How to Convert Integer to Char in Java? With Examples
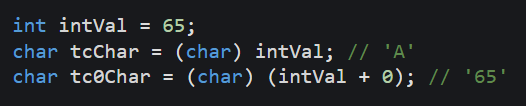
A common challenge in Java programming is converting an integer to a character, which might seem straightforward at first glance. However, converting an int to a char in Java entails a few nuances that developers need to understand and navigate to avoid potential pitfalls and ensure accurate conversions.
In addition, there are several easy methods available, each with its’ own use case.
In this Java tutorial, we will explore several different ways of converting an integer to a character in Java. The following table shows a brief description of the various methods we’ll be covering so that you can choose to jump to a section.
Description | |
Typecasting | Converts int to Unicode character |
Typecasting + 0 | Converts int to Unicode character; Uses an offset to output a number (0-9) e.g. int ‘3’ => char ‘3’ |
Character.forDigit() | Converts to a number (0-9) Convert to any number base up to 36 Can do base conversions, i.e. binary to decimal |
toString() | Converts any digit of an int to a char e.g. int ‘423’ => char ‘4’ or ‘2’ or ‘3’ |
We’ll see these various methods available within the Java language that enable int to char conversion, and take a deep look at their differences and use cases. Throughout this journey, we’ll provide practical examples and step-by-step demonstrations to solidify your understanding and enable you to apply these lessons in your own projects.
Whether you’re a new Java programmer looking for a fundamental grasp of data type conversions or an experienced developer looking to refresh your knowledge, this article will equip you with the necessary insights to tackle integer-to-character conversions effectively. Let’s master the art of converting integers to characters in Java!
Integers vs. Characters in Java
Integers and characters are both primitive data types in Java, but there are many differences between them. Before we tackle the details of converting an integer to a character in Java, it is useful to review the characteristics of these two data types so that we can understand how type conversion actually works.
Let’s start with a brief overview of characters.
Characters in Java
In Java, characters (chars) have the following characteristics:
- Representation: The ‘char’ data type is used to represent a single 16-bit Unicode character. It can hold any character from the Unicode character set, including letters, digits, symbols, and special characters. This allows Java to support a wide range of characters from various languages and character sets.
- Size: Characters in Java occupy 16 bits (2 bytes) of memory. This fixed size ensures consistent memory usage for character variables, making them memory-efficient.
- Widening Conversion: Characters can be implicitly converted to integers (int) due to their 16-bit representation. This means you can use a ‘char’ variable in an arithmetic expression or assign its value to an ‘int’ variable without explicit casting.
- Single Quotes for Character Literals: Character literals are written within single quotes, like ‘A’, ‘9’, ‘$’, etc. The single quotes distinguish character literals from string literals, which are enclosed in double quotes.
Characters in Java play an important role in handling individual characters within strings, character-level processing, and text manipulation. They provide a compact and memory-efficient way to represent and work with characters from various languages and symbol sets, making Java well-suited for internationalization and multilingual applications.
Integers in Java
There are actually four types of integers in Java, each with their own size and range. The int type is the most common, but all four are listed below in size order:
- byte: Occupies 8 bits of memory and can represent values from -128 to 127. It is often used in situations where memory efficiency is crucial, or when dealing with data that fits within its limited range.
- short: Occupies 16 bits of memory and can represent values from -32,768 to 32,767. Being larger than a byte, it is considered a higher data type but lower than anSimilar to ‘byte’, ‘short’ is used when memory conservation is essential or when dealing with data that falls within its range.
- int: Occupies 32 bits of memory and can represent values from approximately -2.1 billion to +2.1 billion (-2^31 to 2^31 – 1). ‘int’ is the default choice for most integer-based operations and is used for a wide range of numeric calculations. It is a lower data type than a long, but sufficiently large for most applications.
- long: The ‘long’ data type is the largest among the integer data types. It occupies 64 bits of memory and can represent values from approximately -9.2 quintillion to +9.2 quintillion (-2^63 to 2^63 – 1). A type of ‘long’ is used when dealing with extremely large integer values that go beyond the range of ‘int’.
It’s important to choose the appropriate integer type based on the range and memory requirements of the data you are working with. For most general-purpose cases, ‘int’ is the preferred choice, but for specialized scenarios where memory conservation or large values are involved, ‘byte’, ‘short’, or ‘long’ might be more suitable.
Converting an Integer to a Character in Java
There are several popular methods for converting an integer to a character, which we’ll cover in the following sections.
