What is a Java Instance Variable? With Examples
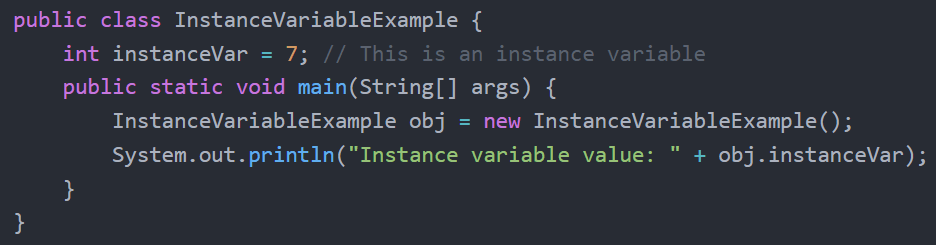
In the realm of Java programming, understanding the concepts that underlie the language’s core features is essential for writing robust and efficient code.
One important concept is that of instance variables, which plays a pivotal role in defining the characteristics and behavior of Java classes. Instance variables serve as containers for storing data that are unique to each object instance of a class. They are distinct from static and local variables, and they empower developers to create dynamic and interactive applications by encapsulating state information within objects.
In this article, we will explore the topic of Java instance variables, exploring their characteristics, usage, and why they are important. Through a series of illustrative examples, we will see how instance variables facilitate the creation of flexible and modular code structures.
Whether you’re a new programmer looking to learn the fundamentals of Java or an experienced developer trying to enhance your understanding, this exploration of instance variables will provide valuable insights into leveraging Java’s object-oriented principles for building powerful software solutions. Let’s embark on this journey to demystify the concept of instance variables and learn their practical applications in the Java programming landscape.
- Local, Instance, and Class/Static Variables in Java
- How to Use Instance Variables in Java
- Features of Instance Variables
- Limitations and Drawbacks of Instance Variables
- Table of Default Values of Instance Variables
- Local vs. Instance vs. Class/Static Variables in Java
- Overview of Variable Types in Java
- Conclusion – Java Instance Variables
Local, Instance, and Class/Static Variables in Java
One of the best ways to conceptually learn about instance variables is to see how they contrast with two other Java variable types: local variables and class/static variables.
Local Variables
Local variables are confined within specific method, constructor, or block of code.
As such, they are only accessible within that limited scope and are useful for temporary data storage and manipulation.
Local variables must be explicitly initialized before use, and are automatically destroyed when the scope they belong to is exited. They are inherently thread-safe because they are confined to their containing scope.
The following example demonstrates the use of a local variable:
public class LocalVariableExample {
public static void main(String[] args) {
int localVar = 10; // This is a local variable
System.out.println("Local variable value: " + localVar);
}
}
Output:
Local variable value: 10
In this example, localVar
is a local variable that is declared within the main
method. Because it is a local variable, it is accessible only within the scope of the main
method.
Static (Class) Variables
Static/class variables are shared among all instances of a class and are associated with the class itself, not with individual object instances.
Static variables are declared with the `static` keyword, and they are accessible across all methods and instances of the class. They have a single copy that exists for the entire program’s duration.
Static variables are often used for constants or data shared across all instances. Because they are not automatically destroyed, static variables are not inherently thread-safe and may require synchronization in multi-threaded environments.
public class ClassVariableExample {
static int classVar = 5; // This is a class (static) variable
public static void main(String[] args) {
System.out.println("Class variable value: " + classVar);
}
}
Output:
Class variable value: 5
In the above code, classVar
is a static variable declared within the ‘ClassVariableExample’ class.
It is associated with the class itself and can be accessed using the class name (ClassVariableExample.classVar
). All instances of the class share the same value for this class variable.
Instance Variables
Instance variables are associated with object instances of a class. They are declared within the class but outside methods or blocks.
Every object instance has its own set of instance variables, which allows objects to have distinct state and characteristics. In other words, the value of an instance variable is different for each instance.
Instance variables persist as long as the object exists, and can be accessed and modified by methods within the class. Like static variables, they are not inherently thread-safe, so synchronization may be required when accessed concurrently by multiple threads.
public class InstanceVariableExample {
int instanceVar = 7; // This is an instance variable
public static void main(String[] args) {
InstanceVariableExample obj = new InstanceVariableExample();
System.out.println("Instance variable value: " + obj.instanceVar);
}
}
Output:
Instance variable value: 7
In this example, instanceVar
is an instance variable declared within the ‘InstanceVariableExample’ class. It is associated with instances of the class, and it holds different values for different instances.
How to Use Instance Variables in Java
The following examples should help demonstrate how instance variables can be used within real applications. Later in this article, we will also see examples demonstrating instance variables vs. local and static variables.
