Compare if Strings are Equal or Not Equal in Java
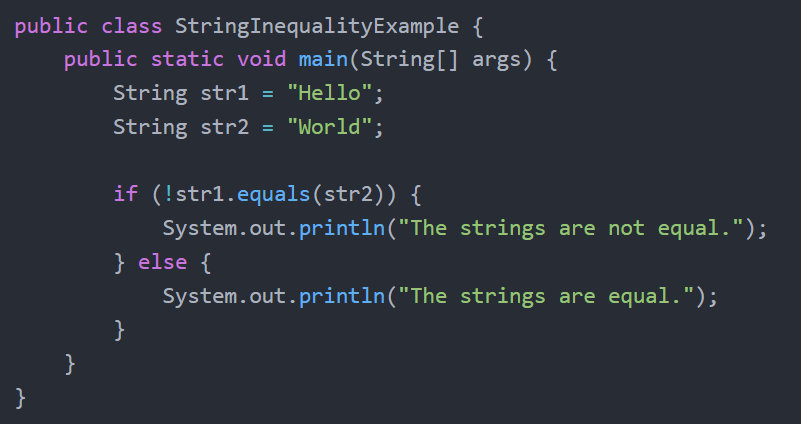
Java is one of the most popular and versatile programming languages, and it provides developers with a wide range of functionalities to handle various data types efficiently.
When it comes to working with strings, one of the most common, fundamental operations is comparing them to determine their equality or inequality.
This can be useful in a wide variety of situations, including validating user inputs, implementing conditional statements, manipulating textual data, and many others. In any case, understanding how to compare strings for equality or inequality is an important skill for any Java developer.
In this article, we delve into the different techniques and best practices for comparing strings in Java. From the conventional ‘==’ and ‘!=’ comparison operators to the more flexible and accurate ‘equals()’ method, we’ll explore the nuances of each approach and help you choose the right method for your specific use case.
Additionally, we’ll discuss potential pitfalls such as case sensitivity, and provide expert tips to ensure robust and error-free string comparisons.
Whether you are a new programmer just starting your Java journey or a seasoned developer seeking a quick reference, this guide aims to equip you with a comprehensive understanding of comparing strings in Java. Let’s dive in and master the art of string comparison in the Java programming language!
Methods for Comparing The Equality of Two Strings in Java
In this guide, we will be covering a number of different ways to comparing the equality of two strings in Java. These include using comparison operators like ‘==’ and ‘!=’, as well as several different methods that can be called to function on strings:
- Using comparison operators:
- The == (equality) operator to perform a basic equality comparison.
- The != (not equal) operator to check if two strings are not equal.
- The .equals() method.
- The !equals() method to test for inequality.
- The equalIgnoreCase() method to deal with case sensitivity.
- The compareTo() method to evaluate equality via lexicographic comparison.
- The compareToIgnoreCase() method, which incorporates case insensitivity into lexicographic evaluation.
Before we dive into these techniques, let’s do a brief review of what strings are in the Java programming language.
Strings in Java
In Java, a string represents a sequence of characters. There are two types of strings in Java: string literals and string objects. While both allow us to work with textual data, there are differences between them in terms of how they are created and stored in memory.
String Literals
String literals are the most common and convenient way to represent strings in Java. A string literal is a sequence of characters enclosed within double quotes (“).
Here’s an example of using a string literal:
String str = "Hello, World!";
When we declare a string using a string literal, Java automatically creates a string object and stores it in the string pool.
The string pool is a special area in the Java heap memory where unique string objects are stored, in order to conserve memory. When we create a string using a string literal, Java first checks to see if an identical string already exists in the pool. If it does, the new string refers to the existing object, minimizing memory usage. If the string does not exist in the pool, a new object is created and added to the pool.
String Objects
In contrast to string literals, we can create string objects explicitly using the `new` keyword and the `String` constructor.
Here’s an example of using a string object:
String greeting = new String("Hello, World!");
Whenever we create a string object, Java allocates memory on the heap to store the string data, regardless of whether an identical string already exists in the pool. This means that even if two string objects have the same value, they will be stored as separate objects in memory.
Creating string objects directly using the `new` keyword is less common because it can be less efficient compared to using string literals, due to the extra memory allocation and lack of string pooling.
The following table outlines key differences between string literals and string objects in Java:
String Literals | String Objects | |
Memory Usage | More efficient | Less efficient |
Mutability | Immutable | Immutable |
Usability | Ease of use | Greater control |
In general, it is recommended to use string literals whenever possible, as they provide better performance and memory efficiency in Java applications.
