How to Print a HashMap in Java
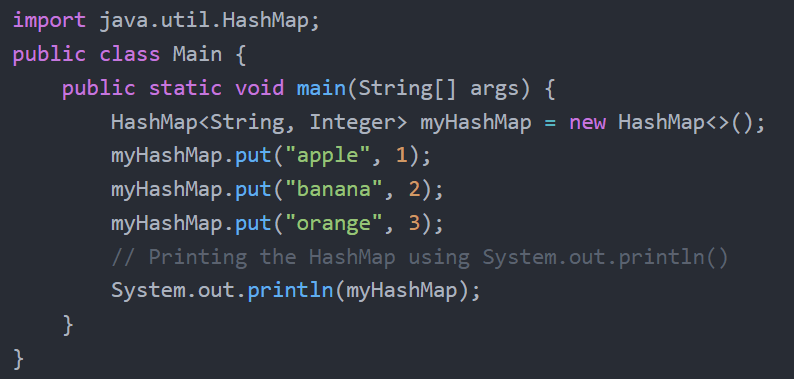
A HashMap is a powerful and widely used data structure that allows Java developers to store and manage key-value pairs efficiently.
HashMaps provide fast access and retrieval of data, making them an essential tool in various Java applications. However, while manipulating and accessing data within a HashMap is straightforward, printing its contents for debugging or analysis purposes can get a bit tricky.
In this article, we will delve into the fundamentals of HashMaps in the Java programming language, explaining their underlying principles and functionalities. We will explore various methods that will help you print the contents of a HashMap effectively, and look at the relative strengths of each method. Whether you are a novice Java programmer looking to grasp the basics or an experienced developer seeking advanced techniques, this guide will cater to your needs.
Let’s begin by understanding the core concepts of HashMaps and then proceed to learn approaches for printing their contents in Java. By the end of this article, you will have a comprehensive understanding of HashMaps and the confidence to deploy the appropriate printing methods based on your specific use cases.
- Review of HashMaps in Java
- Creating a HashMap in Java
- Common HashMap Tasks and Methods
- Printing a hashmap in Java
- Printing a HashMap With System.out.println()
- Using the keySet(), entrySet(), and Values() Methods
- Print Java HashMap Using Arrays.asList()
- Print Using Collections.singletonList()
- Print HashMap Using BiConsumer
- Print a HashMap Using an Iterator
- Print a HashMap Using Custom Objects as Keys and Values
- Conclusion
Review of HashMaps in Java
Before diving into the details of printing HashMaps, let’s first review their properties within the context of the Java programming language.
In Java, a HashMap is a widely used implementation of the Map interface, part of the Java Collections Framework. It provides a data structure that allows developers to store and manage key-value pairs in a highly efficient manner.
HashMaps are based on the principle of hashing, which enables rapid access and retrieval of elements, making them ideal for scenarios where quick data retrieval is crucial.
The basic idea behind a HashMap is to associate a set of keys with corresponding values, allowing easy access to values using their associated keys. Each key in a HashMap must be unique, and it cannot have duplicate entries. However, multiple keys can be associated with the same value.
The primary features and characteristics of HashMaps in Java are as follows:
- Key-Value Pairs: Every element in a HashMap is stored as a key-value pair, where the key represents a unique identifier for the value that it maps to.
- Dynamic Size: Unlike arrays, HashMaps in Java can automatically resize themselves to accommodate new elements as they are added.
- Null Values: HashMaps allow unlimited null values as well as one null key. This means that we can map a value to a null key or have a null value associated with a non-null key.
- Unordered Collection: HashMaps do not maintain any specific order of elements, meaning the order in which elements are stored is not preserved. If you need ordered key-value pairs, you can instead use the LinkedHashMap class.
- Performance: HashMaps are known for their fast retrieval times, typically offering O(1) constant time complexity for retrieval, insertion, and deletion operations under optimal circumstances. Note that in certain scenarios with hash collisions, this performance can degrade to O(n).
- Hashing Mechanism: HashMap uses a hashing mechanism that converts keys into unique hash codes, which are used to determine the index of the corresponding value in the underlying array. This mechanism ensures fast access to elements.
