How to Trim or Strip a String in Python
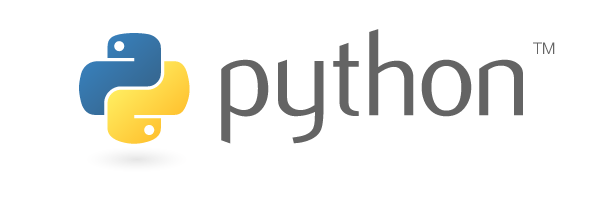
In Python, a string is a sequence of characters. It is a type of array, and can be manipulated in different ways in order to accomplish a variety of tasks.
To this end, Python has a variety of built-in functions that allow us to work with strings. A common task is removing the white spaces from a string. This can include whitespace at the beginning, end, or middle of the string.
In this tutorial we will cover:
- Using strip(), lstrip(), and rstrip() to trim whitespace from the beginning and/or end of a string
- Removing all whitespace from a string with replace()
- Replacing whitespace with another character or sequence or characters
- Removing duplicate whitespace in a string
- Using slice notation to remove the first or last characters of a string
- Types of Whitespace
- Removing Whitespace From Beginning or End of a String
- Remove Whitespace From Beginning of String
- Remove Whitespace From End of String
- Remove All Whitespace in a String
- Remove Duplicate Whitespace in a String
- Slicing the First or Last Characters of a String in Python
- Conclusion – How to Trim Whitespace From a String in Python
Types of Whitespace
Whitespace comes in three different forms:
- There can be whitespace at the beginning/left/start of the string. This is called leading whitespace.
- There may be whitespace at the end/right side of the string. This is called trailing whitespace.
- There may be whitespace in between words or character groupings.
Sometimes whitespace is useful but there are also occasions when we may want to remove any extra spaces from a string.
In Python, we have ways of eliminating or replacing the whitespace in each of these cases. We can use built-in methods to remove whitespace at the beginning and end of a string. We can even remove and replace any whitespace anywhere in the string including the spaces in between words.
Removing Whitespace From Beginning or End of a String
To start with, let’s look at the case where we want to remove the leading or trailing whitespace. Python has a built-in function to handle this: strip()
The strip function can be used to trim whitespace from the beginning and end of a string. strip() will look at both the beginning and the end of the string.
There are three Python strip functions: strip(), lstrip(), and rstrip(). If we want to eliminate whitespace only the beginning or end of the string, we will need to use lstrip() or rstrip(). More on this below.
Syntax of the Strip Function
string_name.strip([args])
- strip() – Removes whitespace before and after the string
- lstrip() – Removes leading whitespace (at the start of the string)
- rstrip() – Removes trailing whitespace (at the end of the string)
The strip() function will remove whitespace or specific characters from the beginning and end of a string. Each of these functions will also take an optional argument that it will look for instead of whitespace.
Using Strip() With No Argument
When we do not provide strip() with an argument, it will automatically target and remove whitespace before and after the main body of the string.
The string below has a number of spaces before and after the main body of text. We can use the strip() function to remove the whitespace at both ends of a string:
lorem = ” Lorem ipsum dolor sit amet “
print(lorem.strip())
Output:
Lorem ipsum dolor sit amet
Note that in the above output, the whitespace before and after the main string has been removed.
There are two things to note here:
- The leading whitespace before the word ‘Lorem’ and the trailing whitespace after the word ‘amet’ have both been removed.
- The whitespaces in between the words are still there.
Using Strip() With An Argument
If we give strip() an argument, then it will search for the character or character sequence that we specify. In the following example, we are targeting the letter ‘L’:
Single Character
lorem = “Lorem ipsum dolor sit amet”
print(lorem.strip(‘L’))
Output:
orem ipsum dolor sit amet
Note that in the output above, we have removed the letter ‘L’ from the word ‘Lorem’.
Character Sequence
The strip() function can also be used to remove a character sequence at the beginning or end of a string. In this example, we can remove the sequence ‘Lor’:
lorem = “Lorem ipsum dolor sit amet”
print(lorem.strip(‘Lor’))
Output:
em ipsum dolor sit amet
As we can see, this stripped the first three characters from the string.
