Numbers in Java
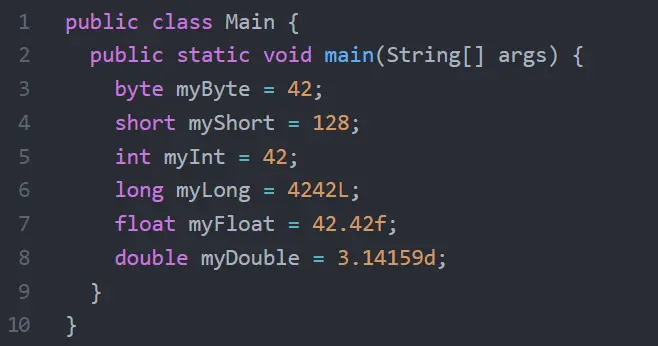
Java has several numeric data types that can be used to store and work with numbers. These data types are all considered to be primitive, although numbers can also be used in reference types like arrays.
There are six primitive numeric types in Java: byte, short, int, long, float, and double.
The following table summarizes the numeric data types in Java:
Data Type | Size | Stores |
---|---|---|
byte | 1 byte | Integers from -128 to 127 |
short | 2 bytes | Integers from -32,768 to 32,767 |
int | 4 bytes | Integers from -2,147,483,648 to 2,147,483,647 |
long | 8 bytes | Integers from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
float | 4 bytes | Floating-point numbers, good for storing 6 – 7 decimal digits |
double | 8 bytes | Floating-point numbers, good for storing up to 15 decimal digits |
In this tutorial, we will look at each of these data types, and explore how to optimize your Java program by choosing the right type for every case.
Integer Data Types
Java has four integer data types: byte, short, int and long. These data types are used to store integer values, meaning that they can only store whole numbers. They can be used to store positive or negative numbers.
Let’s look at each of the numeric data types:
Byte
The smallest data type for storing integers, a byte stores an 8-bit integer value. It has a range of -128 to 127.
public class Main {
public static void main(String[] args) {
byte myByte = 42;
System.out.println(myByte);
}
}
Output:
42
Note that we will get an error if we try to initialize a byte to a value above 127:
public class Main {
public static void main(String[] args) {
byte myByte = 128;
System.out.println(myByte);
}
}
Output:
error: incompatible types: possible lossy conversion from int to byte
To resolve this error, we need to use an integer type capable of holding larger values.
Short
A short is twice the size of a byte, and uses 16-bits to store an integer value. It has a range of -32,768 to 32,767.
public class Main {
public static void main(String[] args) {
short myShort = 128;
System.out.println(myShort);
}
}
Output:
128
Int
Often used by default, an int is twice the size of a short and holds a 32-bit integer value.
It has a range of -2^31 to 2^31 – 1, which corresponds to a range of -2147483648 to 2147483647.
public class Main {
public static void main(String[] args) {
int myInt = 42;
System.out.println(myInt);
}
}
Output:
42
Long
The long is twice the size of an int and holds a 64-bit integer value. It has a range of -2^63 to 2^63 – 1. The long data type is often indicated by appending an ‘L’ to the end of the number.
public class Main {
public static void main(String[] args) {
long myLong = 4242L;
System.out.println(myLong);
}
}
Output:
4242
Floating Point Data Types
Java has two floating point data types: float and double. They are used to store positive or negative floating point numbers, which contain a decimal point. Like the integer data types, they can be used to store positive or negative numbers.
Float
A float is the smallest data type used to hold a floating-point value, and is 32-bits. It is considered best practice to append an ‘f’ to the end of a floating-point number, which we can see in the example below.
public class Main {
public static void main(String[] args) {
float myFloat = 42f;
System.out.println(myFloat);
}
}
Output:
42.0
Note that a decimal point is automatically added to a floating point number, even if it wasn’t included in the initialized value of the variable.
Double
Also known as a ‘double-precision floating point number’, a double is twice the size of a float. It holds a 64-bit floating-point value. It is common to append a ‘d’ to the end of a double, which we can see in the example below.
public class Main {
public static void main(String[] args) {
double myDouble = 3.14159d;
System.out.println(myDouble);
}
}
Output:
3.14159
Scientific Notation
When working with very large or small numbers, it’s common to use scientific notation. Scientific notation involves using a decimal (floating-point) number and multiplying it with the value of 10 to an appropriate power.
For example, we can express the number 10,800 as 10.8 x 103, or .00045 as 4.5 x 10-4.
Java supports the use of scientific notation by using a floating-point number with the character E to denote ’10 to the power of’. For example, E04 means ’10 the the power of 4′, and E-03 means ’10 to the power of minus three’.
public class Main {
public static void main(String[] args) {
float myFloat = 42E3f;
double myDouble = 42E-3d;
System.out.println(myFloat);
System.out.println(myDouble);
}
}
Output:
42000.0
0.042
Optimizing Your Java Program by Choosing the Right Numeric Data Types
Choosing the appropriate numeric data type in Java is important for optimizing a program for several reasons:
- Memory Usage: Different numeric data types occupy different amounts of memory. Choosing the smallest data type that can hold the range of values you expect to work with helps conserve memory. This is particularly important in applications where memory usage is a critical factor, including mobile applications or systems with limited resources.
- Performance: Operations on smaller data types are typically faster than operations on larger data types. When you use smaller data types, the CPU can process more data in a single operation, leading to better performance. This is especially important in mathematical calculations and loops.
- Storage and Bandwidth: Smaller data types can reduce the storage requirements of your data structures, which can be beneficial when storing data. Smaller data types also reduce the bandwidth needed to transmit data, making network communication more efficient.
- Precision: Choosing the right floating-point data type (
float
ordouble
) is important for maintaining precision in mathematical calculations. Double-precision floating-point numbers (double
) provide higher precision than single-precision floating-point numbers (float
). If your application requires high precision, usingdouble
is essential to avoiding or reducing rounding errors in calculations. - Readability: Choosing meaningful and appropriate data types can help make your code more readable to other developers. The correct data type can help conveys the intent of the variable and helps prevent mistakes caused by using incorrect data types inadvertently.
In summary, careful consideration of the numeric data types in Java can lead to more memory-efficient, faster, and reliable programs. It’s important to balance the trade-offs between memory usage, performance, and precision based on the specific requirements of your application.