Go Variables
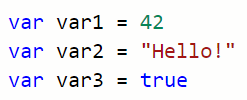
In the Go programming language, variables are used to store values of different types such as numbers, strings, or Booleans.
There are two ways to declare variables in Go: using the var keyword before the variable name or using a colon before the assignment operator ‘:=’.
var var1 = 42
var2 := true
These methods have some differences, which are covered in detail below.
Go requires either the data type or a value assignment in order for a variable to be declared. If a value is assigned then the data type can be inferred by the value. If a value isn’t assigned then the type needs to be specified (this only works when declaring using the var keyword).
In this article, we’ll cover the details of working with variables in golang.
Declaring Variables in Go
There are several different ways to declare variables in Go, and these methods have some subtleties differences between them.
Declare a Variable Using the var Keyword
The var keyword can be used to declare a variable without any details other than the name of the variable and the type:
var var1 int
We are declaring var1 as an integer type, so Go will automatically assign a value of integer 0 to var1 until we reassign it. We can declare other types such as strings and bools in a similar fashion:
func main() {
var var1 int
var var2 string
var var3 bool
fmt.Println(var1)
fmt.Println(var2)
fmt.Println(var3)
}
Output:
0
false
Note that the integer is automatically assigned a value of ‘0’, the string is empty (i.e. “”), and the Boolean is assigned a value of ‘false’.
Alternatively, if we assign a value then Go will be able to infer the type based on the value we provide:
var var1 = 42
var var2 = "Hello!"
var var3 = true
It is optional to specify the data type in this situation:
var var1 int = 42
var var2 string = "Hello!"
var var3 bool = true
Declare a Variable Using :=
The := operator can be used to declare and assign a value to a variable without using the var keyword.
var1 := 42
var2 := "Hello!"
var3 := true
This looks cleaner than using the var keyword but there are two limitations of this method:
- Assignment must occur during the declaration; there isn’t an option to declare and then assign later.
- Variables declared using := can only live inside functions. In contrast, variables declared using the var keyword can live inside or outside of functions.
var vs. :=
The following table covers the differences between using var and := when declaring a variable.
var | := |
Can be declared without assigning a value | Value must be assigned during declaration |
Type must be annotated if declared without assigning a value | Type inferred from assigned value |
Can be used inside or outside functions | Can only be used inside functions |
Variable Reassignment
Variables can be reassigned after initial assignment. In other words, the value assigned to a variable can be changed as many times as needed.
In the following example, we declare two variables: var1 and var2. We print both variables and then reassign and print them again:
func main() {
var1 := 21
var2 := "Hello"
fmt.Println(var1)
fmt.Println(var2)
var1 = 42
var2 = "Goodbye"
fmt.Println(var1)
fmt.Println(var2)
}
Output:
21
Hello
42
Goodbye
Variables can only be reassigned a value that matches the original type that was either explicitly declared or inferred during the declaration. For example, the following code throws an error:
var1 := 21
var1 = "Hello"
This code throws an error because we are trying to assign a string to a variable with an integer type.
Variables vs. Constants in Go
Unlike variables, the value assigned to a constant can’t be changed after assignment. It is considered best practice to use a variable when the assigned value needs to (or might need to) be changed after the initial assignment.
When we know that the value will not need to be reassigned (changed), it is best practice to use constants instead of variables. The following provides a summary of variables vs. constants:
- Variables can be reassigned, while constants are always read-only and unchangeable.
- Variables are declared using var, while constants are declared using const. .
- Constants cannot be declared using :=
- Variables and constants technically follow the same naming rules, but the most common convention is to name variables using either camelCase or PascalCase and to name constants using UPPERCASE. This helps to visually distinguish variables from constants.