How to Use Exponents in Java: a Comprehensive Guide
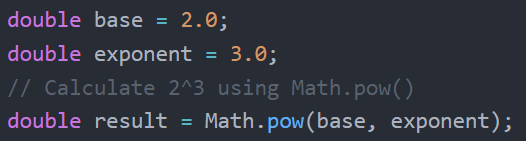
When it comes to Java programming, there are moments when we need to harness the power of mathematics to solve complex problems. Exponents, which represent repeated multiplication of a number by itself, are an important mathematical concept that we frequently see in various computational scenarios.
In the realm of Java programming, understanding how to effectively calculate exponents is not only a valuable skill, but is also a critical component in developing algorithms.
One of the things that can make it difficult to perform exponent calculations, is that Java doesn’t have an exponent operator. While other languages have native support for an exponent operator such as **, in Java we need to either use a function like Math.pow() or calculate the exponent manually. The Math.pow() function is easy to use and can calculate negative and fractional exponents as well.
This article aims to be your definitive guide to mastering the topic of exponents in Java. We’ll learn about:
- Math.pow(): We’ll explore the built-in `Math.pow()` function and learn how to leverage it for a variety of common exponentiation tasks.
- For Loops: Using for loops to compute exponents.
- While Loops: An alternative iterative approach using while loops to calculate exponents.
- Recursive Calls: Explore the power of recursion as we learn how to create recursive functions for exponentiation.
- Negative Exponents: We’ll tackle the challenge of calculating exponents with negative numbers, shedding light on the nuances of this operation.
- Fractional Exponents: Take a close look at how to calculate fractional exponents.
Whether you’re interested in optimizing your code, enhancing your problem-solving skills, or simply expanding your Java programming knowledge, this article will equip you with the tools and techniques to confidently handle exponents in the Java programming language. Let’s begin our journey into the world of exponents and uncover the secrets to unleashing their computational powers in your Java applications.
What Are Exponents?
While this isn’t an article on mathematics, it may be helpful to review what exponents are. This will give us a better understanding of the concept and allow us to compare them with and understand the use of exponents in Java.
An exponent, in mathematics, is a small number placed above and to the right of another number or expression, like 23.
In text, exponents are often represented using a carat, i.e. 2^3:
23 = 2^3 = 2 * 2 * 2 = 8
Either way, an exponent indicates how many times that number or expression should be multiplied by itself. The exponent is also referred to as a power. The exponentiation operation is known as getting the ‘power of a number’.
In its simplest form, when you have a number “a” raised to an exponent “n,” it’s written as “a^n.”
This means that we need to multiply “a” by itself “n” times. For example, 2^3 means that 2 is multiplied by itself 3 items:
- 21 = 2
- 22 = 2 × 2 = 4
- 23 = 2 × 2 × 2 = 8
- 24 = 2 × 2 × 2 × 2 = 16
More interesting things happen when an exponent isn’t an integer.
For example, a fractional exponent represents taking the root of a number. A fraction of 1/2 indicates a square root, while a fraction of 1/3 is a cube root.
- 41/2 = 2
- 81/3 = 2
- 161/4 = 2
A negative exponent means that we need to take the inverse (one over) of a number. For example, n^-1 = 1/n:
- 2-1 = 1/2
- 3-1 = 1/3
- 4-1 = 1/4
Using Exponents in Java
There are several common techniques for working with exponents in Java. We’ll cover the most important built-in method, Math.pow() as well as how to calculate the exponent of a number from scratch using loops and recursion.
Using Math.pow() to work with exponents in Java
Java’s `Math.pow()` function is a built-in method that allows us to calculate exponents.
It’s part of the `java.lang.Math` class, which provides a collection of helpful mathematical functions. The `Math.pow()` function specifically calculates the result of raising a base number to a specified exponent.
double Math.pow(double base, double exponent)
- base: This is the base number, also known as the base value.
- exponent: This is the power to which we are raising the base number.
For example, in the following line:
double Math.pow(2, 3)
Math.pow(2,3) is the same as 23. In other words, it calculates the value of the first argument to the power of the second argument.
The `Math.pow()` function returns a `double` type value, which means it’s suitable for both integer and non-integer exponents.
Here’s a practical example of how to use Math.pow():
public class ExponentExample {
public static void main(String[] args) {
double base = 2.0;
double exponent = 3.0;
// Calculate 2^3 using Math.pow()
double result = Math.pow(base, exponent);
System.out.println(base + "^" + exponent + " = " + result);
}
}
Output:
2.0^3.0 = 8.0
In this example, we calculate 2^3 using `Math.pow(2.0, 3.0)` and store the result in the `result` variable. The program then prints the return value, which is 8.0, indicating that 2 raised to the power of 3 is equal to 8.0.
We can use the `Math.pow()` function whenever we need to work with exponents. It’s a convenient and efficient way to calculate exponents, especially when dealing with non-integer or fractional powers.
Calculating Exponents Using a For Loop
We can use a for loop to calculate an exponent. This simple function allows us to calculate an exponent without the usage of any external functions or libraries.
