Rust Integers
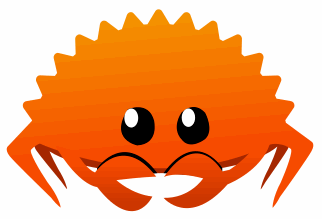
In Rust, an integer is a whole number, such as 1, 2, or 3. An integer does not have a decimal point, even if the value after the decimal point is zero (e.g. 1.0).
Integers are a primitive data type in Rust. The i32
type is default, which is a 32-bit signed integer.
Integers are one of two numeric data types in Rust, with the other being floating point numbers.
Rust provides both signed and unsigned integer types of varying sizes. Signed integer types can be either positive or negative in value, while unsigned types can only be positive.
Here’s an example of an integer in Rust:
let i = 42;
In this example, i
is an i32
(32-bit signed integer) with the value 42
.
We can perform various operations on integers, such as arithmetic operations (+
, -
, *
, /
, etc.), bitwise operations (&
, |
, ^
, etc.), and comparison operations (==
, !=
, >
, <=
, etc.).
Signed vs. Unsigned Integers
Size | Signed | Unsigned |
16 bits | i16 | u16 |
32 bits | i32 | u32 |
64 bits | i64 | u64 |
128 bits | i128 | u128 |
arch (32 or 64 bits) | isize | usize |
In Rust, a signed integer is a data type that can represent both positive and negative whole numbers, whereas an unsigned integer can only represent positive whole numbers. This means that a signed integer has a range of positive and negative values that is symmetrical around 0, while an unsigned integer only has positive values.
For example, the i32
type in Rust is a signed 32-bit integer, which means it can represent values from -2^31 to 2^31 – 1, inclusive. In contrast, the u32
type is an unsigned 32-bit integer, which means it can represent values from 0 to 2^32 – 1, inclusive.
In general, whether to use a signed or unsigned integer will depend on the specific requirements of your program. If you need to represent negative numbers, you will need to use a signed integer. Otherwise, you can use either a signed or unsigned integer, depending on the range of values you need to represent and other factors.
Rust Integer Math
To do math with integers in Rust, we can use the standard arithmetic operators such as +
, -
, *
, and /
. These operators can be used to perform addition, subtraction, multiplication, and division, respectively.
Here is an example of how we might use these operators in a Rust program:
let x = 5;
let y = 2;
let sum = x + y; // 7
let difference = x - y; // 3
let product = x * y; // 10
let quotient = x / y; // 2
In this example, the values of x
and y
are first defined as 5
and 2
, respectively. Then, the +
, -
, *
, and /
operators are used to perform the corresponding arithmetic operations on these values. Finally, the results of these operations are stored in the sum
, difference
, product
, and quotient
variables, respectively.
In addition to the standard arithmetic operators, Rust also provides a number of other methods and functions that we can use to perform more complex mathematical operations on integers. For example, we can use the pow
method to calculate the power of a number, the abs
method to calculate the absolute value of a number, and the gcd
function to calculate the greatest common divisor of two numbers.
Integer Addition
To add two integers in Rust, we can use the +
operator. This operator takes two operands, which can be any two valid integers, and returns the sum of the two operands.
Here is an example of how we might use the +
operator to add two integers in a Rust program:
Copy codelet x = 5;
let y = 2;
let sum = x + y; // 7
In this example, the values of x
and y
are first defined as 5
and 2
, respectively. Then, the +
operator is used to add these values together and store the result in the sum
variable.
In general, the +
operator can be used to add any two valid integers in Rust. It is a simple and straightforward way to perform this common mathematical operation.
Integer Subtraction
In Rust, we can use the -
operator to perform integer subtraction. Here’s an example:
let x = 5;
let y = 3;
let z = x - y;
println!("The difference between {} and {} is {}", x, y, z);
This code will print out the following:
The difference between 5 and 3 is 2
Keep in mind that the -
operator can only be used on two integers. If we try to subtract a non-integer value from an integer, we’ll get a compile-time error.
