Go Strings

In the Go programming language, a string is a slice of bytes. You can think of a string as a data type comprised of a series of characters.
As a data type, strings are frequently found stored in variables, inside structs, and used with functions in a variety of ways. There are many helpful string methods – functions designed to work on a string object.
In this tutorial, we will go over the essentials of working with strings in Golang.
Creating a String in Go
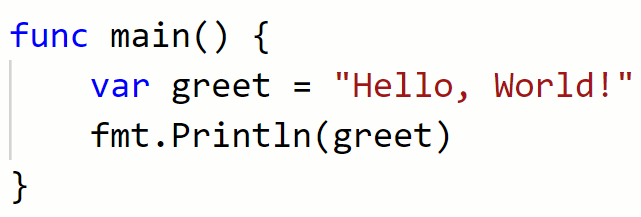
A string variable can be created by placing a series of characters inside double quotes:
var greet = "Hello, World!"
In this example, we used the var keyword to create a new string variable called ‘greet‘. The value of ‘greet’ is “Hello, World!”
Now that we know how to create a string, there are many useful things that we can do with it. Let’s start with how to print strings to the console.
Printing a String in Go
There are many ways of printing strings in Go. The simplest is to include the string directly in the print statement:
package main
import (
"fmt"
)
func main() {
fmt.Println("Hello, World!")
}
Output:
Hello, World!
We can also print a string variable by referring to it by name.
In the following example, we printed the string variable named ‘greet’ and printed it by passing greet into the fmt.Println() function:
package main
import (
"fmt"
)
func main() {
var greet = "Hello, World!"
fmt.Println(greet)
}
Output:
Hello, World!
These two methods of printing strings can be combined to print more complex statements to the console:
package main
import (
"fmt"
)
func main() {
var greet = "Hello, World!"
fmt.Println("String: ", greet)
}
Output:
String: Hello, World!
Getting the Length of a String
We can get the length of a string using the len() method. To use len(), we pass the string (or name of the string variable) inside the parentheses:
package main
import (
"fmt"
)
func main() {
var greet = "Hello, World!"
var length = len(greet)
fmt.Println("String: ", greet)
fmt.Println("Length: ", length)
}
Output:
String: Hello, World! Length: 13
Access the Characters of a String
The characters of a string can be accessed using an index number. The first character of the string has an index of ‘0’.
To print a character, we can use the fmt.Printf() function. We have to denote the location of the character with %c:
package main
import (
"fmt"
)
func main() {
var hello = "Hello, World!"
var mychar = hello[0]
fmt.Printf("String: %c", mychar)
}
Joining Strings Together
The easiest way to join strings together is by using the ‘+’ operator. This allows us to easily combine concatenate or combine strings in various ways:
package main
import (
"fmt"
)
func main() {
var hello = "Hello"
var world = "World!"
var combined = hello + ", " + world
fmt.Println(combined)
}
Output:
Hello, World!
The strings package contains lots of methods to help us work effectively with strings. One of these helpful methods is Join(), which allows us to concatenate strings together.
The Join() method works by concatenating the elements of an array. The first argument passed into Join() is the array, and the second is an optional separator:
package main
import (
"fmt"
"strings"
)
func main() {
var greet = []string{"Hello", "world!"}
fmt.Println(strings.Join(greet, " "))
}