The for loop in the example below will iterate ‘exponent’ number of times, each time multiplying the base by itself:
public class ExponentExample {
public static void main(String[] args) {
double base = 2.0;
double exponent = 4.0;
// Calculate 2^3 using Math.pow()
double result = 1;
for (int i = 0; i < exponent; i++) {
result *= base;
}
System.out.println(base + "^" + exponent + " = " + result);
}
}
Output:
4.0^2.0 = 16.0
We can see that our for loop has correctly performed the exponent calculation. On the first loop, it sets the result equal to 1 * 2.0 = 2.0. On the second iteration of the loop, it gets multiplied by the base again, becoming 2.0 * 2.0 = 4.0. On the third loop it equals 4.0 * 2.0 = 8.0. Finally on the fourth loop it becomes 8.0 * 2.0 = 16.0. The loop will not iterate again because the dummy variable i is no longer less than the exponent.
This loop method is cumbersome, but it allows us to calculate the exponent without relying on any external or pre-built functions and will work for simple cases.
Calculating Exponents Using a While Loop
A while loop can also be used to calculate an exponent.
public class ExponentExample {
public static void main(String[] args) {
double base = 4.0;
double exponent = 2.0;
// Calculate 2^3 using Math.pow()
double result = 1;
double count = 0;
while (count < exponent) {
result *= base;
count++;
}
System.out.println(base + "^" + exponent + " = " + result);
}
}
Output:
4.0^2.0 = 16.0
This simple example demonstrates how a while loop can be used to calculate an exponent.
Calculating Exponents Using Recursion
We can use recursion to calculate exponents by making the same function call itself repeatedly. This gives us a custom function that we can call whenever we want to calculate an exponent.
In the following example, we create a function called Exp() and use it to calculate an exponent value by recursively calling itself:
package exponent_example;
public class Exponent_example {
public static void main(String[] args) {
double base = 4;
int exponent = 2;
double result = Exp(base, exponent);
System.out.println(base + "^" + exponent + " = " + result);
}
public static double Exp(double base, double exponent) {
if (exponent <= 0)
return 1;
return base * Exp(base, exponent - 1);
}
}
Output:
4.0^2.0 = 16.0
Note that the Exp() function takes the base as the first parameter, and the exponent as the second parameter. It performs a recursive call on itself, resulting in the calculation of the exponent.
Calculating Negative Exponents in Java
A negative exponent means that we need to take the inverse of the number. For example, 2 to the power of -1 ( 2^-1 ) is equal to 1/2. The rule is,
a^-b = 1/(a^b)
Most of the above methods will not work for calculating negative exponents in Java. For this, it’s best to use a built-in function like Math.pow().
Using Math.pow(), we can calculate the value of negative exponents in the same way as we did with positive exponents:
public class ExponentExample {
public static void main(String[] args) {
double base = 4.0;
double exponent = -1.0;
// Calculate 2^3 using Math.pow()
double result = Math.pow(base, exponent);
System.out.println(base + "^" + exponent + " = " + result);
}
}
Output:
4.0^-1.0 = 0.25
Notice in the above program that the output is 0.25, because 4^-1 = 1/4 = .25
The Math.pow() function can be used not only to calculate negative exponents, but fractional exponents as well (and even negative fractional exponents!)
Calculating Fractional Exponents in Java
We can also use the Math.pow() function to calculate fractional exponents in Java.
A fractional exponent is the equivalent to taking the root of the number. For example, we can get the square root using an exponent of 1/2.
The following example program shows how Math.pow() can be used to calculate a fractional exponent:
public class ExponentExample {
public static void main(String[] args) {
double base = 4.0;
double exponent = .5;
// Calculate 2^3 using Math.pow()
double result = Math.pow(base, exponent);
System.out.println(base + "^" + exponent + " = " + result);
}
}
In this example we used Math.pow() to calculate a square root, but it’s important to recognize that an exponent can theoretically be of any value, including floating-point numbers.
Working With Exponents in Java
In this article, we’ve tackled the subject of exponents in Java, and we’ve seen several different methods for calculating exponents. We briefly covered exponents from a mathematical perspective before going on to discover multiple different ways for calculating exponents.
We began by introducing the powerful and versatile pow() function from the math library. Math.pow() allows us to easily calculate the power of any number without any additional code. Next, we saw how to manually calculate exponents using for loops, while loops, and recursion. These examples gave us a deeper look into the calculation of exponents while sharpening our skills. We saw that while these options gave us the ability to calculate exponents from scratch, they were also cumbersome and very limited.
In particular, using loops or recursion only allowed us to perform a calculation when the exponent is a positive integer. In special cases, such as if the exponent is negative, fractional, or otherwise complex term, then we will need to again turn to a simple and robust method like Math.pow(). In the last two sections of this tutorial, we returned to the Math.pow() function, this time learning how to use it to calculate the result of negative and fractional exponentiation. This feature allows Java to shine when it comes to complex mathematical operations involving exponents.
If you’re a Java developer or are looking for more Java tutorials, check out our main Java page for our complete course and list of articles! Or see our other tutorials on various programming languages!