Additionally, if the result of a subtraction operation is negative, the result will be a negative integer. For example:
let x = 3;
let y = 5;
let z = x - y;
println!("The difference between {} and {} is {}", x, y, z);
This code will print out the following:
The difference between 3 and 5 is -2
Integer Multiplication
In the Rust programming language, we can use the *
operator to perform integer multiplication. Here is an example:
let x = 5;
let y = 4;
let result = x * y;
println!("The result of the multiplication is: {}", result);
This code will print “The result of the multiplication is: 20” to the console.
Note that we can also use the .mul()
method on integers to perform multiplication, like this:
let x = 5;
let y = 4;
let result = x.mul(y);
println!("The result of the multiplication is: {}", result);
This code will have the same result as the previous example.
Integer Division
In the Rust programming language, the /
operator performs integer division when used with integer operands. This means that if we divide two integers using this operator, the result will be an integer that is the quotient of the division operation. For example:
let a = 5;
let b = 2;
let c = a / b; // c will be 2, because 5 divided by 2 is 2 with a remainder of 1
If we want to perform floating-point division instead, we can use the /
operator with floating-point operands, or we can use the f32
or f64
type to explicitly specify that the operands are floating-point numbers. For example:
let a = 5.0; // a is a floating-point number with the value 5.0
let b = 2.0; // b is a floating-point number with the value 2.0
let c = a / b; // c will be 2.5, because 5.0 divided by 2.0 is 2.5
let a: f32 = 5.0; // a is a 32-bit floating-point number with the value 5.0
let b: f32 = 2.0; // b is a 32-bit floating-point number with the value 2.0
let c = a / b; // c will be 2.5, because 5.0 divided by 2.0 is 2.5
Note that the f32
and f64
types specify the size (in bits) and precision of the floating-point numbers, so you should choose the appropriate type based on your specific needs.
Rust Integer Overflow
In the Rust programming language, an integer overflow occurs when a numerical operation produces a result that is outside the range of values that can be represented by the integer type used in the operation. For example, if a program attempts to add two u8
values (which can represent unsigned 8-bit integers in the range 0 to 255) and the result of the operation is greater than 255, an integer overflow will occur.
To prevent integer overflow in Rust, the language provides a number of built-in safeguards. For example, the Rust standard library includes a Wrapping
type that can be used to wrap around the maximum value of an integer type, allowing numerical operations to continue even when an overflow would normally occur. Additionally, the Rust compiler can be configured to emit warnings or errors when it detects potentially overflowing operations in the code.
It’s important to note that integer overflow can be a serious security concern in some cases, as it can lead to unpredictable behavior in a program and potentially even allow attackers to exploit vulnerabilities in the program. For this reason, it’s important to use the available tools and techniques to prevent integer overflows in Rust.
Wrapping an Integer Type
As we saw in the last section, “wrapping” an integer type refers to using the Wrapping
type provided by the Rust standard library to wrap around the maximum value of the integer type. This allows numerical operations on the wrapped value to continue even when an overflow would normally occur.
To wrap an integer type, we first need to import the Wrapping
type from the std::num::wrapping
module. Then, we can create a Wrapping
value by passing the integer value that we want to wrap to the Wrapping
constructor. Here’s an example:
use std::num::wrapping::Wrapping;
let x = Wrapping(100u8);
Once we have a Wrapping
value, we can perform numerical operations on it just like we would with a regular integer value. When an overflow occurs, the Wrapping
value will automatically wrap around to the minimum value for the integer type and continue the operation. Here’s an example:
use std::num::wrapping::Wrapping;
let x = Wrapping(100u8);
let y = Wrapping(200u8);
// This would normally cause an overflow, but the Wrapping type
// will automatically wrap around to the minimum value and continue
// the operation.
let z = x + y; // Wrapping(44)
In the example above, the x + y
operation mathematically equals 300. This would normally cause an overflow for an u8
value (which has a maximum value of 255), but the Wrapping
type allows the operation to continue and instead produces a result of Wrapping(44)
. Note that this is equal to 300 – 255 -1 = 44. The extra -1 is because the count starts again at 0 (i.e. the first integer is 0 not 1).