These techniques work for special characters as well:
lorem = ‘Lorem ipsum!’
print(lorem.strip(‘!,L’))
Output:
orem ipsum
We can also specify multiple characters by separating them with a comma:
print(lorem.strip(‘L,m’))
Output:
orem ipsu
If we want strip() to look for whitespace as well as characters, we can simply use a space instead of a character in the argument.
Remove Whitespace From Beginning of String
To remove the leading whitespaces from the left side of the string we can use the lstrip() function.
lstrip() uses the same basic syntax as strip() but it only works on the leading spaces of the string. The strip() function, in contrast, will look for pattern matches on both the beginning and end of the string sequence.
We can use lstrip() without an argument in order to remove any white space characters on the left side of a string:
lorem = ‘ Lorem ipsum’
print(lorem.lstrip())
Output:
Lorem ipsum
When used with an argument, lstrip() will remove any leading characters that match the arguments provided:
lorem = ‘Lorem ipsum’
print(lorem.lstrip(‘L’))
Output:
orem ipsum
Remove Whitespace From End of String
The rstrip() function can be used to remove whitespace on the right side of the string. As with lstrip(), it uses the same basic syntax of the strip() function. When used without an argument, it will remove any trailing spaces from the given string:
lorem = ‘Lorem ipsum ‘
print(lorem.rstrip())
Output:
Lorem ipsum
We can also use rstrip() with an argument that it will look for at the end of the string.
lorem = ‘Lorem ipsum’
print(lorem.rstrip(m))
Output:
Lorem ipsu
How to Trim New Lines
The rstrip() function will remove trailing whitespace as well as the newline character \n.
Remove All Whitespace in a String
We can remove or remove and replace all of the whitespace in a string using the replace() function.
The replace() function will remove all whitespace including leading, trailing, and the whitespace between words.
lorem = ‘Lorem ipsum dolor sit amet’
print(lorem.replace(” “, “”))
Output:
Loremipsumdolorsitamet
In the above example, the replace() function is looking for any whitespace and then replacing it with no space (i.e. nothing). You can use other arguments in the replace() function. For example, we could replace whitespace with a character or replace one character for another.
Using replace() to Replace Whitespace
To replace whitespace with another character or set of characters we can use the replace() function with appropriate arguments. Let’s take the example of html encoding. Whitespace has a name code of in html. This allows whitespace to be interpreted correctly using html.
We can use replace() to replace all of our whitespace with the html name code:
lorem = ‘Lorem ipsum dolor sit amet’
lorem_html = lorem.replace(” “,” ”)
print(lorem_html)
Output:
Lorem ipsum dolor sit amet
Remove Duplicate Whitespace in a String
There are times when we may want to remove extra whitespaces from our string. The join() and split() functions can be used together to remove the duplicate whitespace and newline characters in a string. This method will retain a single space while eliminating the extra blank spaces.
lorem = ‘Lorem ipsum dolor sit amet’
corrected = ” “.join(lorem.split())
print(corrected)
Output:
Lorem ipsum dolor sit amet
Slicing the First or Last Characters of a String in Python
We can use string slicing to eliminate the first or last characters of a string in Python. In these cases, we will be creating a new string. We will need to use index notation, which uses square brackets:
string[start:stop]
For example, we can remove the first letter by designating that the slice start at the ‘1’ index, which is the second letter of the string:
lorem = ‘Lorem ipsum dolor sit amet’
slice = lorem[1:]
print(slice)
Output:
orem ipsum dolor sit amet
We could also slice off the last character by ending the slice at the -1 index location, which is the last character of the string:
lorem = ‘Lorem ipsum dolor sit amet’
slice = lorem[:-1]
print(slice)
Output:
orem ipsum dolor sit amet
Conclusion – How to Trim Whitespace From a String in Python
In this tutorial, we have seen multiple methods for removing whitespace from a string in Python.
- Use the strip(), lstrip(), and rstrip() functions to remove the leading or trailing whitespace or other characters.
- Remove or replace any of the whitespace (or any other character sequence) in a string
- Eliminate duplicate whitespace from a string
- Use slice notation to remove leading or trailing characters from a string