There are many ways to work with instance variables, but we can assemble a simple outline that can be reconfigured and extended later on:
- Create the instance variable.
- Initialize the instance variable with a constructor.
- Create any methods we want to use to work with the instance variable; for example, getting or modifying its value.
- Create an instance of the class containing the instance variable.
- Finally, using the methods that we created to perform useful actions on or with the instance variable.
Instance Variable Example # 1:
Here’s a simple example of how to work with a Java instance variable. Note that we are performing a few different actions, similar to the outline above:
- Create the instance variable ‘color’, which stores the color of a Car object.
- Initialize the instance variable to ‘initialColor’ using a constructor.
- Create the ‘getColor()’ method to get the color.
- Create the ‘setColor()’ method to change the color.
- Create an instance ‘myCar’ containing the instance variable.
- Finally, we get and change the color of the ‘myCar’ object.
public class Car {
// Instance variable name 'color'
private String color;
// Constructor
public Car(String initialColor) {
color = initialColor; // Initialize the instance variable
}
// Method to get the color
public String getColor() {
return color;
}
// Method to change the color
public void setColor(String newColor) {
color = newColor;
}
public static void main(String[] args) {
Car myCar = new Car("Blue"); // Create an instance of the Car class
System.out.println("Initial color: " + myCar.getColor());
myCar.setColor("Red"); // Change the color
System.out.println("New color: " + myCar.getColor());
}
}
Output:
Initial color: Blue
New color: Red
In the above example, we can see all the basic steps required to work with instance variables.
The `Car` class has an instance variable named `color`, which stores the color of the car. The constructor initializes the `color` instance variable when a new `Car` object is created. The `getColor()` method retrieves the value of the `color` instance variable, and the `setColor()` method allows changing the value of the `color` instance variable. The `main()` method demonstrates creating a `Car` object, creating an instantiated object of the class. It then calls the methods for setting and getting its color.
Instance Variable Example # 2:
Let’s continue learning about Java instance variables by looking at another, slightly more complex example.
In this example, we will extend the code from example # 1 to demonstrate how instance variables store different values for different class instances.
In this case, we will be adding a second object to the ‘Car’ class, called yourCar. We’ll then call the getColor() and setColor() methods on both objects in order to demonstrate further how to work with methods and instance variables.
public class Car {
private String color;
public Car(String initialColor) {
color = initialColor;
}
public String getColor() {
return color;
}
public void setColor(String newColor) {
color = newColor;
}
public static void main(String[] args) {
Car myCar = new Car("Blue");
Car yourCar = new Car("Yellow");
System.out.println("Initial color of my car: " + myCar.getColor());
System.out.println("Initial color of your car: " + yourCar.getColor());
myCar.setColor("Red");
myCar.setColor("Black");
System.out.println("\nNew color of my car: " + myCar.getColor());
System.out.println("New color of your car: " + yourCar.getColor());
}
}
Output:
Initial color of my car: Blue
Initial color of your car: Yellow
New color of my car: Black
New color of your car: Yellow
The most important point from this example is that each object stores its own copy of the instance variable, which allows each object to store its’ own values and function in unique ways.
Now that we have seen a few examples of Java instance variables, let’s take an in-depth look at their features and limitations.
Features of Instance Variables
Instance variables in Java have distinctive features and characteristics that define their behavior within a class and its object instances:
1. Object-Specific: Instance variables are associated with individual object instances. Each object of a class has its own set of instance variables, allowing them to store and maintain unique state information.
2. Class Scope: Instance variables are declared within a class but outside any method or block. They can be accessed and modified by all methods within the class.
3. Lifetime: The lifetime of an instance variable matches the lifetime of the object it belongs to. An instance variable is created when an object is instantiated and persists until the object is no longer referenced and is garbage collected.
4. Initialization: Instance variables have default initial values (see table below) if not explicitly initialized. An instance variable gets initialized during object creation, either with explicit values in the constructor or through default initializers.
5. State Management: Instance variables help define the state of an object, and are therefore useful for state management. They store information that characterizes the object and helps it to behave in a specific way. For example, in a `Person` class, instance variables like `name`, `age`, and `address` can help define the state of each individual person object.
6. Accessibility: Instance variables are accessible from a class’s methods and constructors. They can also be accessed from outside the class using object references, as long as the access modifiers allow it.
7. Data Encapsulation: Instance variables can be protected to encapsulate data or made private. This restricts direct access from outside the class and enforces a high level of data integrity by controlling how it is accessed and modified.
9. Inheritance: The instance variables of a superclass are inherited by their subclasses. Depending on access modifiers (private, protected, default, public), subclass instances may directly access and modify inherited instance variables.
