Rust Enums and Match
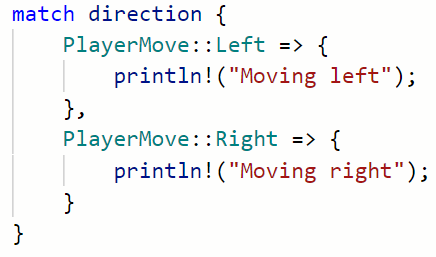
In the Rust programming language, enums are commonly used with match expressions to execute unique code based on the variant of an enum instance.
Enums and match expressions work exceptionally well together because the list of patterns in a match expression can be set to the possible variants of an enum.
Enums are comprised of a number of possible variants, and match expressions check if a value corresponds with a pattern in a list of patterns. When the patterns in the match statement are associated with the variants of an enum, a powerful pairing is created.
Let’s look at the syntax for using an enum with a match expression:
enum EnumName {
Variant1,
Variant2,
Variant3,
Variant4,
}
match enum_variant {
EnumName::Variant1 => {}
EnumName::Variant2 => {}
EnumName::Variant3 => {}
}
We can see that the variants of the enum EnumName are used in the match expression by directly associating each variant with the enum using two colons:
EnumName::Variant => {}
The code inside the curly braces ‘{}’ will execute if the enum_variant matches the variant specified by EnumName::Variant.
Let’s look at a practical example. In the code below, we create an enum called PlayerMove, which has two variants: Left and Right. Then we use a match expression inside the print_move function to evaluate if move1 and move2 are Left or Right moves:
enum PlayerMove{
Left, Right,
}
fn print_move(direction:PlayerMove) {
match direction {
PlayerMove::Left => {
println!("Moving left");
},
PlayerMove::Right => {
println!("Moving right");
}
}
}
fn main() {
let move1 = PlayerMove::Right;
let move2 = PlayerMove::Left;
print_move(move1);
print_move(move2);
}
Standard Output:
Moving right
Moving left