Go Constants
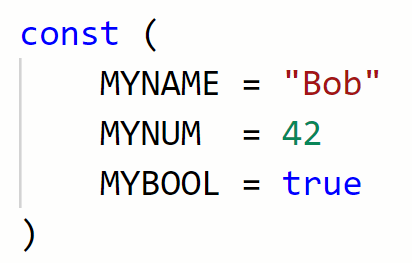
In Go, a constant is a name that is permanently paired with a value.
Constants are similar to variables but unlike variables, the value that is assigned to a constant cannot be changed. Another way of saying this is that constants are read-only.
Constants are declared using the const keyword:
const CONSTANTNAME type = value
Constants can be strings, Booleans, or numeric types (integers, floats, or complex numbers):
package main
import (
"fmt"
)
func main() {
const MYNAME = "Bob" // String constant
const MYNUM = 42 // Integer constant
fmt.Println("Hi,", MYNAME, "! Your number is: ", MYNUM)
}
Output:
Hi, Bob ! Your number is: 42
In this tutorial, we will cover the details of constants in Golang, including declaring (creating) constants, naming conventions, the two types of constants, properties of constants, and constants vs. variables.
Constant Naming Convention in Go
– Constant names are typically written in UPPERCASE to distinguish them from variables. However this is a personal choice and is not enforced or used by all Go developers.
– Constants names must technically abide by the rules of variable naming.
Types of Constants in Go
There are two types of constants in Go: typed constants and untyped constants.
Typed constants are declared with a specific data type.
Untyped constants are not declared with a specified data type, and the compiler is left to infer the data type of the constant based on the assigned value.
We’ll go into more details on both types of constants in the next sections.
Typed Constants
Typed constants are declared with the data type specified:
const CONSTNAME type = value
The following example shows how to declare string and integer typed constants:
package main
func main() {
const MYNAME string = "Bob"
const MYNUM int = 42
}
Untyped Constants
Untyped constants don’t have the data type specified. In this case, the compiler looks at the value assigned to the constant and infers the data type based on the value.
We’ve already seen untyped constants, in the first example given in this tutorial. Here’s a simplified look at string and integer untyped constants:
package main
func main() {
const MYNAME = "Bob"
const MYNUM = 42
}
Properties of Constants in Go
In Golang, constants are read-only and unchangeable. This is primarily what distinguishes them from variables.
This means that if we try to change the value assigned to a constant, the compiler will throw an error:
package main
func main() {
const MYNUM = 42
MYNUM = 21
}
Error:
./prog.go:5:2: cannot assign to MYNUM (untyped int constant 42)
Declaring Multiple Constants
Multiple constants can be declared at the same time by grouping them using parentheses. After declaration, each constant can be referred to individually:
package main
import (
"fmt"
)
const (
MYNAME = "Bob"
MYNUM = 42
MYBOOL = true
)
func main() {
fmt.Println(MYNAME)
fmt.Println(MYNUM)
fmt.Println(MYBOOL)
}
Output:
Bob 42 true
Constants vs. Variables in Go
It is best practice to use constants instead of variables when we know that the value will not need to be reassigned. This helps to prevent accidental reassignment (along with distinguishing between them using UPPERCASE).
Use a variable when the assigned value needs to (or might need to) be changed after the initial assignment.
The following provides a summary of variables vs. constants:
- Constants are always read-only and unchangeable, while variables can be reassigned.
- Constants are declared using const, while variables are declared using var.
- Variables can be declared using := but constants cannot.
- Constants and variables technically follow the same naming rules, but the most common convention is to name constants using UPPERCASE and to name variables using either camelCase or PascalCase. This helps to visually distinguish constants from variables.