- Iteration: HashMaps can be efficiently iterated through using various methods to access and process their keys, values, or key-value pairs.
Overall, HashMaps in Java are an essential data structure, widely used in various applications, including caching, data retrieval, and general-purpose storage of key-value associations.
Understanding the principles and usage of HashMaps is crucial for any Java developer to efficiently manage and manipulate data in their programs.
Creating a HashMap in Java
We can create a HashMap in Java using the following steps:
Step 1: Import the java.util package
Before using the HashMap class, we need to import the `java.util` package, which contains the HashMap class and other essential classes and interfaces for collections.
import java.util.HashMap;
Step 2: Declare and instantiate the key-value pairs of HashMap
To create a HashMap, we need to declare a variable of type `HashMap<K, V>`, where `K` represents the type of keys, and `V` represents the type of values you want to associate with those keys. Then, we can use the `new` keyword to instantiate our HashMap.
For example, if we want to create a HashMap that associates string keys with integer values, we could use the following:
HashMap<String, Integer> myHashMap = new HashMap<>();
That’s it! Our hashmap has already been created.
Note that the type of the key is a string, while the type of each value is an integer. In the next section, we’ll review some common methods for working with Java HashMaps before moving on to printing them.
Common HashMap Tasks and Methods
Adding elements to a HashMap
We can add elements to a HashMap using the `put()` method. The `put()` method takes two arguments: the key and the specified value we want to associate with that key.
myHashMap.put("apple", 1);
myHashMap.put("banana", 2);
myHashMap.put("orange", 3);
Accessing elements from a HashMap
We can use the `get()` method to access elements in a HashMap, passing the key as an argument. The `get()` method returns the value of the key that we specify.
int appleValue = myHashMap.get("apple"); // appleValue will be 1
Remove elements from a HashMap
If we need to remove HashMap elements, we can use the `remove()` method and pass the key as an argument.
myHashMap.remove("banana");
Check if a HashMap contains a key
To check if a HashMap contains a specific key, we can use the `containsKey()` method. This returns a Boolean (true or false):
boolean containsKey = myHashMap.containsKey("orange");
Check if a HashMap is empty
We can check if a HashMap is empty using the `isEmpty()` method. Like the containsKey() method, this also returns a Boolean.
boolean isEmpty = myHashMap.isEmpty();
That’s it! We have successfully created a HashMap in Java and performed basic operations on it.
HashMaps are powerful data structures that offer fast access and manipulation of key-value pairs, making them essential in various Java applications. Now let’s move on to the main topic of this article: printing the contents of HashMap!
Printing a hashmap in Java
There are many different ways of printing a HashMap in Java. The wide array of methods available for printing a HashMap can itself be daunting, so we will cover the easiest and most common techniques first.
Keep in mind that one of the reasons that there are so many ways to print a HashMap is that there are different things that we may actually want to print. For example, we may want to print the entire HashMap, print its keys, or print the associated value or set of values for a given set of keys. We may also want to print the data in a specific format.
The following techniques will cover many different use cases, and it’s a good idea to get a feel for each one. If you’re having trouble, it may be a good idea to try out a different method to see what it can offer.
Printing a HashMap With System.out.println()
The easiest way to print a HashMap is by simply passing it into `System.out.println()` without using any additional method or loop. Behind the scenes, this technique makes use of the `toString()` method.
The `toString()` method is a method of the HashMap class and provides a string representation of the entire HashMap. When we print a HashMap using `System.out.println()`, it automatically calls the `toString()` method, which displays the key-value pairs in the HashMap.
Here’s an example of how we can do this:
import java.util.HashMap;
public class Main {
public static void main(String[] args) {
HashMap<String, Integer> myHashMap = new HashMap<>();
myHashMap.put("apple", 1);
myHashMap.put("banana", 2);
myHashMap.put("orange", 3);
// Printing the HashMap using System.out.println()
System.out.println(myHashMap);
}
}
Output:
{apple=1, banana=2, orange=3}
The `toString()` method provides a concise and straightforward representation of the HashMap’s key-value pairs using a simple print statement.