Note: It should be somewhat obvious, but one of the main limitations when converting an integer to a character is that a char is only one character long. This means that we can’t convert an integer with more than one digit into a character having more than one digit. In other words, we can convert an integer with a value of 0-9 directly to a character of value 0-9 but we can’t go any higher without using letters or other characters. It is, however, easy to convert an integer to a character with any Unicode value, as we will see below!
Convert an int to a char with Typecasting
The simplest way to convert an int to a char in Java is by typecasting. Typecasting is the process of explicitly converting a value from one data type to another, allowing us to change the data representation as needed.
When we typecast an ‘int’ to a ‘char’, we are essentially mapping the integer value to its corresponding Unicode/ASCII equivalent character. In other words, the numeric value of the integer will be interpreted as a corresponding Unicode character.
Here’s one example of how we can convert an ‘int’ to a ‘char’ using typecasting:
public class IntToCharExample {
public static void main(String[] args) {
// Define the int value that we want to convert to char
int intVal = 65; // Numeric '65' corresponds with an ASCII value of 'A'
// Typecasting to convert the int to char
char charVal = (char) intVal;
// Print the original int value and the converted char value
System.out.println("int value: " + intVal);
System.out.println("char value: " + charVal);
}
}
Output:
int value: 65
char value: A
In the example above, we are typecasting the integer value 65 to its corresponding character ‘A’. This is called ‘typecasting‘ because we are casting the value of the integer variable intVal into the character variable charVal.
It’s essential to note that when converting an ‘int’ to a ‘char’, the ‘int’ value should represent a valid Unicode character code point within the range of the ‘char’ data type. If the ‘int’ value is outside the valid range for ‘char’, the resulting ‘char’ will represent a different character, and the conversion may not be meaningful. Always look up the value of an integer being converted in a Unicode or ASCII table to avoid any potential surprises.
Always ensure that you understand the underlying Unicode representation and the potential limitations when performing explicit type casting in Java.
Typecast to The Same Numeric Value By Adding ‘0’
One common scenario is when we want to convert an integer from 0-9 directly into a character having the same numeric value (e.g. an int of ‘1’ to a char of ‘1’). To do this, we can simply add ‘0’ while performing the type casting, i.e.
char charValue = (char) (intValue + ‘0’);
This works because in Java, characters are represented as Unicode code points, and the ASCII value of the digit ‘0’ is 48. When we add an integer value between 0 and 9 to the character ‘0’, it gives us the corresponding character representation of that digit.
Here’s an example showing how we can put this into practice:
public class IntToCharExample {
public static void main(String[] args) {
// Step 1: Define the int value that you want to convert to char
int intValue = 5;
// Step 2: Use type casting to convert the int to char by adding '0'
char charValue = (char) (intValue + '0');
// Step 3: Print the original int value and the converted char value
System.out.println("Original int value: " + intValue);
System.out.println("Converted char value: " + charValue);
}
}
Output:
Original int value: 5
Converted char value: 5
In this approach, we use typecasting to convert the int value to a char by adding ‘0’ to it.
For example, when ‘intValue’ is 5, adding ‘0’ to it will result in 53, which corresponds to the character ‘5’.
Note that this approach is only valid when converting integers that represent digits (0 to 9) to their corresponding characters. If we try to convert an int value outside this range or representing other characters, the result may not be meaningful. For more general conversions between int and char type variables, other methods like `Character.forDigit()` or simple typecasting should be used.
Convert an int to a char With Character.forDigit()
In Java, we can convert an integer data type to a character using the `Character.forDigit()` method. The `Character.forDigit()` method was specifically designed to convert an integer value (typically representing a digit) to a character representation in any number base up to 36.
The `Character.forDigit()` method in Java is a static method from the `Character` class. This method is particularly helpful when dealing with digit-to-character conversions, such as converting an integer value to its corresponding character representation in a specific radix (base). It takes two arguments: the digit to be converted, and a radix. We’ll cover the specific syntax and how to work with Character.forDigit() in the next few sections.
The syntax of the `Character.forDigit()` method is as follows:
public static char forDigit(int digit, int radix)
Parameters:
- Digit: The integer value (digit) to be converted to a character. The digit must be within the range of 0 to ‘radix – 1’. In other words, if the radix is 10 then the digit must be from 0 to 9.
- Radix: The radix (base) of the numeral system used to interpret the ‘digit’ parameter. For binary, the radix value is 2; for octal it is 8, and for decimal it is 10. Java supports a radix of up to 36.
Return Value:
- The Character.forDigit() method returns the character representation of the given ‘digit’ in the specified ‘radix’.
