Rust Arrays
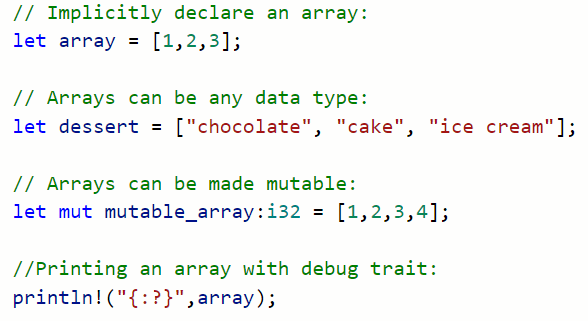
In the Rust programming language, arrays are a compound data type made of a sequence of elements of the same type. Arrays can be made mutable, enabling us to change the values of elements in the array. However, the length and type of an array (even a mutable array) can never be changed.
Arrays are similar to tuples. A tuple is also a compound data type, but its’ elements can have mixed data types (strings, booleans, etc). In contrast, every element of an array has the same type.
Arrays are also similar to vectors, but arrays always have a fixed length whereas vectors can be added to or have elements removed.
Like variables and tuples, arrays are declared using the let keyword and can be made mutable using mut.
To define an array, we can either specify the data type and size explicitly, or we can let the compiler figure out these details using values that we provide:
let my_array1:[i32;3]; // Declaring an array w/explicit annotation
let my_array2 = [1,2,3]; // Letting the compiler determine size/type
In the above example, we declared two arrays. They both contain elements that are of type i32 (32 bit integers), and have a length of 3.
Arrays are a powerful type in Rust, and there are many helpful operations that we can perform using arrays. In this article, we will cover the essentials of using arrays in Rust.
Declaring an Array in Rust
We can define an array using the let keyword. Values are assigned using a square bracket notation:
let my_array:[i32;3] = [1,2,3];
Alternatively, we can assign values at a later time:
let my_array:[i32;3];
my_array = [1,2,3];
Implicitly Define an Array
Arrays can be defined implicitly, which means that we aren’t required to specify the data type. For example:
let my_array = [1,2,3];
Rust will automatically create the same array that we explicitly declared above.
Implicit definition can also be used to automatically create and define arrays. Note the semicolon syntax used here:
let my_array = [2 ; 5];
This creates an array of 5 elements, each of which have a value of ‘2’:
fn main() {
let my_array = [2 ; 5];
println!("{:?}",my_array);
}
Standard Output:
[2, 2, 2, 2, 2]
Array Indexing in Rust
Array elements can be accessed via index number.
As with other programming languages, indexing in Rust starts at 0. In other words, the first element has an index of zero, the second element has an index of one, and the last element has an index of n-1 where n is the length of the array.
To access the first element in an array, we can use a square bracket notation and an index value of zero:
my_array[0]; // Gets the first element of 'my_array'
We can access the other elements of the array in the same fashion:
let my_array:[i32;3] = [1,2,3];
my_array[0]; // Equal to '1'
my_array[1]; // Equal to '2'
my_array[2]; // Equal to '3'
Printing an Array
Printing a Single Array Element
We can print a single array element using the index of the element:
let my_array = [1,2,3];
println!("{}",my_array[0]); // Prints the first element in the array; outputs '1'
Printing Multiple Array Elements
We can use standard indexing notation to print multiple elements, just as we would multiple variables:
let my_array = ["Bob", "blue"];
println!("My name is {} and my favorite color is {}.",my_array[0],my_array[1]);
Standard Output:
My name is Bob and my favorite color is blue.
Printing a Full Array
We can use the debug trait {:?} to print a full array:
let my_array = [1,2,3];
println!("{:?}",my_array);
Standard Output:
[1, 2, 3]
Mutable Arrays in Rust
Arrays are immutable by default, which means that once values are assigned to the elements of the array, they cannot be reassigned. Like variables, arrays can be made mutable using mut:
let mut my_array:[i32;3]; // Declaring a mutable array
This allows us to change element values after they have been defined:
// Declare and print a mutable array:
let mut my_array = [1,2,3];
println!("{:?}",my_array);
// Change array element values:
my_array = [2,3,4];
println!("{:?}",my_array);
Standard Output:
[1, 2, 3]
[2, 3, 4]
However, this does not allow us to increase the length of the array or change the data type:
// Declare an array of length 3:
let mut my_array = [1,2,3];
println!("{:?}",my_array);
// Attempt to assign four values to the array:
my_array = [1,2,3,4];
println!("{:?}",my_array);
Standard Error:
expected an array with a fixed size of 3 elements, found one with 4 elements
Array Length
Array length is the number of elements that the array contains.
We can get the length of an array using the len() function. We can use dot notation:
my_array.len();
To print the length of an array, we can call len() in the println!() macro argument:
let my_array = [1,2,3];
println!("Length of array: {}", my_array.len());
Standard Output:
Length of array: 3
Rust Array Slicing
Arrays slicing allows us to retrieve part of the array.
Slicing in Rust requires a two word object: a pointer and the slice index/length.
In the following example, we are creating a slice of an array called ‘slice’. We use a pointer (&array) to access the array (named ‘array’). Then we specify the slice using square brackets using the notation [starting_index..ending_index]:
let array = [1,2,3,4];
let slice: &[i32] = &array[1..3];
println!("{:?}",slice);
We can see that by referencing &array[1..3] we have obtained a slice of array from index 1 through index 3.
Pretty Printing an Array
Up to this point, we have been using the debug trait ‘:?’ to print an array.
We also have the option to ‘pretty print’ an array using ‘:#?’ inside the curly braces in our println! statements. This will print each element of the array on a new line.
The following example provides a direct comparison between these two methods:
fn main() {
let my_array = [1,2,3];
println!("{:?}",my_array);
println!("{:#?}",my_array);
}
Standard Output:
[1, 2, 3]
[
1,
2,
3,
]