10. Dynamic Behavior: Instance variables contribute to the dynamic nature of Java objects. They allow objects to hold varying data even if they are instances of the same class.
11. Instance-Specific Methods: The behavior of instance-specific methods is often driven by instance variables. Instance-specific methods can operate on the instance variables to perform actions and computations unique to each object.
In summary, instance variables provide the means to define and manage the individual state of objects. Their uniqueness to each object instance allows for the creation of flexible and customized objects with distinct behavior and characteristics.
Limitations and Drawbacks of Instance Variables
While instance variables in Java offer many features and advantages, they also come with limitations and potential drawbacks that developers need to be aware of. These include:
1. Memory Overhead: Since each instance of a class holds its own copy of instance variables, memory usage can become a concern when dealing with a large number of objects. This can lead to increased memory consumption, particularly if the instance variables are storing large amounts of data.
2. Initialization Complexity: Instance variables must be initialized properly, either with explicit assignments in constructors or using default initializers. Failure to properly initialize instance variables can lead to unexpected behavior or runtime errors.
3. Inheritance Issues: Because subclasses inherit instance variables from a superclass, there may be instances where the subclass doesn’t need certain inherited instance variables, leading to unnecessary memory consumption or potential confusion.
4. Encapsulation and Access Control: While instance variables can be encapsulated by using access modifiers like `protected`, `private`, or package-private (default), this can limit the ability to access or modify them in certain contexts. It’s important to balance encapsulation and accessibility.
5. Thread Safety: Instance variables are not inherently thread-safe. If multiple threads access and modify the same instance variables concurrently, it can result in data inconsistencies as well as race conditions. Best practices include proper synchronization mechanisms to ensure thread safety.
6. Immutability and State Management: When instance variables are mutable, managing the state of objects can become complex. Uncontrolled changes to instance variables can lead to objects being in unexpected states.
7. Complex Object Initialization: Objects that have a large number of instance variables or that require complex initialization logic can lead to convoluted constructors and reduce code readability.
8. Garbage Collection: Instance variables can impact the behavior of garbage collection. Objects with longer lifetimes due to instance variables can cause memory leaks if not properly managed.
9. Serialization: Instance variables may need special handling when objects are serialized or deserialized, especially if they contain sensitive or transient data.
10. Limited to Object Instances: Instance variables are limited to object instances, which means that they can’t be accessed without an object reference. This may be a limitation in certain situations, particularly when dealing with purely static utility methods.
11. Design Complexity: Poorly chosen or designed instance variables can lead to inflexible code. Designing classes with well-chosen instance variables requires careful consideration of object responsibilities, encapsulation, and data modeling.
12. Maintenance Challenges: Changes to instance variables may require updates to the various parts of the code that use them. This can increase maintenance efforts and the potential for introducing errors.
Understanding these limitations and challenges helps developers make informed decisions when designing classes and utilizing instance variables. By addressing these concerns, developers can create more efficient, maintainable, and robust software systems.
Table of Default Values of Instance Variables
The following table shows the default values of instance variables in Java:
Instance Variable Data Type | Default Values |
---|---|
char | \u0000 |
boolean | false |
byte | (byte) 0 |
short | (short) 0 |
int | 0 |
double | 0.0d |
float | 0.0f |
long | 0L |
Object | null |
Local vs. Instance vs. Class/Static Variables in Java
If you’re still confused about the differences between local, instance, and static variables in Java, the following example should help.
Example Including Local, Instance, and Class Variables
The following code example includes all three variable types:
public class VariableExample {
static int classVar = 5; // Class (static) variable
int instanceVar = 10; // Instance variable
public void exampleMethod() {
int localVar = 15; // Local variable
System.out.println("Class variable: " + classVar);
System.out.println("Instance variable: " + instanceVar);
System.out.println("Local variable: " + localVar);
}
public static void main(String[] args) {
VariableExample obj = new VariableExample();
obj.exampleMethod();
}
}
Output:
Class variable: 5
Instance variable: 10
Local variable: 15
In this example, we have the following:
- A class (static) variable `classVar`, which is shared among all instances of the class and is accessed using the class name (`VariableExample.classVar`).
- An instance variable `instanceVar`, which holds different values for different instances of the class (i.e. different `VariableExample` objects).
- A local variable `localVar`, which is declared within the method `exampleMethod` and is only accessible within that method.
A Deeper Look at Instance vs. Local and Class Variables
For added clarity, let’s look at a deeper comparison of Java local variables, instance variables, and static (class) variables:
Local Variables:
- Scope: Local variables are declared within a method, constructor, or block of code. They are accessible only within the scope where they are declared. Once the scope is exited, the local variable can no longer be accessed.
- Lifetime: Local variables have a limited lifetime. They are created when the method/block is entered and are destroyed when the method/block exits.
