How to Round Decimal Numbers in Java: double and float
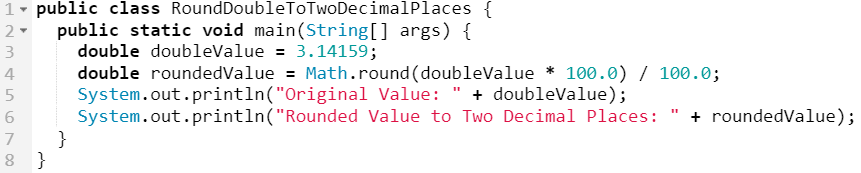
In Java, we can work with decimal numbers using the `float` and `double` data types. Both are designed for representing floating-point numbers, but they have some key similarities and differences.
Some of the most common tasks that can be performed with decimal numbers include rounding operations. This includes: a) rounding to an integer, and b) rounding to a specified number of decimal places.
In this article, we will learn several variations for rounding decimal numbers, including both float and double.
Before we dive into rounding operations, let’s compare and contrast `float` and `double` to help you understand how to work with decimals in Java:
Precision of float vs. double
- float: A float is a single-precision floating-point data type, which means it can represent decimal numbers with moderate precision. It uses 32 bits to store the value.
- double: A double-precision floating-point data type, offering better precision compared to `float`. It uses 64 bits to store the value, allowing it to represent decimal numbers with greater accuracy.
Range of float vs. double
- float: A float can represent a wide range of values, but it has a lower maximum value and less precision compared to `double`. It is suitable for many applications but may not be precise enough for tasks requiring high precision.
- double: A double has a larger range and can represent extremely large or small decimal numbers with great precision. It is commonly used for most floating-point calculations in Java.
Using float vs. double
- float: You may choose `float` when memory usage is a concern and the lower precision offered by float isn’t a concern. For example, in graphics programming or when dealing with large arrays of floating-point data.
- double: double is the standard choice for most applications because it provides a good balance of precision and range. It’s often used in scientific and engineering computations where precision is crucial.
Default Value of float and double
The default value for both `float` and `double` variables is `0.0`.
Literal Representation of float vs. double
- You can declare `float` variables by appending an ‘F’ or ‘f’ to the literal value. For example: `float myFloat = 3.14F;`
- double variables can be declared without any suffix or by appending ‘D’ or ‘d’ (although the suffix is optional). For example: `double myDouble = 3.14;`
Here’s an example illustrating the difference between `float` and `double`:
public class DecimalExample {
public static void main(String[] args) {
float floatValue = 3.14159F;
double doubleValue = 3.14159;
System.out.println("Float Value: " + floatValue);
System.out.println("Double Value: " + doubleValue);
}
}
Output:
Float Value: 3.14159
Double Value: 3.14159
In this example, `floatValue` and `doubleValue` represent the same decimal value, but keep in mind that `doubleValue` can hold a higher precision number due to its `double` data type.
In summary, when working with decimals in Java, choose the appropriate data type (`float` or `double`) based on your application’s requirements for precision and memory usage. In most cases, `double` is the preferred choice for general-purpose calculations.
How to Round a float or double
In Java, we can round a float or double in a few different ways. The most common would be to use a method from the Math class. The Math class contains the following methods, which we can use to round to an integer number:
- round() rounds to the nearest integer – either up or down using the ’round half up’ method
- floor() can be used to round down
- ceil() can be used to round up
We can see that these three methods correspond to three different rounding modes, which are helpful in a variety of situations. Here’s an example of how to use Math.round():
public class MathRoundExample {
public static void main(String[] args) {
double number1 = 15.49;
double number2 = 15.5;
// Using Math.round() to round numbers to the nearest integer
long roundedNumber1 = Math.round(number1);
long roundedNumber2 = Math.round(number2);
// Displaying the original numbers and the rounded numbers
System.out.println("Original number 1: " + number1);
System.out.println("Rounded number 1: " + roundedNumber1);
System.out.println("Original number 2: " + number2);
System.out.println("Rounded number 2: " + roundedNumber2);
}
}
Note that these methods will only round a floating point number to an integer value.
The rest of this article will be focused on rounding to a certain number of decimal places.
For more information on rounding using the Math class, check our our complete article on the topic. It covers the use of each of the three rounding methods in-depth and includes a deep-dive into rounding negative numbers!
Round a double to a Specified Number of Decimal Places
In Java, we can round a `float` or `double` to a specified number of decimal places using either the Math or DecimalFormat classes. Here’s how we can do it:
Using the Math class
We can round a double to a specified number of places using the Math class. There are actually several different ways that this can be done. The following example is relatively straightforward and makes use of the round() and pow() methods:
public class RoundFloatDoubleExample {
public static void main(String[] args) {
double doubleValue = 3.14159;
int decimalPlaces = 3; // Specify the number of decimal places you want
double roundedValue = Math.round(doubleValue * Math.pow(10, decimalPlaces)) / Math.pow(10, decimalPlaces);
System.out.println("Original Value: " + doubleValue);
System.out.println("Rounded Value: " + roundedValue);
}
}
Output:
Original Value: 3.14159
Rounded Value: 3.142
In this approach, we multiply the `doubleValue` by 10 raised to the power of the desired number of decimal places, round the result using `Math.round()`, and then divide it back by 10 raised to the same power to get the rounded value.