However, it’s important to note that the order of the elements in the output may not be the same as the insertion order. If we need to maintain a specific order, you can use a LinkedHashMap, which maintains the insertion order of elements.
Using the keySet(), entrySet(), and Values() Methods
There are several other methods that make use of System.out.println() to print a HashMap in Java and extend the functionality of this simple technique. For example, these methods can be used to access and display the keys, values, or key-value pairs.
Print Keys and Values using `keySet()` and `get()` methods:
import java.util.HashMap;
public class Main {
public static void main(String[] args) {
HashMap<String, Integer> myHashMap = new HashMap<>();
myHashMap.put("apple", 1);
myHashMap.put("banana", 2);
myHashMap.put("orange", 3);
// Printing keys and values
for (String key : myHashMap.keySet()) {
System.out.println("Key: " + key + ", Value: " + myHashMap.get(key));
}
}
}
Output:
Key: apple, Value: 1
Key: banana, Value: 2
Key: orange, Value: 3
Print Key-Value Pairs using `entrySet()` method:
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
HashMap<String, Integer> myHashMap = new HashMap<>();
myHashMap.put("apple", 1);
myHashMap.put("banana", 2);
myHashMap.put("orange", 3);
// Printing key-value pairs
for (Map.Entry<String, Integer> entry : myHashMap.entrySet()) {
System.out.println("Key: " + entry.getKey() + ", Value: " + entry.getValue());
}
}
}
Output:
Key: apple, Value: 1
Key: banana, Value: 2
Key: orange, Value: 3
Technique 3: Print Values using `values()` method:
import java.util.HashMap;
public class Main {
public static void main(String[] args) {
HashMap<String, Integer> myHashMap = new HashMap<>();
myHashMap.put("apple", 1);
myHashMap.put("banana", 2);
myHashMap.put("orange", 3);
// Printing values
for (Integer value : myHashMap.values()) {
System.out.println("Value: " + value);
}
}
}
Output:
Value: 1
Value: 2
Value: 3
You can choose the approach that best suits your needs based on whether you want to print keys, values, or key-value pairs in your HashMap.
Print Java HashMap Using Arrays.asList()
Another common method for printing a HashMap is by using the Arrays.asList() method.
To print the contents of a HashMap using `Arrays.asList()` method, we need to first convert the HashMap’s key-value pairs into a list of Map.Entry objects. Then we can use `Arrays.asList()` to convert the list into a string value representation. Here’s an example:
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
HashMap<String, Integer> myHashMap = new HashMap<>();
myHashMap.put("apple", 1);
myHashMap.put("banana", 2);
myHashMap.put("orange", 3);
// Convert HashMap to a list of Map.Entry objects
String hashMapAsString = Arrays.asList(myHashMap.entrySet()).toString();
// Printing the HashMap using System.out.println()
System.out.println(hashMapAsString);
}
}
Output:
[apple=1, banana=2, orange=3]
Please note that the `Arrays.asList()` method is not specifically designed for handling Map.Entry objects. Therefore, the output is not as neat and straightforward as the `toString()` method of the HashMap. Additionally, the order of elements in the output may not be the same as the insertion order, similar to the `toString()` method.
While using `Arrays.asList()` to print a HashMap is possible, it’s often simpler to use the `toString()` method directly, as shown in previous examples. If you need more control over the output format or order, you might consider iterating through the HashMap’s key-value pairs manually and constructing the output as per your project’s requirements.
Print Using Collections.singletonList()
This method of printing a HashMap utilizes the Collections.singletonList() method, which packages the HashMap as a list containing a single element.
