Rust Functions
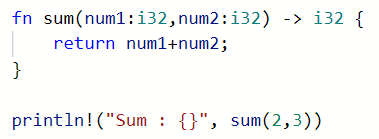
In the Rust programming language, a function is a reusable block of code. Functions are important because they improve code efficiency and organization. They help make applications faster and safer while keeping the code clean and organized.
Functions are defined using the fn keyword:
fn my_function() {}
The name of this function is ‘my_function’. The main body of the function is encapsulated by curly braces {}.
Whenever we want to use my_function(), we simply call it by its name:
my_function();
The code inside my_function will be executed whenever the function is called in this way, and it can be called as many times as we want.
This article will cover functions in-depth, including: defining and calling functions, working with parameters and arguments, and returning values from functions.
The main() Function vs. User Defined Functions
The main() function is the most important function in Rust, and is the start of any program. All of the program code lives inside the main() function, and it has some unique properties.
Other than the main() function, every other function in Rust is a user defined function. This article will focus on the general case of user defined functions rather than the main function.
Using Functions in Rust
Using a function requires two steps: (1) the function must be defined; and (2) the function has to be called. We will cover each of these in turn.
Defining a Function
A function is defined using the fn keyword followed by the name.
fn my_function() {}
Consider a practical example where we use a function to print the string “You Win!” to the console:
fn win() {
println!("You Win!");
}
win();
Standard Output:
You Win!
Note that in order for the win() function to execute, it needed to be called from inside the main() function. Function calling is covered in more detail below.
Function Naming in Rust
Functions use snake_case by convention, just like variables. This means that all letters are undercase and words should be separated using an underscore.
Using Parameters
Functions can take data as an input using parameters. Parameters gives functions much more usefulness because they allow functions to directly interact with data that is passed into them.
Parameters require two things: a name, and a data type. They are passed to the function inside parentheses ‘()’:
fn my_function(param1, param2) {}
For this to compile correctly, we have to specify the data type of each parameter:
fn my_function(param1: type, param2: type) {}
Let’s look at an example of a function called sum that takes two numbers and prints the sum to the console:
fn sum(num1: i32, num2: i32) {
println!("Sum : {}", num1 + num2);
}
sum(2,3);
Standard Output:
Sum : 5
Returning a Value from a Function
Functions can return a value using the return keyword. The type of the value being returned must also be specified in the function definition using an arrow ‘->’ followed by the data type.
fn sum(num1: i32, num2: i32) -> i32 {
return num1 + num2;
}
println!("Sum : {}", sum(2,3))
You can also return a value without using the return keyword.
Calling a Function
Functions have to be called in order to be executed. The Rust compiler won’t actually allow us to have functions that never get called, which helps to keep code safe and efficient.
Functions are called using their name and any arguments that are passed in to the function’s parameters:
my_function(arg1, arg2);
If my_function returns a value, then that value can be assigned to a variable on the same line:
my_var = my_function(arg1, arg2);
Or it can be operated on, passed into other functions, or otherwise worked with. The point here is that when a function is called, a return value can be used like any other value.