Declaring Multiple Variables in Java
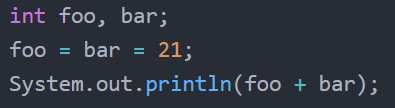
In Java, we can use a comma-separated list to simultaneously declare multiple variables of the same type.
This can make it easy to declare sets of variables, reduces the size of our code and simplifies it.
Declaring Multiple Values Without Initializing
We can declare multiple variables of the same type on a single line without initializing them.
The following example demonstrates this:
public class Main {
public static void main(String[] args) {
int foo, bar;
}
}
In this example, we have declared two integer variables foo and bar. We’ve left them uninitialized for now, but we will need to do so at some point before attempting to call them.
Declaring and Initializing Multiple Variables
We can also declare and initialize variables of the same type on a single line using the assignment ‘=’ operator:
public class Main {
public static void main(String[] args) {
int foo = 12, bar = 30;
System.out.println(foo + bar);
}
}
Output:
42
In this case, we’ve successfully initialized foo to a value of 12 and bar to a value of 30. We then printed the sum of foo and bar to the console using System.out.println().
Declare and Initialize Multiple Variables to the Same Value
If we have multiple variables of the same type that need to be initialized to the same value, we can declare them all on one line and then initialize them all on a separate line:
public class Main {
public static void main(String[] args) {
int foo, bar;
foo = bar = 21;
System.out.println(foo + bar);
}
}
Output:
42