- Usage: Local variables are used for temporary storage of data within a specific method or block. They help in managing and manipulating data locally without affecting other parts of the code.
- Initialization: Local variables must be explicitly initialized before they are used within the method or block where they are declared.
- Thread Safety: Local variables are inherently thread-safe since they are limited to the scope of a single method or block and are not shared among multiple threads.
Instance Variables:
- Scope: Instance variables are declared within a class, but outside any method, constructor, or block. They are associated with instances (objects) of the class and are accessible by the class’s methods and constructors.
- Lifetime: The lifetime of an instance variable matches the lifetime of the object they belong to. They are created when the object is instantiated and persist until the object is garbage collected.
- Usage: Instance variables store data that belongs to a specific object instance, not a method or class. They define the state of a specific object and its characteristics.
- Initialization: Instance variables can have default initial values (e.g., `null` for reference types, `0` for numeric types, `false` for booleans) if not explicitly initialized.
- Thread Safety: Instance variables are not inherently thread-safe. Proper synchronization is required if they are accessed or modified concurrently by multiple threads.
Static (Class) Variables:
- Scope: Static variables are declared with the `static` keyword, within a class but outside of any method or block. They are associated with the class itself rather than with instances of the class and are accessible throughout the class’s methods and constructors.
- Lifetime: Static variables have a lifetime that matches the entire duration of the program. They are created when the class is loaded and destroyed when the class is unloaded (typically when the program terminates).
- Usage: Static variables are shared among all of the instances of a class. They are often used to store data that is common to all objects of the class, such as constants or counters.
- Initialization: Static variables can have default initial values if not explicitly initialized and are initialized when the class is loaded.
- Thread Safety: Like instance variables, static variables are not inherently thread-safe; proper synchronization is required if they are to be accessed or modified concurrently by multiple threads.
Overview of Variable Types in Java
We’ve taken a deep look at instance variables and how they compare with local and static variables. To complete our understanding of instance variables, it can be helpful to see how they fit in among all the different types of Java variables.
The first three should be very familiar:
- Local Variables: Local variables are declared within a method, constructor, or a block of code and have limited scope. They are only accessible within the block where they are declared.
- Instance Variables (also called ‘Non-static Member Variables’): Instance variables are non-static variables declared within a class but outside any method, constructor, or block. They are associated with an instance of the class and each instance of the class has its own copy of these variables.
- Static Variables (Class Variables): Static variables are declared with the `static` keyword within a class, but outside any method or block. They are associated with the class itself, rather than with instances of the class. All instances of the class share the same static variable.
- Parameter Variables: These are variables that are passed into a constructor or method as parameters. They are used to receive values from the caller and are used within the method’s scope.
- Final Variables: Variables declared with the `final` keyword are constants and cannot be reassigned after their initial value is assigned. A final variable must be initialized when declared.
- Primitive Data Type Variables: As the name suggests, primitive data type variables store primitive types such as `int`, `double`, `char`, `boolean`, etc. They hold the actual values and are not objects. Java has eight primitive data types.
- Reference Variables: Reference variables are used to hold references to objects. They do not store the actual object data, but instead point to the memory location where the object is stored. Reference variables are used with non-primitive data types.
- Array Variables: Array variables are used to store arrays, which are collections of multiple values of the same data type.
- Local Class Variables: If a class is defined within a method, it can have its own variables. These variables are similar to local variables, and are only accessible within the class’s scope.
Hopefully this overview of the different types of variables in Java will help you to conceptually understand how instance variables fit into the overall landscape.
Conclusion – Java Instance Variables
In conclusion, a thorough understanding of instance variables in Java is indispensable for creating well-structured and efficient object-oriented programs. They empower us to encapsulate object-specific state and information, enabling the creation of dynamic and interactive applications.
Instance variables define the attributes and characteristics that make each object within a class distinct, contributing to the very essence of object-oriented programming. By encapsulating state within objects, instance variables give us the power to create modular, reusable, and highly maintainable code.
However, with these advantages come certain limitations and challenges that must be considered. Proper initialization, careful design, and thoughtful use of access modifiers are needed to harness the full potential of instance variables. Thread safety and memory management must also be addressed when multiple threads access the same variables concurrently or when dealing with a multitude of objects.
As we’ve seen, instance variables play a pivotal role in establishing the foundation of Java’s object-oriented paradigm. They allow us to build robust and flexible systems by encapsulating state, defining behavior, and supporting dynamic object interactions. We hope that with this knowledge, you will be well-equipped to navigate the intricacies of instance variables and forge elegant solutions that use their potential to the fullest extent.
If you enjoyed this Java tutorial, we invite you to check out our other Java content including a complete course on the Java programming language!