Rust Vectors
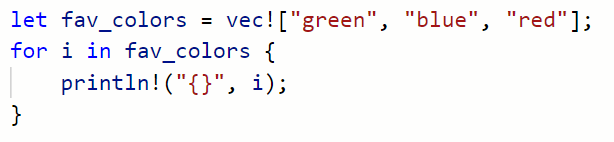
In Rust, vectors are a resizable, compound data type whose elements are of the same data type.
Vectors are similar to arrays but can grow or shrink in size.
Creating a Vector
There are two ways to create a vector: using the vec! macro or Vec::new().
Create a Vector Using vec! Macro
To create a vector, we use the vec! macro.
let my_vector = vec![<element 1>, <element 2>, <element 3>];
For example, we can create a vector that contains our favorite colors:
let fav_colors = vec!["green", "blue", "red"];
Note that the colors are strings so they are placed in double quotes.
Create a Vector Using Vec::new() Method
We can use the Vec::new() method to create a new, empty vector:
let my_vector = Vec::new()
This vector is empty, so we will need to add values to it for the compiler to be happy.
Vector Mutability
Vectors are immutable by default but can be made mutable using the mut keyword:
let mut fav_colors = vec!["green", "blue", "red"];
Rust won’t allow creation of a mutable vector unless the vector actually gets changed in the code. There are several methods such as push() and pop() that allow us to manipulate vectors in various ways.
Accessing Vector Elements
Vector elements can be accessed via index number or by using the get() method.
Access Vector Element by Index
Elements of a vector can be accessed by index number. The first vector element is assigned the index ‘0’.
let fav_colors = vec!["green", "blue", "red"];
println!("{}", fav_colors[0]);
Standard Output:
green
Access Vector Elements Using get() Method
Vector elements can be accessed using the get() method. To use get(), we must still specify the index of the element that we are getting.
let fav_colors: Vec<&str> = vec!["green", "blue", "red"];
println!("{:?}", fav_colors.get(0));
Standard Output:
Some("green")
This method doesn’t just give us the value of the element; it places the value inside an Option, which can have the value of Some or None.
To extract the actual vector element, we would need to write code that evaluates the Option.
Printing a Vector
The Rust compiler will throw an error if you try to print a vector using only its’ name. To print an entire vector, we can use either the debug trait or a loop.
Printing a Vector With Debug Trait
To use the debug trait, we can use a colon and question mark inside the curly braces:
println!(“Printing the whole vector: {😕}”, my_vector)
For example:
let fav_colors = vec!["green", "blue", "red"];
println!("{:?}", fav_colors);
Standard Output:
["green", "blue", "red"]
Note that this prints the whole vector; if we only want to access a single element, we can use that element’s index.
Printing a Vector Using a Loop
We can print an entire vector using a for loop to iterate over each element:
for i in my_vector {}
Note that ‘i’ here is the actual element, not the index value of the element.
For example:
let fav_colors = vec!["green", "blue", "red"];
for i in fav_colors {
println!("{}", i);
}
Standard Output:
green
blue
red