It’s important to note that using the Wrapping
type can make it easier to avoid integer overflow, but it doesn’t completely eliminate the possibility of overflow. It’s still up to the programmer to use the Wrapping
type correctly and ensure that their code doesn’t produce unexpected results due to integer overflow.
Rust Integer to String
We can convert an integer value to a string using the to_string()
method provided by the std::string::ToString
trait. This method can be called on any type that implements the ToString
trait, which includes most built-in types such as i32
, u64
, and f32
.
Here’s an example of how to convert an i32
value to a string:
let x = 42;
let s = x.to_string();
In this example, the to_string()
method is called on the x
value, which has the i32
type. The method returns a String
value containing the string representation of the x
value.
It’s important to note that the to_string()
method may not always produce the same output for a given input. For example, the method may include leading or trailing zeros in the string representation of a numeric value, or it may use a different notation for floating-point values. The specific output of the to_string()
method can vary depending on the type and value of the input.
Additionally, the to_string()
method may not always be the best option for converting an integer to a string. For example, if you only need to convert a small number of values and don’t need to store the resulting strings, it may be more efficient to use the format!
macro provided by the Rust standard library. This macro allows us to embed the value of an integer (or any other type) directly into a string by using a special syntax. Here’s an example:
let x = 42;
let s = format!("The value of x is {}", x);
In this example, the format!
macro is used to create a string that includes the value of the x
variable. The value of x
is embedded in the string using the {}
placeholder, which is replaced with the string representation of the x
value when the macro is expanded. This can be a more efficient and convenient way to convert an integer to a string in some cases.
Rust Integer to Float
We can convert an integer value to a floating-point value using the as
keyword and the appropriate floating-point type. This keyword can be used to cast an integer value to any type that implements the std::convert::From
trait, which includes all of the built-in floating-point types such as f32
and f64
Here’s an example of how to convert an i32
value to an f64
value:
let x = 42;
let y = x as f64;
In this example, the x as f64
expression uses the as
keyword to cast the x
value from the i32
type to the f64
type. This produces a new f64
value that contains the floating-point representation of the x
value.
It’s important to note that this conversion may not always produce the same result for a given input. For example, if the integer value is too large to be represented accurately by the floating-point type, the resulting value may be rounded or truncated. Additionally, some integer values may not have a exact representation in floating-point format, in which case the conversion may introduce a small amount of error.
If you need to convert an integer to a floating-point value and you want to avoid potential loss of precision or accuracy, you can use the to_f64()
or to_f32()
method provided by the std::convert::TryInto
trait. These methods allow us to convert an integer value to a floating-point value and handle any errors that may occur during the conversion process. Here’s an example:
use std::convert::TryInto;
let x = 42;
let y = x.try_into().unwrap(); // f64
In this example, the try_into()
method is called on the x
value, which has the i32
type. The method returns a Result
value that indicates whether the conversion was successful. In this case, the unwrap()
method is used to unwrap the Result
and return the converted value directly. If the conversion fails, the unwrap()
method will panic.
Overall, converting an integer to a floating-point value in Rust is a relatively simple process, but it’s important to be aware of the potential limitations and risks of such a conversion. Use the appropriate method and type for your specific needs to ensure that the conversion is performed accurately and safely.
Integers in The Rust Programming Language – Conclusion
In this article, we have explored many different ways of working with integers in the Rust programming language. We have seen different types of integers including signed and unsigned integers of varying sizes, and learned how perform mathematical operations with them.
We learned about integer overflow and how to prevent it. Finally, we saw a few techniques for converting integers into other data types in Rust.
Hopefully this article has been helpful to you in your journey as a Rustacean!