Java Strings
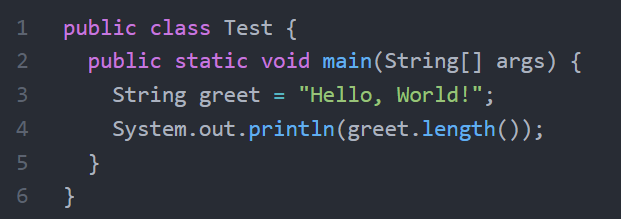
In Java, a string is an object that represents a sequence of characters. Strings are commonly used in Java programming for working with textual data.
Unlike some other programming languages, in Java, strings are objects. More specifically, they are instances of the ‘String
‘ class. Java provides a rich set of methods and operations for manipulating strings, and they fit well into Java’s object-oriented programming (OOP) paradigm.
Creating Strings in Java
In Java, strings can be created using either a string literal or by creating an instance of the String class using the new
keyword.
To create a string using a string literal, we can simply initialize the value of a string variable within double quotes:
String myStr = "Hello, World!";
In this example, we created a string variable called ‘myStr’, which holds the value “Hello, World!”
We can also create an instance of the String (java.lang.String) class using the ‘new’ keyword:
String str2 = new String("Hello, World!");
In most cases, these two types of strings perform similarly. They are both objects, and are both able to take advantage of the methods provided by Java. However they do have some differences, which will be covered in a future article.
In general, it’s often considered best practice to use the simpler method of creating a string literal. String literals are easier to read, and their use can improve performance as well.
Common Operations and Methods with Strings
There are several common operations and methods that allow us to work with strings with a lot of flexibility. These include: string concatenation, getting the length of a string, slicing out part of a string, accessing a character or substring by index, replacing a character or substring, splitting a string, and comparing two strings.
These common operations and methods will be covered in this section.
String Concatenation
Strings can be concatenated using the +
operator or the concat()
method.
To use the ‘+’ operator, we simply place the operator between two strings. It’s important to pay attention to any required spacing as we do this:
String firstName = "John";
String lastName = "Doe";
String fullName = firstName + " " + lastName;
We can also use the concat() method to concatenate two strings. In the following example, we use this method twice: once to add a space (” “) and once to concatenate lastName.
String firstName = "John";
String lastName = "Doe";
String greeting = firstName.concat(" ").concat(lastName);
Get the Length of a String
The length()
method returns the length (number of characters) of a string:
public class Test {
public static void main(String[] args) {
String greet = "Hello, World!";
System.out.println(greet.length());
}
}
Output:
13
In this example, we used the length()
method to get the length of the ‘greet’ string inside the print statement.
Accessing a Character of a String
Any character in a string can be accessed using the charAt()
method:
public class Test {
public static void main(String[] args) {
String greeting = "Hello, World!";
System.out.println(greeting.charAt(7));
}
}
Output:
W
Getting the Index of a Character or Substring
The indexOf()
method returns the index of the first occurrence of a character or substring in a string.
When we want to find the index of a character, we place the character inside single quotes (‘ ‘), e.g. string.indexOf('A')
. The indexOf()
method returns the first occurrence of that character in the string:
public class Test {
public static void main(String[] args) {
String greeting = "Hello, World!";
System.out.println(greeting.indexOf('W'));
}
}
Output:
7
When given an argument of a string, we need to place the string inside double quotes, e.g. string.indexOf("hello")
. In this case, the indexOf()
method returns the index of the first character in the first occurrence of the string:
public class Test {
public static void main(String[] args) {
String greeting = "Hello, World!";
System.out.println(greeting.indexOf("or"));
}
}
Output:
8
Slicing a Substring From a String
The substring(beginIndex, endIndex)
method extracts a portion of a string based on index.
In order to use the substring()
method, we must provide the starting and ending indexes. The first character of a string has an index of ‘0’.
public class Test {
public static void main(String[] args) {
String greeting = "Hello, World!";
System.out.println(greeting.substring(7,12));
}
}
Output:
World
We can also combine the substring()
method with the length()
method in order to slice to the end of the string:
public class Test {
public static void main(String[] args) {
String greeting = "Hello, World!";
System.out.println(greeting.substring(7,greeting.length()));
}
}
Output:
World!
Replacing Characters or Substrings
The replace(oldChar, newChar)
or replace(oldStr, newStr)
methods can be used to replace characters or substrings within a string:
public class Test {
public static void main(String[] args) {
String hello = "Hello, World!";
String goodbye = hello.replace("Hello", "Goodbye");
System.out.println(goodbye);
}
}
Output:
Goodbye, World!
Splitting Strings
The split(delimiter)
method splits the string into an array of substrings based on the given delimiter:
public class Test {
public static void main(String[] args) {
String greeting = "Hello, World!";
String[] words = greeting.split(" ");
System.out.println(words[0]);
System.out.println(words[1]);
}
}
Output:
Hello,
World!
In this example, we created an array of strings called ‘words’. Then we accessed the individual elements of the array by index, printing them one at a time.
Comparing Two Strings
Strings can be compared using the equals()
method for content equality or compareTo()
method for lexicographical comparison.
The equals() method will return true only if two strings are identical:
public class Test {
public static void main(String[] args) {
String str1 = "hello";
String str2 = "Hello";
boolean isEqual = str1.equals(str2);
System.out.println(isEqual);
}
}
Output:
false
In this case, str1 and str2 are not equal because the first letter of str1 is not capitalized while the first letter of str2 is.
We can also perform lexigraphical comparison using the compareTo() method.
public class Test {
public static void main(String[] args) {
String str1 = "hello";
String str2 = "Hello";
int comparisonResult = str1.compareTo(str2);
System.out.println(comparisonResult);
}
}
Output:
32
These are just a few examples of common string operations and methods in Java.
The String
class provides many more methods for various string manipulations, making it a versatile and powerful tool for working with text in Java programs.