Now that we have a good understanding of strings in Java, let’s look at the most common ways to compare two strings for equality or inequality!
How to Compare The Equality of Two Strings in Java
As we mentioned earlier in the article, we’ll be covering the usage of several techniques and methods that are useful for comparing the equality or inequality of two strings in Java.
We’ll start with the simplest example: using the ‘==’ (equality) comparison operator.
Using The ‘==’ Operator
In Java, we can use the `==` (equality) comparison operator to compare the equality of two strings.
However, it’s important to understand that the `==` operator behaves differently for primitive data types (like `int`, `double`, etc.) and objects (like strings).
For primitive types, the `==` operator checks for value equality. In other words, it compares actual numerical or Boolean type values to determine if they are the same.
For objects, including strings, the `==` operator instead compares references, not the contents of the objects.
In the case of strings, it checks whether the two string variables refer to the same memory address, rather than whether the textual content of the strings is the same. The output of the ‘==’ operator is always a Boolean result (true or false).
Here’s an example of comparing strings using the `==` operator:
public class StringComparisonExample {
public static void main(String[] args) {
String str1 = "Hello";
String str2 = "Hello";
String str3 = new String("Hello");
System.out.println(str1 == str2);
System.out.println(str1 == str3);
}
}
Output:
true
false
In the example above, `str1` and `str2` are both string literals with the same value (“Hello”). When we compare these two strings using `==`, the result is `true` because they both refer to the same string literal in the string pool.
On the other hand, `str3` is created as a new string object using the `new` keyword. Even though it has the same textual content as `str1` and `str2`, it is stored as a separate object in the heap memory. Therefore, the comparison `str1 == str3` returns `false`.
To compare the actual content of strings for equality, we can use the `equals()` method provided by the `String` class, as it performs a content-based comparison. We’ll see examples of this below.
Before moving on to the equals() method, let’s see how the ‘!=’ operator can also be used to compare two strings for inequality.
Using The ‘!=’ Operator
In Java, we can use the `!=` operator to compare the inequality of two strings. Like the `==` operator, the `!=` operator compares the references of the two string objects, not their content. It checks whether the two strings refer to different memory locations.
Here’s an example of comparing strings for inequality using the `!=` operator:
public class StringInequality {
public static void main(String[] args) {
String str1 = "Hello";
String str2 = "World";
if (str1 != str2) {
System.out.println("The strings are not equal.");
} else {
System.out.println("The strings are equal.");
}
}
}
Output:
The strings are not equal
In this example, `str1` and `str2` are string literals with different values (“Hello” and “World”). Since they are separate objects in the string pool (different memory locations), the comparison `str1 != str2` evaluates to `true`, and the output will be “The strings are not equal.”
Remember that using the `!=` operator to compare strings only checks if they are different objects in memory. To compare the actual content of strings for inequality, we can use the `equals()` method, as it performs a content-based comparison.
Using the equals() Method
In Java, we can compare the equality or inequality of two strings using the `equals()` method, which is provided by the `String` class. The `equals()` method performs a content-based comparison, meaning it checks whether the textual content of the strings is the same.
Here’s how we can use the `equals()` method to compare strings for equality or inequality:
public class StringEqualityExample {
public static void main(String[] args) {
String str1 = "Hello";
String str2 = "Hello";
String str3 = new String("Hello");
String str4 = "World";
if (str1.equals(str2)) {
System.out.println("str1 and str2 are equal.");
} else {
System.out.println("str1 and str2 are not equal.");
}
if (str1.equals(str3)) {
System.out.println("str1 and str3 are equal.");
} else {
System.out.println("str1 and str3 are not equal.");
}
}
}
Output:
str1 and str2 are equal.
str1 and str3 are equal.
In the above code, `str1` and `str2` are both string literals with the same content (“Hello”). The `equals()` method correctly determines that they are equal.
On the other hand, `str1` and `str3` have the same content, but `str3` is created as a new string object using the `new` keyword. Unlike when we used the comparison (‘==’ and ‘!=’) operators, the equals() method evaluates to ‘true’ because the actual string contents are the same!
Using the `equals()` method is a common practice to compare the content of strings for equality or inequality in Java, because it ensures that we are comparing the textual values, not just the memory references.
Note: One thing to keep in mind is that the equals() method is case sensitive, meaning that “hello” and “Hello” would evaluate as not being equal. We’ll learn how to deal with this using the equalsIgnoreCase() method below. But first let’s see how to extend the equals() method to evaluate string inequality using the ‘!’ operator.
Using the !equals() Method
In Java, we can compare the inequality of two strings using the `!equals()` method.
