Rust Loop Labels
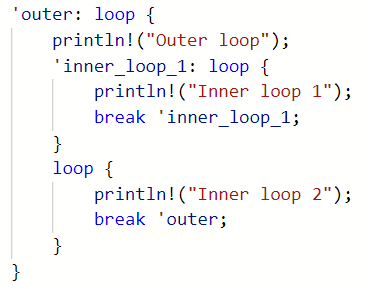
In Rust, loop labels are used to identify nested loops. This allows us to differentiate loops when we are working with nested code.
Specifically, loop labels allow us to use break and continue statements on an outer loop from inside of a nested loop.
For example, we can break out of an outer loop from inside an inner loop by using the break keyword with the loop label.
Loop label syntax uses an apostrophe/single quote (‘) character followed by the label name and a colon (:).
‘<loop label name>: <loop declaration>
For example, we can name an outer loop ‘outer and an inner loop ‘inner:
'outer: loop {
// Body of outer loop
'inner: loop {
// Body of inner loop
}
}
The individual outer and inner loops can now be referenced for added functionality using their loop labels: ‘outer for the outer loop and ‘inner for the inner loop.
This allows us to specify the desired functionality from inside the inner loop. In this example, there are four options available from inside the inner loop:
continue 'outer
continue 'inner
break 'outer
break 'inner
Let’s look at an application of using loop labels:
'outer: loop {
println!("Outer loop");
'inner_loop_1: loop {
println!("Inner loop 1");
break 'inner_loop_1;
}
loop {
println!("Inner loop 2");
break 'outer;
}
}
Standard Output:
Outer loop
Inner loop 1
Inner loop 2
Without loop labels, we would be limited to interacting with only the innermost loop (from inside that loop). We wouldn’t be able to break out of an outer loop from inside an inner loop.
Compiler Issues with Loop Labels
Loop labels need to be used for the compiler to accept them.
If a loop label is introduced and then isn’t used, the compiler will throw an error letting us know that the loop doesn’t need to be labelled.
This strictness forces the codebase to be more efficient because there is no need to store the loop label in memory if it isn’t needed for the program to function.