Java Data Types
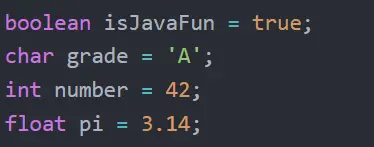
A data type is a classification of the value type of a variable, such as integer or character. They allow programmers to specify the characteristics needed to hold a specific type of value in order to help optimize the program. For example, primitive data types are size-optimized, which enables programs to be leaner and faster than they would otherwise be.
The Java programming language supports a number of data types, which can be categorized into two groups: primitive data types and reference data types. Primitive types store actual values, while reference types store the memory addresses of the objects that they refer to.
In Java, variables must be declared with their data types before they can be used. The appropriate choice of data type is essential because it determines the range of values the variable can hold and the operations that can be performed on it.
Primitive and Reference Data Types in Java
In Java, primitive data types represent simple values like numbers or characters. They are predefined and have a fixed size in memory.
Java has eight primitive data types: Boolean, char, byte, short, int, long, float, and double.
Of these eight primitive types, six are numeric. Only Boolean and char are designed to hold non-numeric values.
- Primitive Data Types:
Java has eight primitive data types that can represent simple values and numbers. These are:
- Boolean: The simplest data type, requiring only one bit. A Boolean represents a true or false value.
- char: A char holds a 16-bit Unicode character.
- byte: A byte stores an 8-bit integer value. It has a range of -128 to 127.
- short: The short has 16-bit integer value. It has a range of -32,768 to 32,767.
- int: An int holds a 32-bit integer value. It has a range of -2^31 to 2^31 – 1.
- long: Holds a 64-bit integer value. Range of -2^63 to 2^63 – 1.
- float: A float holds a 32-bit floating-point value.
- double: Holds a 64-bit floating-point value.
The following table summarizes the primitive data types in Java:
Data Type | Size | Stores |
---|---|---|
boolean | 1 bit | True (1) or false (0) values |
char | 2 bytes | A single character/letter, or ASCII values |
byte | 1 byte | Whole numbers from -128 to 127 |
short | 2 bytes | Whole numbers from -32,768 to 32,767 |
int | 4 bytes | Whole numbers from -2,147,483,648 to 2,147,483,647 |
long | 8 bytes | Whole numbers from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
float | 4 bytes | Fractional numbers, good for storing 6 – 7 decimal digits |
double | 8 bytes | Fractional numbers, good for storing up to 15 decimal digits |
In Java, we can specify the data type of a variable in the declaration:
boolean isJavaFun = true;
char grade = 'A';
int number = 42;
float pi = 3.14;
Reference Data Types
Reference data types are used to store references (memory addresses) to objects. The size of a reference type is unknown prior to initializing, which allows them to store various types of data much more flexibly than primitive data types.
For example, a string can be of a variable length: “Hello”, “World”, and “Hello, World!” are all valid examples of strings. It wouldn’t make sense to restrict the length of a string the way that we do with primitive types.
Reference data types in Java include strings, classes, interfaces, and arrays.
Here are some example declarations:
String text = "Hello, Java!";
int[] numbers = {1, 2, 3, 4, 5}; // Array of integers
Object obj = new Object(); // Object is a root class for all Java classes