This is really just an extension of the equals() method. The `equals()` method checks if the content of two strings is equal, and by using the negation operator `!` before the `equals()` method invocation, we can determine whether the content of the strings are not equal.
The following example shows how we can use the `!equals()` method to compare two strings for inequality:
public class StringInequalityExample {
public static void main(String[] args) {
String str1 = "Hello";
String str2 = "World";
if (!str1.equals(str2)) {
System.out.println("The strings are not equal.");
} else {
System.out.println("The strings are equal.");
}
}
}
Output:
The strings are not equal.
In the example above, we are comparing the string literals `str1` and `str2` using the `!equals()` method. Since the two strings have different contents (“Hello” and “World”), the `equals()` method returns `false`, and the negation operator `!` turns it into `true`.
As a result, the code inside the `if` block is executed, and the output is “The strings are not equal.”
The `equals()` method is case-sensitive, and therefore so is the ‘!equals()’ method. So, “hello” and “Hello” would be considered different strings. If you want a case-insensitive comparison, you can use the `equalsIgnoreCase()` method instead.
Dealing With Case-Sensitivity Using the equalsIgnoreCase() Method
In Java, we can compare the case-insensitive equality or inequality of two strings using the `equalsIgnoreCase()` method.
The `equalsIgnoreCase()` method checks if the content of two strings is equal, disregarding their case. By using the negation operator `!` before `equalsIgnoreCase()`, we can also determine whether the content of the strings is not equal (ignoring case).
Here’s an example of how we can use the `equalsIgnoreCase()` method to compare strings for case-insensitive equality and inequality:
public class CaseInsensitiveStringEquality {
public static void main(String[] args) {
String str1 = "Hello";
String str2 = "hello";
String str3 = "World";
// Compare for case-insensitive equality with equalsIgnoreCase()
if (str1.equalsIgnoreCase(str2)) {
System.out.println("The strings are equal.");
} else {
System.out.println("The strings are not equal.");
}
// Compare for case-insensitive inequality with !equalsIgnoreCase()
if (!str1.equalsIgnoreCase(str3)) {
System.out.println("The strings are not equal.");
} else {
System.out.println("The strings are equal.");
}
}
}
Output:
The strings are equal.
The strings are not equal.
In the example above, we compare the strings `str1` and `str2` using the `equalsIgnoreCase()` method. Since the two strings have the same textual content (“Hello” and “hello”) but differ in case, the `equalsIgnoreCase()` method returns `true`. As a result, the output is “The strings are equal.”
Next, we compare `str1` and `str3`, which have different contents (“Hello” and “World”). The `equalsIgnoreCase()` method returns `false`, and the negation operator `!` turns it into `true`, causing the code inside the `if` block to execute. Therefore, the output is “The strings are not equal.”
Using `equalsIgnoreCase()` is a handy approach when you need to perform case-insensitive string comparisons in Java, such as when dealing with user inputs or comparing strings from external data sources.
Using the CompareTo() Method
In Java, we can compare the equality or inequality of two strings using the `compareTo()` method. The `compareTo()` method is used to determine the lexicographic order of two strings.
If two strings are the same the CompareTo() method will output zero. Otherwise, CompareTo() will output the difference.
The following code shows how we can use the `compareTo()` method to compare strings for equality or inequality:
public class StringComparisonExample {
public static void main(String[] args) {
String str1 = "Hello";
String str2 = "Hello";
String str3 = "World";
int result1 = str1.compareTo(str2);
if (result1 == 0) {
System.out.println("str1 and str2 are equal.");
} else {
System.out.println("str1 and str2 are not equal.");
}
int result2 = str1.compareTo(str3);
if (result2 == 0) {
System.out.println("str1 and str3 are equal.");
} else {
System.out.println("str1 and str3 are not equal.");
}
}
}
Output:
str1 and str2 are equal.
str1 and str3 are not equal.
In the example above, we first compare the strings `str1` and `str2`. Since the two strings have the same textual content (“Hello”), the `compareTo()` method returns `0`. This indicates that the strings are equal.
Next, we compare `str1` and `str3`, which have different contents (“Hello” and “World”). The `compareTo()` method returns a value less than `0` when `str1` comes before `str3` in lexicographic order.
If we want to check for inequality, we can simply use the negation of the equality comparison:
public class StringComparisonExample {
public static void main(String[] args) {
String str1 = "Hello";
String str2 = "Hello";
// Compare for inequality
if (!(str1.compareTo(str2) == 0)) {
System.out.println("The strings are not equal.");
} else {
System.out.println("The strings are equal.");
}
}
}
Output:
The strings are equal.
