Rust Characters
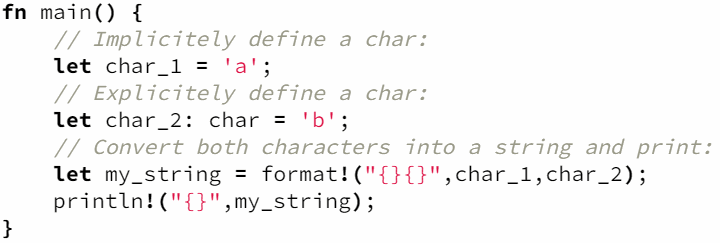
In the Rust programming language, the char
type is used to represent a single Unicode scalar value. A char
value is a 32-bit integer that can be used to represent any Unicode scalar value, including emoji and other symbols.
Here is an example of how to declare a char
variable in Rust:
let c: char = 'A'
In this example, the c
variable is declared as a char
. It is initialized with the value 'A'
. Note that the char
type is specified using the colon ‘:' char
syntax, and that char
values are written using single quotes.
Rust’s char
type is versatile and can be used to represent a wide range of characters and symbols. For example, the following code shows how to declare and initialize some common char
values:
let exclamation_mark: char = '!';
let emoji: char = '😀';
let newline: char = '\n';
In this code, the exclamation_mark
variable is initialized with an exclamation mark, the emoji
variable is initialized with a smiling face emoji, and the newline
variable is initialized with a newline character.
Overall, the char
type in Rust is a useful and powerful tool for working with character data. It allows developers to represent and manipulate characters and symbols in a safe and efficient manner.
Rust Character Creation
Variables with char type can be created by explicit or implicit definition. Explicit definition involves specifying the data type, while implicit definition allows Rust to define the type for us.
Implicit Definition of a char
In Rust, we can implicitly define a char
variable with the let
keyword followed by the variable name and the =
symbol. For example:
let ch = 'a';
This defines a char
variable named ch
with the value 'a'
.
Keep in mind that char
variables in Rust must be surrounded by single quotes ('
), not double quotes ("
) like strings. Additionally, char
variables can only hold a single character, not multiple characters like a string.
Explicit Definition of a char
In Rust, you can explicitly define a char
variable by specifying a char
type. For example:
let ch: char = 'a';
Note that this is almost identical to the example of implicit definition, but here the type is specified after the variable name using a colon: ‘ch: char‘.
This defines a char
variable named ch
with the value 'a'
.
By explicitly defining the type of the variable, you are telling the Rust compiler that you want the variable to be a char
type and not any other type. This can be useful if you want to make sure that your code is type-safe and that the variable you are defining will only accept values of the char
type.
Rust Char Type Casting
In general, Rust does not allow us to easily convert between the char type and other types. However there are a few useful operations that we can perform, which will be covered below.
Character to Integer in Rust
In Rust, we can convert a char
type to an int
type using the as
keyword to perform type casting.
Type casting allows us to convert a variable of one type into another type. For example, the following will convert a char variable with a value of ‘a’ into an integer:
let ch = 'a';
let _num = ch as u32;
println!("{}", _num); // Prints '97'
This will convert the char
value 'a'
to the int
value 97
. We can see that this method has a serious limitation: it returns the Unicode code point (the Unicode value) of the character, not the original value.
We can also use the to_digit()
method to convert a char
to an int
, but this method will only work for characters that represent decimal digits (0-9). For example:
let ch = '5';
let num = ch.to_digit(10);
This will convert the char
value '5'
to the int
value 5
.
Overall, it is important to carefully consider which method of conversion is most appropriate for your specific needs when working with char
and int
types in Rust.
Rust Char to String
We can convert a char
type variable to a String
with the to_string()
method. For example:
let ch = 'a';
let s = ch.to_string();
This will convert the char
value 'a'
to the String
value "a"
. This method returns a String
variable that contains the same character as the original char
value.
One clever method to convert a char into a string is by using the format!()
macro:
let my_char = 's';
let s = format!("{}", my_char);
This will convert the char
value 's'
to the String
value "s"
.