How the Character.forDigit() method works:
- The `Character.forDigit()` method first checks if the ‘digit’ parameter is within the valid range for the specified radix (0 to ‘radix’ – 1). If the ‘digit’ is outside this range, the method returns the null character (`’\u0000’`).
- If the ‘digit’ is within the valid range, the method then calculates the corresponding character representation based on the radix. For example, if the radix is 10 (decimal), ‘digit’ values 0 to 9 will be converted to characters ‘0’ to ‘9’, respectively. For values above 9, a radix greater than 10 is required, and the returned character will be a letter.
- The method then returns the character representation of the ‘digit’. This may range from the number 0 (digit value ‘0’) to the letter z (digit value 35).
The following example shows how to perform int conversion to char using the Character.forDigit() method:
int digit = 3;
char charValue = Character.forDigit(digit, 10);
System.out.println("Converted char value: " + charValue);
Output:
Converted char value: 3
In the above example, we convert the integer value 3 to its corresponding character ‘3’ using `Character.forDigit(3, 10)`. The first argument, ‘digit’, corresponds to the integer that we are converting, while the second argument ’10’, is the radix and indicates that we want to perform a base-10 conversion.
Remember that the `Character.forDigit()` method is primarily intended for converting digits to characters. If you need to perform more general integer-to-character conversions, where the integer value may not represent a digit, typecasting or other methods should be used instead.
Convert an int to a char Using toString()
Another powerful way to convert an integer to a char type is by using the toString() method. This method allows us to convert any specific digit from an integer to a character. This is a two-step process: first we convert the integer to its’ string representation, and then we grab a character from the string.
One advantage of this method is that it allows us to grab any of the digits from an integer and convert it directly into a char, rather than the entire integer. In many applications, it may only be the first or the second digit that we want to turn into a char for some other data processing. This method allows us to do that easily. However for more simple conversions, the above methods might be better.
The following code shows how to convert an int to a char in Java using the `toString()` method:
public class IntToCharExample {
public static void main(String[] args) {
// Step 1: Define the int value that you want to convert to char
int number = 423;
// Step 2: Convert the int to a String using the toString() method
String str = Integer.toString(number);
// Step 3: Get the first character from the String
char a = str.charAt(0);
char b= str.charAt(1);
char c = str.charAt(2);
// Step 4: Print the original int value and the converted char value
System.out.println("int value: " + number);
System.out.println("char with first digit: " + a);
System.out.println("char with second digit: " + b);
System.out.println("char with third digit: " + c);
}
}
Output:
int value: 423
char with first digit: 4
char with second digit: 2
char with third digit: 3
In the above code, we first convert the integer variable ‘intValue’ to a string using the `Integer.toString(intValue)` method. Next, we use the `charAt(index)` method to retrieve each character from the resulting string using index notation and converted them individually into a single character. We then printed all three chars to the console to demonstrate that we can now work with each one individually.
To reiterate, the strength of this method is that it allows us to convert any of the digits in an integer to a corresponding character. This method is powerful, but it may be overkill depending on your application.
Conclusion
Throughout this article, we have explored several different approaches to achieve integer to character conversion in Java, showcasing both typecasting and the use of helpful built-in methods like `Character.forDigit()` and `toString()`. Each technique has its advantages and use cases, allowing developers to choose the most appropriate method based on the specific needs of a project. Typecasting is often the best option, but there are many of use cases for the other methods as well.By understanding the characteristics of integers and characters in Java, we gained insights into their relative advantages and limitations. Integers excel at mathematical
and handling a wide range of numeric data, while characters are highly efficient and play a crucial role in representing individual characters and enabling character-level processing.
The power of type conversion enables us to transform data from one type to another seamlessly, and our exploration of converting integers to characters demonstrates the usefulness of having different methods in our wheelhouse. Additionally, we also learned how to leverage radix parameters to facilitate conversions between various numeric base systems, enriching our understanding of the Java language’s versatility.
As developers, we should always exercise caution and ensure that conversions are performed within valid ranges and avoid unexpected results. It’s important to do robust testing to ensure that our applications will work no matter what we throw at them. Whether we’re dealing with simple single-digit integers or more complex numerical representations, it is essential that we choose the appropriate conversion method and consider the specific context of our specific Java program.
With the knowledge gained from this article and the practical examples provided, you should be able to confidently tackle challenges related to integer-to-character conversions in Java.
Happy coding!
Learning Java? Check out our main Java page for more helpful tutorials!