Rust Structs and Functions
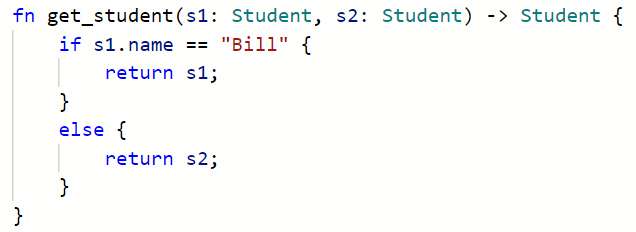
In Rust, structs and functions are both very popular and it is common to combine them in various ways.
The two most common (and basic) are:
- Passing structs into a function as an argument.
- Returning a struct from a function.
In this tutorial, we will cover both of these cases and learn how functions can be helpful when working with structs.
Passing a Struct to a Function
We can pass a struct into a function using the name of the struct.
In the function definition, we use the name of the struct to reference the data type of the parameter:
fn my_function(s: StructName) {}
In this example, s is the parameter and it has a type of StructName.
When we call the function, we provide the struct instance as the argument, which gets passed into the function.
my_function(struct_instance);
In the following example, we use the show_student() function to print out a student’s details in a useful way.
// Declaring a struct called Student:
struct Student {
name: String,
age: i32,
average: i32,
}
// Creating an instance of Student called 'bill':
let bill = Student {
age: 61,
name: "Bill".to_string(),
average: 83,
};
// Creating a function that shows the student details:
fn show_student(s: Student) {
println!("Name: {} , Age: {} , Average: {} ", s.name, s.age, s.average);
}
// Calling the show_student() function:
show_student(bill);
Standard Output:
Name: Bill , Age: 61 , Average: 83
Returning a Struct From a Function
We can return a struct from a function by specifying the struct name as the type to be returned, in the function definition.
fn my_function() -> StructName {}
The output of this function is a struct. If we want to do something with the struct then we will need to call the function from another function or macro.
We can also save the output as a new variable, and then work with that variable later on. In the following example, we are saving the output of the get_student() function into the variable ‘which_student’.
struct Student {
name: String,
age: i32,
average: i32,
}
let bill = Student {
name: "Bill".to_string(),
age: 61,
average: 83,
};
let steve = Student {
name: "Steve".to_string(),
age: 26,
average: 79,
};
fn get_student(s1: Student, s2: Student) -> Student {
if s1.name == "Bill" {
return s1;
}
else {
return s2;
}
}
let which_student = get_student(bill, steve);
println!("{} , {} , {}", which_student.name, which_student.age, which_student.average);