Go Boolean Data Type
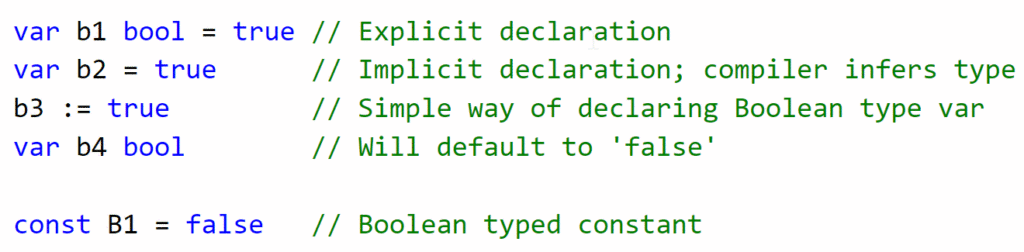
In Go, a Boolean data type can have a value of either true or false.
A Boolean data type can be declared using the bool keyword following the name of the variable or constant.
The default value of a Boolean data type in Go is false.
In this tutorial, we cover the details of Boolean typed variables in Golang, including several ways of declaring Boolean typed variables as well as Boolean typed constants.
Boolean Variables in Go
Variables can have a Boolean type. To declare a Boolean typed variable, we use the var keyword followed by the name of the variable, the bool keyword, and then the assignment:
var variableName bool = value
Let’s look at an example of Boolean variable declaration:
var bool1 bool = true
var bool2 bool = false
Since we are assigning a value of ‘true’ or ‘false’, we don’t need to specify the Boolean data type using the bool keyword in the declaration. The following works just as well:
var bool1 = true
var bool2 = false
Alternatively, we can declare the variable without assigning a value. In this case, we do need to annotate the type using the bool keyword:
var bool1 bool
var bool2 bool
In this example, we need to use the bool keyword because Go has no way of knowing (or inferring) the data type from the var keyword and variable name alone. Note that both bool1 and bool2 will have the default value of ‘false’.
Another option for declaring a Boolean value is to simplify the syntax using :=
bool1 := true
bool2 := false
This syntax is limited to variables; by using := we are declaring bool1 and bool2 to be variables, eliminating the need to use the var keyword.
Boolean Constants in Go
Like variables, constants can also have a Boolean type. Unlike variables, constants can’t be reassigned a new value once a value has been assigned.
Constants use the const keyword and are commonly named using UPPERCASE:
const B1 bool = true
const B2 bool = false
Summary of Boolean Data Types in Go
We’ve covered a variety of ways to declare Boolean variables and constants in Go. The following code summarizes what we’ve covered:
var b1 bool = true // Explicit declaration
var b2 = true // Implicit declaration; compiler infers type
b3 := true // Simple way of declaring Boolean type var
var b4 bool // Will default to 'false'
const B1 = false // Boolean typed constant