Golang Arrays
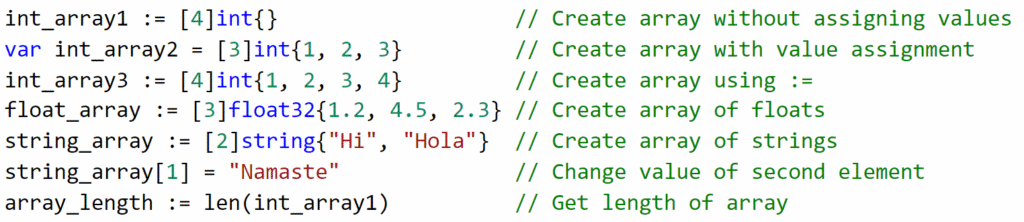
In the Go programming language, arrays are a collection of data of the same type. Arrays are used to store multiple values of the same type within a single variable.
Arrays are incredibly useful, and are one of the most important data structures in any programming language.
In Go, arrays are mutable – meaning that the values within the array can be changed after they are defined. However, even mutable arrays have a fixed length, meaning that the array cannot be shortened, lengthened, or have values ‘pushed’ or ‘popped’ off the end.
In this article, we will cover all of the essentials for working with arrays in Go.
What is An Array in Go?
You can think of an array in Go as a sequence of elements that all have the same data type, contained within square brackets. The following are all examples of valid arrays in Go:
Array of integers: [1, 2, 3, 42]
Array of floats: [5.2, 42.42, 3.14159]
Array of strings: [“Hi”, “Hola”, “Ciao”, “Namaste”]
Note that in each case, the array consists of a sequence of elements enclosed between square brackets. Each array only contains elements of the same type.
The first step of working with an array is to initialize, aka create, aka declare it.
Golang Create an Array
In Go, we have many options for creating an array, including:
- Using the var keyword or the := operator.
- By setting values for the entire array, none of the array, or part of the array.
Create an Array Using the Var Keyword
We can create an array using the var keyword followed by the name of the array.
We need to specify the number of elements (i.e. the array length) between square brackets on the right side of the ‘=’ operator, followed by the data type and then the values themselves between curly braces:
var array_name = [length]type{value1, value2, value3}
Create an Array Using the := Operator
We can also create an array without using the var keyword, by using the := operator:
array_name := [length]type{value1, value2, value3}
Let’s see a few examples of how this looks by creating arrays of different types.
Golang Create an Array of Numbers
We can create an array of numbers by specifying the type of the numbers, such as int, float32, or float64.
var int_array1 = [5]int{1,2,3,4,5}
int_array2 := [6]int{1,2,3,4,5,6}
Here’s how this looks in a working example:
package main
import (
"fmt"
)
func main() {
var int_array1 = [5]int{1, 2, 3, 4, 5}
int_array2 := [6]int{1, 2, 3, 4, 5, 6}
fmt.Println(int_array1)
fmt.Println(int_array2)
}
Output:
[1 2 3 4 5] [1 2 3 4 5 6]
To create an array of floats, we need to specify either float32 or float64:
package main
import (
"fmt"
)
func main() {
float_array := [4]float32{1.2, 4.5, 2.3, 8.6}
fmt.Println(float_array)
}
Output:
[1.2 4.5 2.3 8.6]
Golang Create an Array of Strings
We can create an array of strings using the string keyword:
package main
import (
"fmt"
)
func main() {
str_array := [4]string{"Hi", "Hola", "Ciao", "Namaste"}
fmt.Println(str_array)
}
Output:
[Hi Hola Ciao Namaste]
Initializing an Array Without Assigning Values
There are times when we need to initialize an array without assigning any values, or while only assigning some values. This can be done by not specifying values within the curly braces.
Initializing an Empty Array
Note that for an array of integers, the values default to 0 while for an array of strings, the values are empty (i.e. an array of empty strings):
package main
import (
"fmt"
)
func main() {
int_array := [4]int{} // Initializing array without assigning values
string_array := [5]string{}
fmt.Println(int_array)
fmt.Println(string_array)
}
Output:
[0 0 0 0] [ ]
Partially Initializing an Array
We can partially initialize an array by specifying values for the first elements. The rest of the array will be set to the default values for the type.
In the example below, we create an array of integers with a length of four, but we only specify values for the first two elements. The last two elements default to zero. We also create an array of strings with a length of five, and specify values for only the first three elements:
package main
import (
"fmt"
)
func main() {
int_array := [4]int{1, 2}
string_array := [5]string{"Hi", "Hola", "Namaste"}
fmt.Println(int_array)
fmt.Println(string_array)
}
Output:
[1 2 0 0] [Hi Hola Namaste ]
Initialize Specific Elements of an Array
We can initialize an array while setting values only for specific elements by specifying the element using its’ index number and a colon, followed by the value.
Note that the first element of an array has an index of zero. In the following example, we are specifying only the values of the second and third elements, leaving the first and fourth elements zeroed:
package main
import (
"fmt"
)
func main() {
int_array := [4]int{1: 21, 2: 42}
fmt.Println(int_array)
}
Output:
[0 21 42 0]
We will learn more about working with individual elements using index numbers in the next section.
Accessing the Elements of An Array
The individual elements of an array can be accessed using their index number. The first element of an array has an index of 0, the second element has an index of 1, and the last element has an index of [length – 1].
In the following example, we are getting the third element of an array of integers and an array of strings. Note that in order to retrieve the third element, we need to use an index value of ‘2’:
package main
import (
"fmt"
)
func main() {
int_array := [6]int{1, 2, 3, 4, 5, 6}
string_array := [5]string{"Hi", "Hola", "Namaste"}
fmt.Println(int_array[2])
fmt.Println(string_array[2])
}
Output:
3 Namaste
Changing the Elements of an Array
Arrays in Go are mutable, meaning that the values of their elements can be changed. We can change the value of an element using its’ index number.
In the following example, we change the value of the third element of both an integer array and a string array:
package main
import (
"fmt"
)
func main() {
int_array := [5]int{1, 2, 3, 4, 5}
fmt.Println("Int value before changing:", int_array[2])
int_array[2] = 42
fmt.Println("Int value after changing:", int_array[2])
string_array := [3]string{"Hi", "Hola", "Namaste"}
fmt.Println("String value before changing: " + string_array[2])
string_array[2] = "Ciao"
fmt.Println("String value after changing: " + string_array[2])
}
Output:
Int value before changing: 3 Int value after changing: 42 String value before changing: Namaste String value after changing: Ciao
Once again, note that in order to change the third element we have to access it using an index of ‘2’.
Getting the Length of an Array
We can use the len() function to get the length of an array. This is useful in a variety of situations, like looping or accessing elements at the end of the array.
package main
import (
"fmt"
)
func main() {
int_array := [5]int{1, 2, 3, 4, 5}
fmt.Println("Array length:", len(int_array))
}
Output:
Array length: 5
Accessing the Last Element of an Array
We can use the array length to access the last element of an array by subtracting one from the length, i.e. (len(array) -1).
The element with an index of [len(array) -1] will always be the last element in the array. This can be helpful in a variety of situations.
package main
import (
"fmt"
)
func main() {
int_array := [5]int{1, 2, 3, 4, 5}
fmt.Println("The last element is:", int_array[len(int_array)-1])
}
Output:
The last element is: 5