How to Convert a String Into BigDecimal in Java
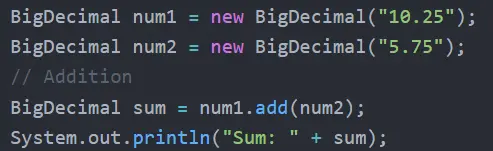
BigDecimal is a class designed for working with large decimal numbers, and it provides a number of helpful methods for performing precise numerical calculations. One common task that programmers face when working with BigDecimal is String to BigDecimal conversion.
One reason is that strings can be used with no loss of precision and no rounding errors. Another common reason is that we may be working with data that is most easily parsed in as String type. We may be able to streamline our code by converting a compatible String directly to BigDecimal.
No matter the reason, this article will help you convert a String to BigDecimal using a few different techniques and essential methods. Along the way, we’ll learn learn more about the BigDecimal class, using BigDecimal constructors, and the general process for converting a variety of data types to BigDecimal.
What is BigDecimal?
BigDecimal is a Java class that provides arbitrary-precision, signed decimal numbers. Part of the `java.math` package, BigDecimal is particularly useful when precise calculations are required.
The `BigDecimal` class allows us to perform arithmetic operations with numbers that have a large number of digits beyond the decimal point, without losing precision.
In other words, the ‘BigDecimal’ class is a data type optimized for working with large decimal numbers.
This is in contrast with primitive data types like `double` or `float`, which have limited precision and can more easily lead to rounding errors.
In order to use BigDecimal, we need to import it:
import java.math.BigDecimal;
Features of BigDecimal
Some key features of the `BigDecimal` class include arbitrary precision, no loss of precision, immutability, and the string constructor. Let’s deconstruct these.
Arbitrary precision means that `BigDecimal` objects can represent numbers with a virtually unlimited number of digits after the decimal point. This feature makes it suitable for applications where precision is crucial.
No loss of precision: Unlike floating-point arithmetic, `BigDecimal` operations are not subject to rounding errors.
Instances of the `BigDecimal` class are immutable, meaning the numeric value cannot be changed once they are created. Operations on `BigDecimal` objects create new objects rather than modifying existing ones.
The string constructor allows `BigDecimal` to be constructed using a `String` representation, which helps in avoiding inaccuracies that may arise when representing decimal numbers using floating-point literals.
BigDecimal Example
Here is a simple example of using `BigDecimal` to perform basic arithmetic operations:
import java.math.BigDecimal;
public class BigDecimalExample {
public static void main(String[] args) {
BigDecimal num1 = new BigDecimal("10.25");
BigDecimal num2 = new BigDecimal("5.75");
// Addition
BigDecimal sum = num1.add(num2);
System.out.println("Sum: " + sum);
// Subtraction
BigDecimal difference = num1.subtract(num2);
System.out.println("Difference: " + difference);
// Multiplication
BigDecimal product = num1.multiply(num2);
System.out.println("Product: " + product);
// Division
BigDecimal quotient = num1.divide(num2, 2, BigDecimal.ROUND_HALF_UP);
System.out.println("Quotient: " + quotient);
}
}
Output:
Sum: 16.00
Difference: 4.50
Product: 58.9375
Quotient: 1.78
In the above program, `BigDecimal` is used to perform basic arithmetic operations, and the `String` constructor is employed to initialize the `BigDecimal` objects with precise values. The `divide` method is used with a specified scale and rounding mode for division.
BigDecimal Constructors
Constructors are used to initialize BigDecimal objects using different data types. For example, BigDecimal objects can be initialized with a value of ‘long’ or ‘double’.
BigDecimal ‘long’ Constructor
public BigDecimal(long val)
BigDecimal ‘double’ Constructor
public BigDecimal(double val)
However, both of these constructors allow the possibility of rounding errors.
In contrast, the ‘String’ constructor prevents any loss of precision, even when working with a large number of decimal digits.
The BigDecimal String Constructor
The String constructor is a type of BigDecimal constructor that allows us initialize a BigDecimal object using a String. We can use the ‘String’ constructor by passing a string argument to BigDecimal():
public BigDecimal(String val)
Notice that the value passed into BigDecimal is a string. Let’s see an example of this:
public BigDecimal("1234.56789")
Note that the string “1234.56789” will be the initial value of the BigDecimal, and even though it is a string, it adheres to standard numerical representation (i.e. it is a valid number). BigDecimal can represent both positive and negative numbers.
This technique allows for the exact conversion of the value specified in the String.
Let’s look at a complete example so that we can see how BigDecimal ‘String’ constructors can be used in Java applications:
import java.math.BigDecimal;
public class BigDecimalStringConstructorExample {
public static void main(String[] args) {
// Using the String constructor to create BigDecimal objects
BigDecimal decimal1 = new BigDecimal("123.456");
BigDecimal decimal2 = new BigDecimal("-987.654");
BigDecimal decimal3 = new BigDecimal("0.12345678901234567890");
// Displaying the BigDecimal objects
System.out.println("decimal1: " + decimal1);
System.out.println("decimal2: " + decimal2);
System.out.println("decimal3: " + decimal3);
}
}
Output:
decimal1: 123.456
decimal2: -987.654
decimal3: 0.12345678901234567890
Other Methods for String to BigDecimal Conversion
In most cases, using the String constructor is the best solution when it comes to converting BigDecimal to String. However there are a few other methods we can deploy, including using the BigDecimal.valueOf() method.
Using the BigDecimal.valueOf() Method
The BigDecimal.valueOf() method can be used to convert a String to BigDecimal. This entails a two-step process:
- Converting the String to a numeric data type, such as ‘double’ or ‘long’. There are a number of methods we can use to convert a String to a numeric data type. For example, we can use Long.valueOf() or Double.valueOf() to convert a string to long, or double, respectively. However there are many other ways to do this as well.
- Converting the numeric data type to a BigDecimal using the BigDecimal.valueOf() method.
Note that using this technique may result in a loss of precision. We may lose digits when we convert from String to a numeric type.
The following example demonstrates String to BigDecimal conversion using Double.parseDouble() method to convert from String to double and BigDecimal.valueOf() to convert from double to BigDecimal:
import java.math.BigDecimal;
public class Main {
public static void main(String[] args) {
// Step 1: Convert String to double
String stringValue = "123.456";
double doubleValue = Double.parseDouble(stringValue);
// Step 2: Convert double to BigDecimal using BigDecimal.valueOf()
BigDecimal decimalValue = BigDecimal.valueOf(doubleValue);
// Display the results
System.out.println("String value: " + stringValue);
System.out.println("Double value: " + doubleValue);
System.out.println("BigDecimal value: " + decimalValue);
}
}
Output:
String value: 123.456
Double value: 123.456
BigDecimal value: 123.456