Rust Logical Operators
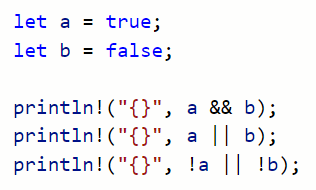
In the Rust programming language, logical operators are symbols that are used to perform logic operations.
There are three logical operators in Rust: AND, OR, and NOT.
Logical operators are designed to work with Boolean data types. They are a type of operator that receives a Boolean input and produces a Boolean output. This means that they operate on and produce true/false values.
Operator | Operation | Syntax | Function |
&& | AND | a && b | True if both operands are true |
|| | OR | a || b | True if at least one operand is true |
! | NOT | ! | Switches true to false or false to true |
AND Operator
The AND operator is ‘&&‘. It evaluates two Boolean inputs and outputs true only if both operands are true.
AND Operator Truth Table
The following truth table shows the possible results of the AND operator:
Operand A | Operand B | A && B |
True | True | True |
True | False | False |
False | True | False |
False | False | False |
AND Operator Example
let a = true;
let b = false;
println!("{}", a && b);
Standard Output:
false
OR Operator
The OR operator is ‘||‘. It evaluates two Boolean inputs and outputs true if at least one operand is true.
OR Operator Truth Table
The following truth table shows the possible results of the OR operator:
Operand A | Operand B | A || B |
True | True | True |
True | False | True |
False | True | True |
False | False | False |
OR Operator Example
let a = true;
let b = false;
println!("{}", a || b);
Standard Output:
true
NOT Operator
The NOT operator is ‘!‘. It is a unary operator, meaning that it acts on a single operand; it has one input and one output. NOT evaluates a single Boolean input and negates its value. A value of true will become false, and a value of false will become true.
NOT Operator Truth Table
The following truth table shows the possible results of the NOT operator:
Operand A | !A |
True | True |
False | False |
NOT Operator Example
let a = true;
println!("{}", !a);
Standard Output:
false
Lazy Boolean Expressions
In Rust, the first operand of an AND or an OR expression is evaluated first.
If the first AND operand is false, there is no need for the other to be evaluated because the expression will always be false.
Similarly, if the first operand in an OR expression is true, the whole expression will always be true.
Rust takes advantage of this by using short-circuit evaluation to reduce computational demand. The AND and OR expressions are considered ‘lazy’ because the second operand is only evaluated after the first.