In this example, the negation operator `!` is used to check for inequality. Since `str1` and `str2` have the same content (“Hello”), the result of `compareTo()` is `0`, and the negation turns it into `false`. Thus, the code inside the `else` block is executed, and the output is “The strings are equal.”
While the `compareTo()` method can be used to compare strings for equality or inequality, it’s essential to understand that its primary functions is to establish the lexicographic order of strings. For direct content-based comparisons, using the `equals()` or `equalsIgnoreCase()` methods is a more straightforward and preferred approach.
public class StringInequality {
public static void main(String[] args) {
String str1 = "Hello";
String str2 = "World";
if (!str1.equals(str2)) {
System.out.println("The strings are not equal.");
} else {
System.out.println("The strings are equal.");
}
}
}
“`
Case-Insensitive Lexicographic Comparison with compareToIgnoreCase()
We can compare the case-insensitive equality or inequality of two strings using the `compareToIgnoreCase()` method. The `compareToIgnoreCase()` method is similar to the `compareTo()` method, but it performs a case-insensitive comparison of the two strings.
If the strings are not equal (disregarding case), compareToIgnoreCase() returns an integer value that indicates the case-insensitive lexicographic difference between the two strings.
Here’s an example of how we can use the `compareToIgnoreCase()` method to compare strings for case-insensitive equality or inequality:
public class CaseInsensitiveStringComparisonExample {
public static void main(String[] args) {
String str1 = "Hello";
String str2 = "hello";
String str3 = "World";
// Compare for case-insensitive equality
int result1 = str1.compareToIgnoreCase(str2);
if (result1 == 0) {
System.out.println("The strings are equal (case-insensitive).");
} else {
System.out.println("The strings are not equal (case-insensitive).");
}
int result2 = str1.compareToIgnoreCase(str3);
if (result2 == 0) {
System.out.println("The strings are equal (case-insensitive).");
} else {
System.out.println("The strings are not equal (case-insensitive).");
}
}
}
Output:
The strings are equal (case-insensitive).
The strings are not equal (case-insensitive).
In the example above, we first compare the strings `str1` and `str2` using the `compareToIgnoreCase()` method. Since the two strings have the same textual content (“Hello” and “hello”), even though they differ in case, the `compareToIgnoreCase()` method returns `0`.
In the second example, we compared `str1` and `str3`, which have different contents (“Hello” and “World”). The `compareToIgnoreCase()` method returns a value greater than `0`, indicating that the first string (`str1`) comes before the second string (`str3`) in lexicographic order when ignoring the case.
Using the `compareToIgnoreCase()` method is useful when you need to compare strings in a case-insensitive manner and also want to know their lexicographic order. It provides a flexible way to handle string comparisons based on your specific requirements.
Conclusion
In this comprehensive article, we explored a number of methods available in Java to compare strings for equality or inequality.
We began by exploring the difference between string literals and string objects, understanding their differences in memory allocation and immutability. We saw that because string literals leverage the string pool for memory optimization, they are the preferred choice for most scenarios.
Next, we explored a range of methods for comparing strings, each offering unique functionalities best suited to specific requirements. We covered the traditional usage of the ‘==’ and ‘!=’ comparison operators, which compare memory references and should be used cautiously with string objects to avoid unexpected results.
Moving on, we explored the robust ‘equals()’ method, the go-to approach for most content-based comparisons. This method allows us to check if two strings share the same textual content. This ensures reliable and accurate comparisons.
To account for case-insensitive scenarios, we examined the ‘equalsIgnoreCase()’ method. This powerful method is valuable when we require comparisons that overlook differences in letter case, expanding the versatility of our string evaluations.
Lastly, we learned about the ‘compareTo()’ and ‘compareToIgnoreCase()’ methods, primarily designed for lexicographic ordering but also useful for assessing string equality or inequality. While these methods provide an interesting alternative, we emphasized their primary purpose and recommended opting for the dedicated content-based methods for simple, straightforward
comparisons.
By understanding and effectively utilizing these methods, Java developers can confidently evaluate string equality or inequality based on project specific requirements. Choosing the most appropriate comparison method empowers programmers to develop reliable and efficient applications, making Java programming even more versatile and enjoyable.
As you continue on your Java programming journey, bear in mind the importance of selecting the right comparison method and, feel free to tailor them to your unique needs. With this knowledge at your disposal, you are now well-equipped to harness the full potential of string comparisons in Java.
Happy coding!
Interested in learning more about programming? Check out our other content or join our community of fellow programmers by signing up for our monthly newsletter! (Sign up below).