Rust Vector Methods
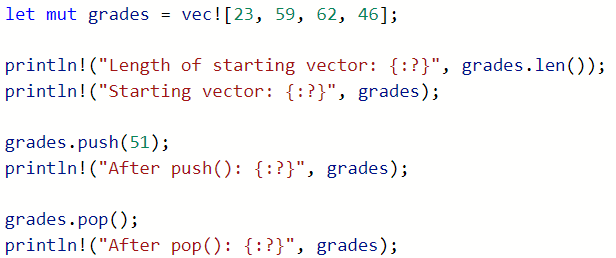
Rust has a number of methods that allow us to easily perform complex tasks with vectors. A method is a type of function that acts on the vector, providing integrated functionality that make vectors extremely powerful.
These methods include push(), pop(), len(), contains(), and remove(). They allow us to add or remove elements, get the length of the vector, or to check if a vector contains an element with a specific value.
Each method has a unique functionality and some have a special syntax as well. Most methods change the vector, so the vector must often be mutable. This can be done using the keyword mut in the vector definition.
In this article, we will cover essential vector methods in the Rust programming language.
Vector Methods
The following table compares several important vector methods:
Table of Vector Methods in Rust
Method | Description |
.push() | Pushes a value onto the end of the vector |
.pop() | Pops the last element from the vector |
.len() | Returns the length of the vector |
.remove(i) | Removes the element at index i |
.contains(<value>) | Returns true if the vector contains <value> |
Vec::new() | Creates a new vector |
Using Vector Methods in Rust
Vector methods use a syntax that specifies the method using a period ‘.’ after the name of vector:
my_vector.method()
For example, we can push an element to the end of a vector as follows:
my_vector.push(42);
This will add an element to the end of the my_vector. The value of the element will be the integer ’42’.
The following example shows the usage of each vector method:
let mut grades = vec![23, 59, 62, 46];
println!("Length of starting vector: {:?}", grades.len());
println!("Starting vector: {:?}", grades);
grades.push(51);
println!("After push(): {:?}", grades);
grades.pop();
println!("After pop(): {:?}", grades);
grades.remove(0);
println!("After removing 0th element: {:?}", grades);
println!("Contains 88? {:?}", grades.contains(&88));
Standard Output:
Length of starting vector: 4
Starting vector: [23, 59, 62, 46]
After push(): [23, 59, 62, 46, 51]
After pop(): [23, 59, 62, 46]
After removing 0th element: [59, 62, 46]
Contains 88? false