To print the contents of a HashMap using `Collections.singletonList()`, we need to first convert the HashMap’s key-value pairs into a list of Map.Entry objects and then use `Collections.singletonList()` to wrap that list as a single element list. Here’s how we can do it:
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
HashMap<String, Integer> myHashMap = new HashMap<>();
myHashMap.put("apple", 1);
myHashMap.put("banana", 2);
myHashMap.put("orange", 3);
// Convert HashMap to a list of Map.Entry objects and wrap it as a single-element list
String hashMapAsString = Collections.singletonList(myHashMap.entrySet()).toString();
// Printing the HashMap using System.out.println()
System.out.println(hashMapAsString);
}
}
Output:
[[apple=1, banana=2, orange=3]]
Please note that using `Collections.singletonList()` here will create a list containing one element, which is the set of key-value pairs in the form of `Map.Entry` objects. The output includes an extra square bracket around the entire list, which might not be the most straightforward representation for printing a HashMap but can be helpful for specific use cases.
As mentioned in previous examples, the `toString()` method of the HashMap provides a more concise and user-friendly representation of the key-value pairs. Using `Collections.singletonList()` to print a hash map may not be the most common approach, but it’s possible if you have specific use cases that require the data to be formatted this way.
Print HashMap Using BiConsumer
We can use `BiConsumer` for more control over the output format when printing a HashMap.
`BiConsumer` is a functional interface that takes two arguments (key and value in this case) and performs an action using those arguments. Inside the lambda expression `(key, value) -> System.out.println(“Key: ” + key + “, Value: ” + value)`, we define a given action that we want to take for each key-value pair, which in this case is printing them in a specific format.
To print the contents of a HashMap using `BiConsumer`, we can use the `forEach` method from the Java HashMap class. `forEach` takes a `BiConsumer` as an argument, which allows us to define a custom action for each key-value pair in the HashMap.
This way, we can print the key-value pairs in a way that suits a desired format.
Here’s an example of how we can use `BiConsumer` to print a HashMap:
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
HashMap<String, Integer> myHashMap = new HashMap<>();
myHashMap.put("apple", 1);
myHashMap.put("banana", 2);
myHashMap.put("orange", 3);
// Printing the HashMap using BiConsumer
myHashMap.forEach((key, value) -> System.out.println("Key: " + key + ", Value: " + value));
}
}
Output:
Key: apple, Value: 1
Key: banana, Value: 2
Key: orange, Value: 3
In the code above, we use the `forEach` method of the HashMap, which takes a `BiConsumer`.
Using `BiConsumer` to print the HashMap gives you more control over the output format, and it is often preferred whenever we need custom formatting or additional processing for each key-value pair. It’s a powerful tool that we can use to work with the elements in the HashMap in a flexible and customized manner.
Print a HashMap Using an Iterator
To print the contents of a HashMap using an Iterator, we can first obtain an Iterator from the HashMap’s entry set and then iterate through the key-value pairs to print them. Here’s how we can do this:
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
public class Main {
public static void main(String[] args) {
HashMap<String, Integer> myHashMap = new HashMap<>();
myHashMap.put("apple", 1);
myHashMap.put("banana", 2);
myHashMap.put("orange", 3);
// Get an Iterator from the entry set of the HashMap
Iterator<Map.Entry<String, Integer>> iterator = myHashMap.entrySet().iterator();
// Printing the HashMap using the Iterator
while (iterator.hasNext()) {
Map.Entry<String, Integer> entry = iterator.next();
System.out.println("Key: " + entry.getKey() + ", Value: " + entry.getValue());
}
}
}
Output:
Key: apple, Value: 1
Key: banana, Value: 2
Key: orange, Value: 3
In the above example, we obtain an Iterator using `myHashMap.entrySet().iterator()`, where `entrySet()` returns a set containing all of the key-value pairs in the HashMap. Then, we use a `while` loop to iterate through the entries using the `hasNext()` and `next()` methods of the Iterator. The `next()` method returns the next entry in the HashMap, which we can access using `getKey()` and `getValue()` methods to print the key-value pairs.
Using an Iterator allows us to have fine-grained control over the traversal of the HashMap and its entries. It is useful when we need to perform more complex operations on the key-value pairs or when you want to maintain a specific order during iteration, including insertion order in the case of LinkedHashMap.
