Rust Functions in Modules
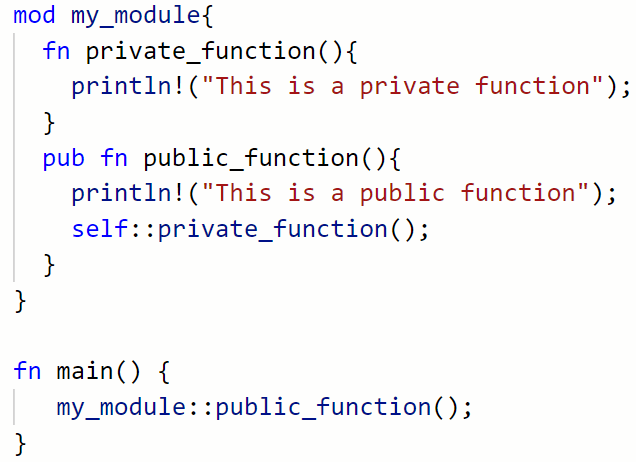
In Rust, we can control the visibility of functions in modules using the pub keyword.
The pub keyword can be used to control the privacy of both functions and modules. An entire module is private by default and can be made public using the pub keyword.
However, it is also possible to keep a module private but allow a function inside the module to be public. Additionally, we can access private functions with public functions within the same module. These features allows us finer control of visibility when dealing with modules and the functions that live inside them.
mod my_module {
pub fn print_hello_world(){
println!("Hello, World!");
}
}
fn main() {
my_module::print_hello_world();
}
Standard Output:
Hello, World!
The key point here is that we are able to call the print_hello_world() function from main() even though my_module is private. This is because print_hello_world() is public.
Accessing a Private Function Using a Public Function
Private functions would be limited in value if we couldn’t access them at all from outside of the module.
To expand the capabilities of private functions, we can call them using a public function that lives in the same module. This keeps the code inside the private function from being accessible outside the module, but allows it to be useful nonetheless.
The following example contains a module (my_module) with two functions: private_function() and public_function(). As these names suggest, private_function() is private while public_function() is public. But public_function() can access private_function() because they live in the same module.
mod my_module{
fn private_function(){
println!("This is a private function");
}
pub fn public_function(){
println!("This is a public function");
self::private_function();
}
}
fn main() {
my_module::public_function();
}
Standard Output:
This is a public function
This is a private function