Hello, World! in Rust
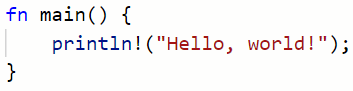
The best way to get started in Rust is to create a Hello, World! program.
The Hello, World! program allows us to confirm that Rust is properly installed and functioning. We’ll get some exposure to basic Rust syntax and also practice compiling and running programs written in Rust.
Create a Project Directory
Good practice is always to start a new project by creating a new directory. In this case, we are creating a directory named ‘rust-projects’:
mkdir hello-world
cd hello-world
Create a Rust Source Code File
Next, we need to create a Rust source file called main.rs
We can do this using the touch command (New-Item in Powershell):
touch main.rs
Paste the following code into the main.rs file:
fn main() {
println!("Hello, world!");
}
This code uses fn to define the main() function. Then it uses println! (a macro) to print out the string “Hello, World!”
If this is fuzzy or unclear, don’t worry! We’ll go over the code in more detail below.
Compile the Source Code
Use rustc to compile main.rs:
rustc main.rs
This will compile main.rs into an executable file called ‘main’ (without the .rs extension).
Run The “Hello, World!” Program
Now we can run main:
./main
You should see the message “Hello, World!” printed in the console.

Rust Basics With “Hello, World!”
Although the code seems short and a bit trivial, we can learn a great deal from the “Hello, World!” program.
Let’s take another look at the code:
fn main() {
println!("Hello, world!");
}
Although the entirety of the code is a singly, short function, we can see the basic structure of a Rust program and syntax.
The first and third lines show us the basic format of a function definition:
fn main() {}
We can see that we are using fn to declare the function, which is called ‘main’. The main body of the function is between curly braces {}.
println!("Hello, world!");
The second line starts with four spaces. Rust uses a four space indentation rather than a tab. The line ends with a semicolon ‘;‘. This tells Rust that the expression on the line is complete and that it should continue to the next line.
The program uses the println! macro to print the string “Hello, world!” to the console. Macros in Rust are indicated by an exclamation point. The string is passed as an argument into the println! macro, which then prints the string directly to the console.