Print a HashMap Using Custom Objects as Keys and Values
To print the contents of a HashMap using custom objects as keys and values, we need to implement a suitable `toString()` method. This method will define how the objects are represented as strings when printed using `System.out.println()` or any other printing mechanism, and provides a great deal more control as a result.
This is a bit more complex than the previous techniques, so we will divide our operation into two steps for added clarity.
Step 1: Create the Custom Objects
Let’s say that we have a custom class named `Person`, which serves as the key in the HashMap, and another custom class named `Address`, which serves as the corresponding value. The following example shows how we can create custom objects:
public class Person {
private String name;
// Other attributes, constructor, and methods
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
// Include other attributes in the string representation if needed
'}';
}
}
public class Address {
private String city;
private String zipCode;
// Other attributes, constructor, and methods
@Override
public String toString() {
return "Address{" +
"city='" + city + '\'' +
", zipCode='" + zipCode + '\'' +
// Include other attributes in the string representation if needed
'}';
}
}
Step 2: Create and Print the HashMap
Now we can create a HashMap with `Person` objects as keys and `Address` objects as values. When we print the HashMap, it will automatically use the `toString()` method of the custom objects to display their contents.
import java.util.HashMap;
public class Main {
public static void main(String[] args) {
HashMap<Person, Address> personAddressMap = new HashMap<>();
Person person1 = new Person("John");
Address address1 = new Address("New York", "10001");
Person person2 = new Person("Alice");
Address address2 = new Address("Los Angeles", "90001");
personAddressMap.put(person1, address1);
personAddressMap.put(person2, address2);
// Printing the HashMap using System.out.println()
System.out.println(personAddressMap);
}
}
Output:
{Person{name=’John’}=Address{city=’New York’, zipCode=’10001′}, Person{name=’Alice’}=Address{city=’Los Angeles’, zipCode=’90001′}}
As you can see, the `toString()` method in the `Person` and `Address` classes determines the custom string representation of the objects when printed in the HashMap. It allowed us to do things that weren’t possible in previous examples, like printing the city and postal code of each person.
Note that by overriding the `toString()` method in our custom classes, we can control how the objects are displayed in any context, not just when printing HashMaps.
Conclusion
Printing a HashMap in Java is a fundamental task that every Java developer should be familiar with. Throughout this article, we have explored various techniques and methods to achieve this task efficiently and effectively.
We began by introducing the HashMap, an essential data structure from the Java Collections Framework that allows us to store and manage key-value pairs efficiently. Understanding the underlying principles of HashMaps, such as hashing and constant-time complexity for optimal operations, forms the foundation for leveraging their power in various Java applications.
To print the contents of a HashMap, we explored several methods, each offering its advantages based on specific requirements. We first learned the simplest method, using the System.out.println() method. We learned how to extend this approach by iterating through the keys or values using `keySet()` or `values()` methods and then using the `get()` method to retrieve corresponding values. We utilized the `entrySet()` method to get a set of key-value pairs (Map.Entry objects) and iterate through them. The third approach utilized the `toString()` method directly, providing a concise and straightforward representation of the HashMap’s key-value pairs.
Following this, we delved into additional methods, such as `BiConsumer` and `Iterator`, to gain more control over the printing process. We saw that using `BiConsumer` allowed us to perform custom actions for each key-value pair, while `Iterator` gave us fine-grained control over traversal, especially in cases where order mattered.
Finally, we explored the usage of `Collections.singletonList()` and `Arrays.asList()` methods, although they might not be the most common approaches for printing HashMaps.
In conclusion, we’ve seen many different ways to print a HashMap in Java, each offering unique benefits and flexibility. As a Java developer, mastering these techniques empowers you to effectively present and manipulate key-value pairs, leading to more efficient code and improved problem-solving skills in various application scenarios.
Remember that choosing the appropriate method depends on your specific use case and the desired output format. Whether you prefer simplicity, custom formatting, or fine-grained control, we are hopeful that the techniques covered in this article provided you with the tools to successfully print HashMaps in Java. HashMaps are powerful data structures that are popular in programming as well as data science, and they are a popular source of questions in any system design interview.
We hope you’ve enjoyed this tutorial. Happy coding!