Printing Variables in Java
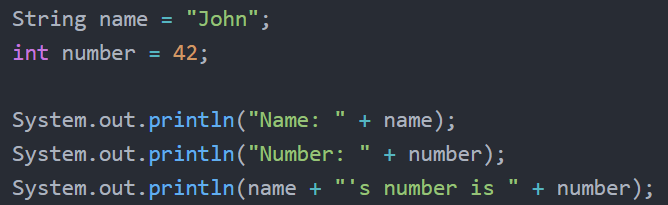
In Java, we can use methods such as System.out.print() and System.out.println() in order to print variables to the console.
These methods also give us the ability to work with variables in the print statement itself. This may seem trivial, but it allows us to print more complex statements without having to store additional variables or having unnecessary code.
Using println() To Print Variables in Java
In Java, we can use the System.out.println()
method to print variables to the console. We can do this by using the variable name inside the print statement (but outside any double quotes):
int myNum = 42;
System.out.println(myNum);
Output:
42
We can also combine the use of variables and text inside print statements. We can put text inside double quotes. The variables still live outside the double quotes.
For example:
public class HelloWorld{
public static void main(String []args){
String name = "John";
int number = 42;
System.out.println("Name: " + name);
System.out.println("Number: " + number);
System.out.println(name + "'s number is " + number);
}
}
Output:
Name: John
Number: 42
John’s number is 42
In this example, we created the variables ‘name’ and ‘number’, and then printed them using println().
Printing Expressions with println()
The System.out.println() method can be used to handle expressions.
For example, we can use println() to perform math using variables:
public class HelloWorld{
public static void main(String []args){
int x = 21;
int y = 2;
System.out.println("x: " + x);
System.out.println("y: " + y);
System.out.println("x * y: " + (x * y));
}
}
Output:
x: 21
y: 2
x * y: 42
In this example, we first create two variables, ‘x‘ and ‘y‘. They are both integers, which means that we can use math operators to perform different mathematical functions with them. After printing each of the variables, we use a third print statement to multiply them together. We can see that we were able to multiply them inside of the println() method.