a multi-line comment
A mult-line comment can comment out multiple lines of text
/*
Errors With Multi-Line Comments
If a multi-line comment is accidentally left open or encompasses a part of the code that shouldn’t be commented out, this can lead to unexpected errors.
For example:
package main
import (
"fmt"
)
func main() {
fmt.Println("Hello, World!")
/*
}
Error:
prog.go:9:2: comment not terminated (and 1 more errors)
There are two errors here. The first is that the comment is unterminated; the second is that the main() function also isn’t terminated because the closing curly bracket ‘}’ is commented out.
Using Comments to Prevent Code Execution
Comments are useful for preventing code from executing. This can aid in debugging, isolating one part of the codebase from another, or just improving run time for faster development.
package main
import ("fmt")
func main() {
fmt.Println("Hello, World!")
// fmt.Println("Goodbye, World!")
}
Output:
Hello, World!
The above program doesn’t print out “Goodbye, World!” because the line is commented out.