How to Iterate Through a List in Python
The Python programming language gives us several excellent ways for iterating through a list.
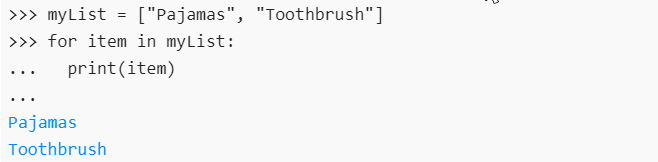
In Python, a list is a data type that is used to store multiple items in a single variable.
One of the best things about lists is that we can iterate through them. This means that we can easily perform the same function for every item in a list. Many common tasks require us to iterate through a list in Python, and there are several different ways to do this.
The Easiest Method to Iterate Through a List in Python
The most common, and simplest way to iterate through a list in Python is by using a ‘for’ loop. We’re putting this here at the top of this article because chances are, this is all you need:
for item in list:
print(item)
Example:
my_favorite_vegetables= [“Broccoli”, “Potatoes”, “Zucchini”]
for vegetable in my_favorite_vegetables:
print(vegetable)
Output:
Broccoli
Potatoes
Zucchini
Python List 101
As a brief review, a list is one of the built-in data types in the Python. Python’s data types include a text type (string ‘str’), numeric types (‘int’, float’, ‘complex’), Boolean type (‘bool’), and others. A list is one of Python’s three sequence types. The other two are ‘tuple’ and ‘range’.
A list is made up of ‘list items‘. List items are automatically indexed, with the first item in the list having an index of 0. The index value of each item depends on its’ location in the list. List items are also commonly called list elements.
Lists are one of Python’s mutable data structures. This means that lists can be modified after creation, which is a highly useful characteristic.
Create a List
We can easily create a new list list using square brackets:
my_favorite_vegetables = [“Broccoli”, “Potatoes”, “Zucchini”]
This creates a new list called ‘my_favorite_vegetables’ which is comprised of strings. We can also make lists with other data types such as integers or Booleans:
my_grades = [85, 93, 79, 87]
my_bools = [False, True, True]
Determine the Length of List
We can get the length of the list using the built-in len() function:
my_grades = [85, 93, 79, 87]
print(len(my_grades))
Output:
4
Using the Range Function to Create a List
If we need a list that consists of a sequence of numbers, we can quickly create one using the range function. For example if we want a list containing a sequence of integers from 1 to 10 we would use list(range(1, 11)):
int_sequence = list(range(1, 11))
print(int_sequence)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
If we wanted only the odd numbers, we could use the following code:
odd_numbers = list(range(1, 10, 2))
print(odd_numbers)
Output:
[1, 3, 5, 7, 9]
Accessing List Items
As mentioned, a specific element of the list can be accessed using the index number of the element within square brackets. For example, the first element in the list can be accessed using an index value of 0 inside square brackets [0]:
my_list = [“Pajamas”, “Toothbrush”, “Shampoo”]
print(my_list[0])
Output: Pajamas
To access the last item in the list (the item at the very end of the list), we can use the index value of -1:
my_list = [“Pajamas”, “Toothbrush”, “Shampoo”]
print(my_list[-1])
Output:
Shampoo
Now that we’ve reviewed a few ways to create lists and access list items, let’s learn how to iterate through them!
8 Easy Methods for Iterating Through a List in Python
In Python, lists are a type of iterable object. This means that we can perform a function for each item in the list, iterating over each of the elements of a list.
There are several different methods for iterating through a list in Python, and we will cover the essential ones here.
Method # 1: For Loop Method
The most common way to iterate is by using a for loop:
for item in list:
print(item)
Example:
my_favorite_vegetables= [“Broccoli”, “Potatoes”, “Zucchini”]
for vegetable in my_favorite_vegetables:
print(vegetable)
Output:
Broccoli
Potatoes
Zucchini
Most coders consider this to be the best, i.e. the most Pythonic, method.
Method # 2: Using Python List Comprehension
List comprehension provides a way of creating and working with lists using a shorter syntax. We can accomplish the previous task in a single line of code using the list comprehension method:
[print(item) for item in list]
Example:
my_favorite_vegetables= [“Broccoli”, “Potatoes”, “Zucchini”]
[print(vegetable) for vegetable in my_favorite_vegetables]
Output:
Broccoli
Potatoes
Zucchini
Method # 3: Using a While Loop
We can also use a while loop to iterate over each element in a list. To do so, we need a dummy variable (i) and use the len() function as in the below example:
i = 0
while i < len(list):
print(list[i])
i += 1
Example:
my_favorite_vegetables= [“Broccoli”, “Potatoes”, “Zucchini”]
i = 0
while i < len(my_favorite_vegetables):
print(my_favorite_vegetables[i])
i += 1
Output:
Broccoli
Potatoes
Zucchini
Method # 4: For Loop and range() function
We can also use a traditional for loop method. This method is not generally recommended since Python allows us to directly loop over the list using for.
for i in range(len(list)):
print(list[i])
Example:
my_favorite_vegetables= [“Broccoli”, “Potatoes”, “Zucchini”]
for i in range(len(my_favorite_vegetables)):
print(my_favorite_vegetables[i])
Method # 5: Using the Enumerate Function
The enumerate() function adds a counter to an iterable and returns it as an enumerating object. Using this method will return an iterable list of tuples:
for i, item in enumerate(list):
print(i, “,”, item)
Example:
my_favorite_vegetables= [“Broccoli”, “Potatoes”, “Zucchini”]
for i, vegetable in enumerate(my_favorite_vegetables):
print(i, vegetable)
Output:
(0, Broccoli)
(1, Potatoes)
(2, Zucchini)
Method # 7: Using the NumPy Library
When working with large, multi-dimensional lists, it can be better to work with an external library such as NumPy. NumPy stands for ‘Numerical Python extensions) and is designed expressly for working with multi-dimensional arrays and matrices.
import numpy as mynum
myArray = mynum.arrange(9)
myArray = myArray.reshape(3, 3)
for i in mynum.nditer(myArray):
print(i)
Output:
0
1
2
3
4
5
6
7
8
Method # 8: Using the Lambda Function
In the Python language, lambda is an anonymous function. An anonymous function is one that does not have a name.
The lambda function is commonly combined with the map function to iterate through a list. Map is a function that takes two values: a function and an iterable:
map(function, iterable)
It returns a list of results after applying the function to each item of the iterable.
When using the lambda function to iterate through a list in Python, we can pass the lambda function to map. This is a popular combination.
my_list = [3, 7, 12, 8]
list_squared = list(map(lambda x: x*2, li))
print(list_squared)
Output:
[9, 49, 144, 64]
In Summary: How to Iterate Through a List in Python
We have seen eight different methods for iterating through a list in Python. The easiest and most common method is the simple for loop, but there may be times when the other methods may be the right choice. For example, if we are working with large, multi-dimensional lists then we may want to use the NumPy library, and if we want to use an anonymous function than we may choose the lambda method.
For simple cases, the for loop (Method # 1) is considered best way because it is the most pythonic way.
If you’re looking for more information on lists and related topics, I definitely recommend looking through the official Python 3 documentation. This is a great place to go deeper into the Python programming language.