Using DecimalFormat
The DecimalFormat class can also be used to round to a specified number of decimal places:
import java.text.DecimalFormat;
public class RoundFloatDoubleExample {
public static void main(String[] args) {
double doubleValue = 3.14159;
int decimalPlaces = 3; // Specify the number of decimal places you want
DecimalFormat decimalFormat = new DecimalFormat("#." + "0".repeat(decimalPlaces));
String roundedValue = decimalFormat.format(doubleValue);
double roundedDouble = Double.parseDouble(roundedValue);
System.out.println("Original Value: " + doubleValue);
System.out.println("Rounded Value: " + roundedDouble);
}
}
Output:
Original Value: 3.14159
Rounded Value: 3.142
In this example, we first create a `DecimalFormat` object with a pattern that specifies the number of decimal places that we want. Then, we format the `doubleValue` using this pattern, limiting it to the desired number of decimal places. Finally, we parse the formatted string back into a `double` to obtain the rounded value.
Both of these approaches will round the `float` or `double` value to the specified number of decimal places. You can choose the one that best fits your coding style and requirements.
Round a Float to a Specified Number of Decimal Places
In Java, we can round a float to a specified number of decimal places using the `Math` class in a similar fashion to how we did this for doubles (just above). Here’s an example:
public class RoundFloatToDecimalPlaces {
public static void main(String[] args) {
float floatValue = 3.14159F;
int decimalPlaces = 3; // Number of decimal places you want
// Calculate the multiplier (e.g., 1000 for 3 decimal places)
float multiplier = (float) Math.pow(10, decimalPlaces);
// Multiply the float by the multiplier, round it, and then divide by the multiplier
float roundedValue = Math.round(floatValue * multiplier) / multiplier;
System.out.println("Original Value: " + floatValue);
System.out.println("Rounded Value to " + decimalPlaces + " decimal places: " + roundedValue);
}
}
Output:
Original Value: 3.14159
Rounded Value to 3 decimal places: 3.142
In this example:
- We have a float named `floatValue` that we want to round to a specified number of decimal places – 3 in this case.
- We calculate a multiplier by raising 10 to the power of the number of decimal places. The multiplier will help shift and round the digits properly.
- We then multiply the floatValue by the multiplier, which effectively moves the decimal point to the right by the specified number of decimal places. We then use the Math.round() method to round the result to the nearest whole number.
- Finally, we divided the rounded value by the multiplier to move the decimal point back to its original position, effectively rounding the `float` to the specified number of decimal places.
In this example, the result will be a float containing the number pi rounded to 2 decimal places. You can adjust the `decimalPlaces` variable to round to the desired number of decimal places.
Rounding a Float to Two Decimal Places
In the previous example, we saw how we can round a float to any number of decimal places.
However, one of the most common rounding operations involves rounding specifically to two decimal places. This is useful in many cases, including numbers that represent monetary amounts.
The following code example shows how we can round a float to two decimal places:
public class RoundFloatToDecimalPlaces {
public static void main(String[] args) {
float floatValue = 3.14159F;
int decimalPlaces = 2; // Number of decimal places you want
// Calculate the multiplier (100 for 2 decimal places)
float multiplier = (float) Math.pow(10, decimalPlaces);
// Multiply the float by the multiplier, round it, and then divide by the multiplier
float roundedValue = Math.round(floatValue * multiplier) / multiplier;
System.out.println("Original Value: " + floatValue);
System.out.println("Rounded Value to " + decimalPlaces + " decimal places: " + roundedValue);
}
}
Output:
Original Value: 3.14159
Rounded Value to 2 decimal places: 3.14
In this case, we simply adjusted the value of decimalPlaces to 2. Our code then took care of the rest.
If you don’t need the ability to change the number of decimal places, you can also simplify the code a bit:
public class RoundFloatToTwoDecimalPlaces {
public static void main(String[] args) {
float floatValue = 3.14159F;
float roundedValue = Math.round(floatValue * 100) / 100.0F;
System.out.println("Original Value: " + floatValue);
System.out.println("Rounded Value: " + roundedValue);
}
}
Output:
Original Value: 3.14159
Rounded Value: 3.14
This example is essentially the same as the previous one, but we have eliminated two variables by simply hardcoding the calculation directly into the roundedValue variable declaration.
Rounding a Double to Two Decimal Places
We can round a double to two decimal places using virtually identical code to the previous example. The following short Java program shows this:
public class RoundDoubleToTwoDecimalPlaces {
public static void main(String[] args) {
double doubleValue = 3.14159;
double roundedValue = Math.round(doubleValue * 100.0) / 100.0;
System.out.println("Original Value: " + doubleValue);
System.out.println("Rounded Value to Two Decimal Places: " + roundedValue);
}
}
Output:
Original Value: 3.14159
Rounded Value to Two Decimal Places: 3.14
As expected, the output shows the rounded double value of 3.14 – pi rounded to two decimal places.
Overview of Rounding double and float in Java
That’s all for this article.
Rounding of decimal or fractional numbers is a common task in programming, and it can be critical to either maintain a certain level of precision, or to round to a specified number of decimal places.
We’ve successfully learned how to round decimal numbers in Java using a few different techniques that can be applied to different situations.
We began our analysis by looking at the float and double data types – the two decimal number types in Java. We then saw how both the Math and DecimalFormat classes could be used to perform decimal-based rounding operations. Next, we took a deeper look at how the Math class can be used to round both floats and doubles to any specified decimal point.
The simple examples provided should be sufficient for many basic operations, and can be easily adjusted to different situations. Finally, we looked at the example of rounding a float or double number specifically to two decimal places. In this case, we essentially hardcoded the values used in the previous example. This is the best practice unless you need to be able to adjust the number of decimal places, because it eliminates two unnecessary variables and a few lines of code.
Keep in mind that the techniques shown here are extensions of the basic rounding methods offered by the Math class. You can use a different rounding method along with these techniques in order to get the precise values you require – for example, performing floor rounding to three decimal places or ceiling rounding to four decimal places.
We hope you’ve enjoyed this Java tutorial! Check out our many other free programming tutorials and courses, including our main course on the Java language!